Software Dependency Management: A Complete Guide
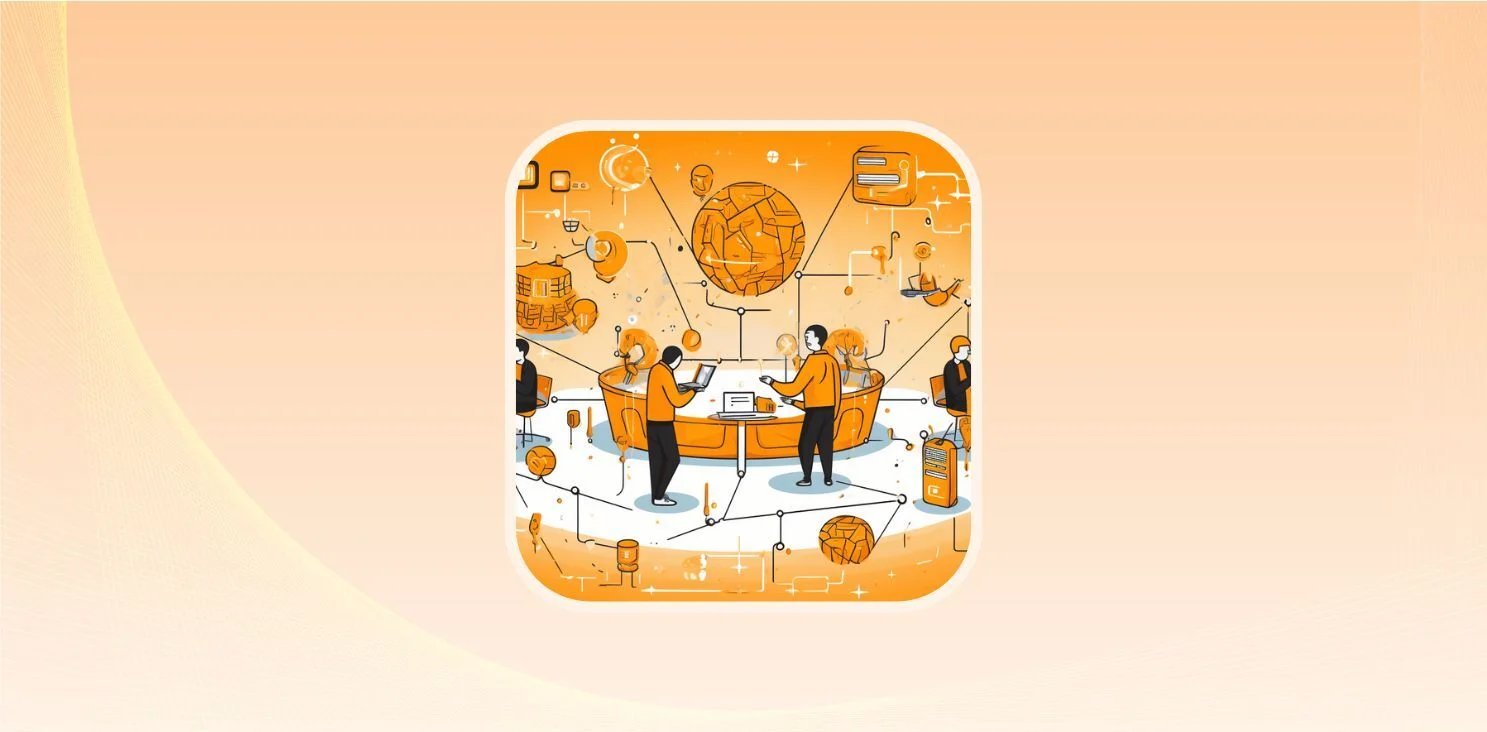
Let’s say you have to build a closet. But the parts are all wrong–some of the screws are outdated, the panels do not fit as they should, and you do not have the right tools for the job. It would be almost impossible to build that wardrobe, and even if you did, it wouldn’t be very stable.
This is exactly what happens in software development with dependencies. Managing dependencies keeps your software stable and reliable by making sure they’re up-to-date, compatible, and secure.
But if you mismanage dependencies, you may end up with version conflicts, outdated components, or even security risks which may result in build failures or even compromised software.
What is Software Dependency Management and Why Does It Matter?
Software dependencies are the components your project leans on—libraries, frameworks, tools. They handle all the heavy lifting so developers aren’t stuck building everything from scratch. But the more you rely on these, the more complex things can get.
So, what is dependency management? It’s your way of staying on top of these components—tracking, updating, and making sure they don’t clash with each other. Why does this matter? Because poorly managed dependencies can cause a whole host of problems.
Here are a few reasons why getting this right is so critical:
- Security Risks Are Everywhere: With old or unpatched dependencies, attackers know exactly where to look. These vulnerabilities can quickly become open doors to exploits if they’re not addressed.
- Version Conflicts Are a Pain: Imagine one part of your app needs version 1.5 of a library, but another demands version 2.0. If these don’t play nice together, you’re left scrambling to fix issues that can grind your project to a halt. Developers call this “dependency hell” for a reason.
- Stability Isn’t a Given: Misaligned dependencies can break your builds or cause your app to behave unpredictably. Bugs pop up in places you least expect, and debugging becomes an uphill battle.
- Licenses Aren’t Just Legalese: Every dependency comes with its own licensing terms. Some of these might restrict how you can use or distribute your software. You could be looking at legal issues if you don’t adhere to those terms.
Understanding the Different Types of Software Dependencies
When you’re working with software dependencies, it’s helpful to categorize them. Not all dependencies behave the same way, and their impact on your project can vary widely. Let’s break this down into a few simple groups to make things clearer.
Direct vs. Transitive Dependencies
- Direct Dependencies: These are the components you deliberately add to your project and use directly. If your code explicitly calls functions from a library or tool, that’s a direct dependency. For instance, if you’re using a library to process dates, and you write code that directly references it, that’s a clear example of a direct dependency.
- Transitive Dependencies: Here’s where it gets more layered. Transitive dependencies are the ones your direct dependencies rely on to function. You don’t interact with these directly, but they’re still critical. Think of it like this: if Library A relies on Library B to work, and you’re using Library A in your project, you’ve now inherited Library B as a transitive dependency. The catch? If Library B updates or changes, it could affect your project in unexpected ways—even though you didn’t actively choose it.
Development vs. Runtime Dependencies
- Development Dependencies: These are the tools you use to build or maintain your project, but they don’t actually ship with the final software. For example, testing frameworks, compilers, or linters are common development dependencies. They help ensure your code is clean, efficient, and error-free during development but aren’t part of the application your users interact with.
- Runtime Dependencies: Runtime dependencies, on the other hand, are non-negotiable for your software to function in a live environment. These are the frameworks or libraries your application relies on when it’s running. If you’ve built a web application, the core framework it uses—whether that’s Ruby on Rails, Spring, or something else—is a runtime dependency.
Balancing these two types is a bit of an art. Too many development dependencies can slow things down, while an overload of runtime dependencies can make your application heavier than it needs to be.
Optional Dependencies
Optional dependencies sit in a category of their own. These aren’t critical for your software to run, but they can add a lot of value. Maybe it’s a plugin that provides analytics or an add-on that introduces some extra customization options for users.
The key with optional dependencies is flexibility. Your software can still operate without them, but if the right components are present, it can take advantage of these extras. That said, it’s easy to go overboard. Adding too many optional dependencies can complicate your setup and increase maintenance overhead.
Common Pitfalls of Inadequate Dependency Management
Dependency management might feel like a background task until it isn’t. When it’s handled poorly—or not at all—the problems stack up quickly.
Security Risks: The Invisible Threat
Picture this: your application runs smoothly for months until, one day, a hacker finds an old, unpatched library in your codebase. It’s a weak point you didn’t even realize was there, but it’s well-documented—and easy to exploit. Outdated dependencies are like that. They lurk in the background, quietly putting your software at risk.
The problem doesn’t stop with old libraries. Adding a new dependency without checking its security credentials is equally dangerous. Maybe it’s a library you found in a pinch, one that solves a specific problem, but you didn’t dig deep into its reputation or maintenance history. A dependency with a shaky foundation can introduce vulnerabilities that aren’t immediately obvious.
The Struggles of Version Conflicts
If you’ve ever had two libraries demand incompatible versions of the same dependency, you know the frustration of “dependency hell.” One part of your application insists on version 1.4, while another needs version 2.0. Resolving this isn’t always straightforward, and it’s a time sink that can derail your development schedule.
Even when version conflicts aren’t at play, dependency mismanagement can lead to instability. If one environment uses a slightly different library version than another, you might find your app working fine in staging but crashing in production. Debugging these inconsistencies is frustrating, especially when you’re up against tight deadlines.
The Weight of Technical Debt
Every dependency you add to your project brings complexity with it, leading to the accumulation of technical debt. Over time, these layers pile up, making the codebase harder to update or refactor. It’s not always noticeable at first, but as your project grows, the cracks start to show.
Legacy dependencies are another headache. These outdated libraries might still work, but they often don’t play well with modern tools. They can slow development to a crawl, introduce bugs, and cause compatibility issues that take far longer to resolve than anyone expects.
Legal Issues You Didn’t See Coming
Here’s a scenario: you’re working on a commercial project, and one of your dependencies turns out to have licensing restrictions you didn’t check. Maybe it prohibits redistribution in certain contexts, or it requires attribution in a way you didn’t realize. Now you’re facing potential legal trouble for something that could’ve been avoided with a quick review.
Licensing terms aren’t always clear, either. Some are ambiguous, leaving room for misinterpretation. If you’re not proactively managing this, you could run into problems when it’s time to release your software.
Performance Problems
Have you noticed how applications with too many dependencies tend to feel slow and bloated? Every library you add brings overhead. If those libraries aren’t optimized—or if you’re using more than you need—you’ll start to see the impact on performance.
Slower load times, higher memory usage, and inefficient algorithms hidden in poorly maintained dependencies all add up. Users notice this kind of thing quickly, and it can make or break their experience with your software.
Debugging Dependencies: A Tedious Game
When something goes wrong in a complex dependency chain, finding the root cause can feel like solving a riddle with half the clues missing. You’re not just debugging your code—you’re digging through layers of third-party libraries, some of which you might not have even realized were part of your project.
And then there’s the unpredictability. Issues that arise from mismanaged dependencies often show up in inconsistent ways. A bug might only occur under specific conditions, making it tough to replicate and even tougher to fix.
6 Software Dependency Management Best Practices
Dependency management isn’t something you notice when it works—it’s only when things break that you start paying attention. From surprise crashes to security breaches, failing to manage dependencies properly can derail even the best-planned projects.
Start with Automation
Picture this: you’re working on a project, and no one remembers who last updated a core library. Everyone’s guessing—and nothing works. This is where you can lean on tools like npm, Maven, or Bundler come in. They handle installations, updates, and even version tracking.
And don’t stop there. Add tools like Codacy into your CI/CD pipeline to watch over your dependencies and flag issues before they turn into disasters.
Updates Aren’t Optional
Here’s the harsh truth: skipping updates to “save time” will cost you more later. The longer you wait, the harder it becomes to patch outdated libraries.
Think of it this way: keeping dependencies updated isn’t just about fixing vulnerabilities. It’s about staying ahead—using better-optimized versions, avoiding deprecated features, and reducing technical debt that slows future progress.
Choose Stability Over Chaos
Imagine releasing your app, only to find out it crashes on staging because of a library update no one accounted for. Sound familiar? Using tools like lockfiles ensures everyone on your team is working with the same versions. It’s predictable. Stable. And let’s be honest—it saves a ton of time when debugging.
Be Selective: Do You Really Need That Library?
You can add libraries for the simplest things—like formatting strings or parsing dates. Sure, it works, but now your project’s bloated, harder to maintain, and way more vulnerable.
Ask yourself: “Do we actually need this or can we write our own function?”
Isolate Dependencies
Isolating dependencies in containers or virtual environments prevents conflicts, keeping your projects tidy and manageable.
And the best part? Consistency. Whether you’re working on your local system or deploying to production, everything behaves the same.
Write the Rules
Dependency management without documentation sets teams up for failure. Write down your policies. It doesn’t have to be perfect, but it has to exist. Documentation also provides clarity on an organizational level.
Keep Your Supply Chain in Check with Codacy
Managing dependencies isn’t optional anymore. It’s a necessity. But the truth is, keeping track of vulnerabilities, licenses, and outdated components can feel overwhelming—especially when the stakes are high. Codacy changes that.
Codacy’s Software Composition Analysis (SCA) automatically scans open-source components for issues that could put your project at risk. It’s not just a one-time check—it’s continuous monitoring.
What’s great is how Codacy fits right into your CI/CD pipeline. You don’t need to overhaul your workflow to stay secure—Codacy works alongside your processes so your code remains stable, up-to-date, and ready to go live.
Curious about how Codacy works? Book a demo or start a free trial.