Code Smells and Anti-Patterns: Signs You Need to Improve Code Quality
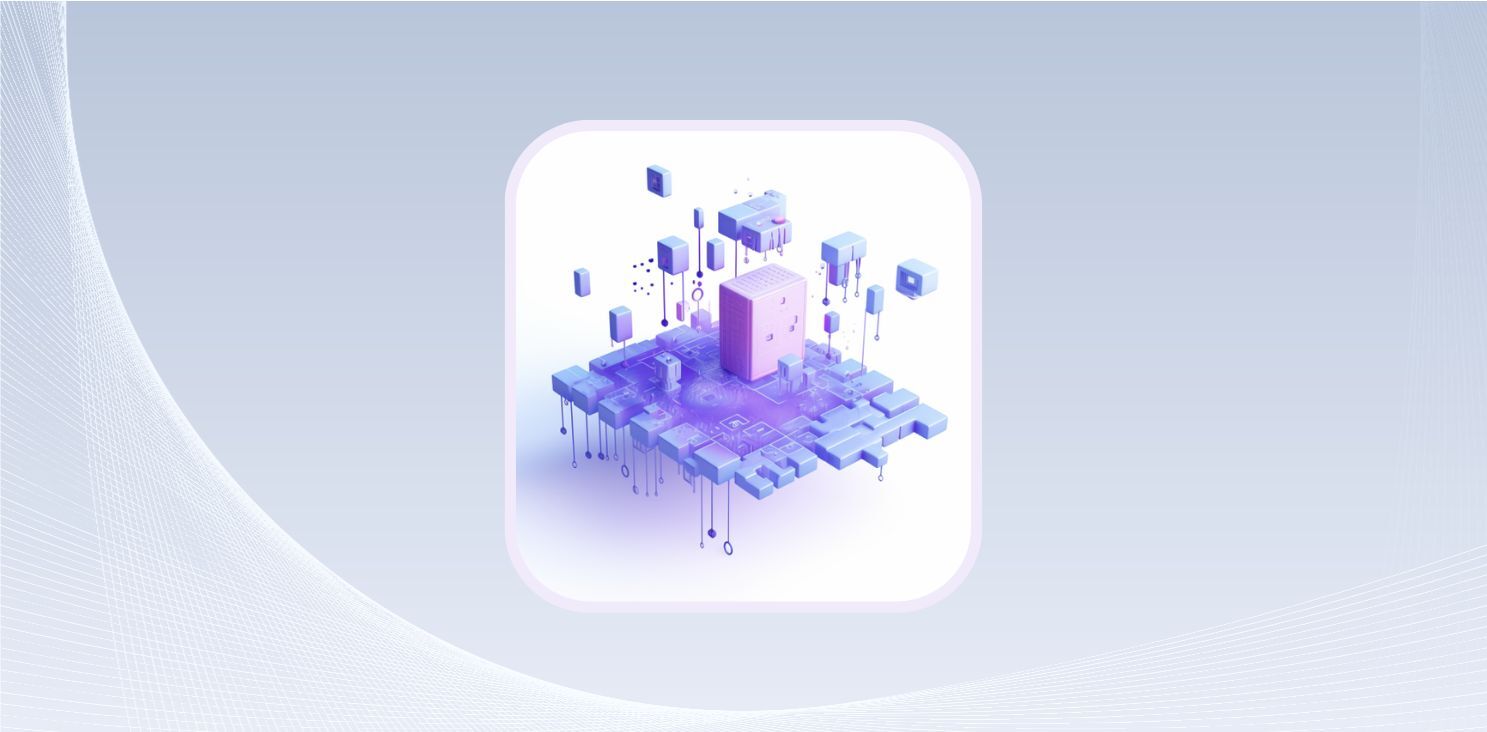
Software quality isn't a luxury–it's a necessity. As developers, we know that writing code isn't just about making things work; it's about crafting solutions that are efficient, maintainable, and clear.
That’s the theory. The practice devolves into something else. Amid tight deadlines and evolving requirements, it's common for codebases to develop specific 'symptoms' of potential problems lurking beneath the surface.
These symptoms, known as "code smells" and "anti-patterns," are tell-tale signs that our code might need attention. In this article, we'll delve into what these terms mean, why they matter, and how recognizing them can be the first step toward elevating the quality of our code.
What Are Code Smells?
Let's unpack the two essential terms: code smells and anti-patterns.
Simply put, a code smell is like that suspicious odor coming from your refrigerator—a hint that something might be off. In the context of coding, it means there's likely an underlying problem. It doesn't necessarily say that the code is wrong or broken but indicates areas where improvements might be needed. Think of code smells as yellow flags on the road, warning you to proceed cautiously.
While code smells hint at potential issues, anti-patterns are known practices that can lead to trouble. Imagine having a manual with all the wrong ways to assemble a piece of furniture—that's what an anti-pattern is in the coding world. It’s a common solution or approach that seems good but can introduce more problems than it solves. It's like using a hammer where a screwdriver is needed; sure, it might work, but it's not the best way to get the job done.
In essence, while code smells are symptoms of deeper problems, anti-patterns are proven ways that can lead us down the wrong path. Recognizing both is the first step to ensuring the health and longevity of our software projects.
Five Common Code Smells
Each code example demonstrates a specific code smell and provides a more optimal approach to handling the same problem. The key takeaway is that keeping code clean and maintainable often involves recognizing these patterns and knowing how to refactor them effectively.
By delving deeper into each code smell, developers can better understand their implications on code quality and potential pitfalls. Recognizing these smells early on and addressing them proactively ensures a cleaner, more efficient, and maintainable codebase.
Understanding the consequences of these code smells is critical. It not only emphasizes the importance of clean code practices but also illustrates the potential pitfalls and challenges that arise when such smells go unaddressed.
1. Duplicated Code
Duplicated code is when the same code appears in multiple places. This is one of the most straightforward code smells to spot. If the same or very similar code appears in numerous places, it clearly indicates duplication.
Here’s an example:
def calculate_area_rectangle(length, width):
return length * width
def calculate_area_square(side):
return side * side
Both functions do the same multiplication. The calculate_area_square function is unnecessary.
Duplicated code means that if there's a bug in that logic, it may exist in multiple places. It also means that any updates or changes must be done in all those places, which is error-prone and inefficient. Duplicated code also makes it difficult to determine which code is being executed, especially if slight variations exist between duplicates.
Consider creating a shared method or function that houses the repeated logic and call that method wherever necessary.
For a square, you should pass the same value for length and width.
def calculate_area_rectangle(length, width):
return length * width
2. Large Class/Method
Sometimes, classes or methods grow too large, often doing too many things and making it difficult to understand and maintain. Such classes or methods often violate the Single Responsibility Principle.
A sprawling class or method can take time to digest and understand, especially for newcomers to the code. Additionally, the more a single method or class does, the harder it is to test. It might require complex setups and may have many edge cases. Changing a large class or method can inadvertently affect unrelated functionality, leading to unexpected bugs.
In this example, the User class shouldn't be responsible for calculating discounts.
class User:
def __init__(self, name, age):
self.name = name
self.age = age
def get_details(self):
return f"Name: {self.name}, Age: {self.age}
def calculate_discount(self, product_price):
if self.age > 60:
return product_price * 0.1
return 0
Instead, you should create a separate `DiscountCalculator` class or function.
class DiscountCalculator:
@staticmethod
def calculate_for_user(user, product_price):
if user.age > 60:
return product_price * 0.1
return 0
3. Long Parameter List
A function or method that requires a large number of parameters. Not only does this make the method signature unwieldy, but when a method takes many parameters, it's harder to remember the order, purpose, and possible values of each, making mistakes more likely.
Methods with long parameter lists also tend to be more susceptible to changes in requirements, often necessitating refactors, and the more specific the criteria to call a method (i.e., numerous parameters), the less likely the method can be reused elsewhere without changes.
You have to remember the order of this list to call this function properly:
def create_user(name, age, address, phone, email, gender, occupation):
pass
Consider grouping related parameters into objects or use **kwargs to handle a variable number of arguments.
def create_user(name, age, **kwargs):
pass
4. Feature Envy
Feature envy is when a method seems more interested in another class than the one it's in. This happens when a method in one class spends more time interacting with data or methods from another class than its own. It suggests that the method may belong in the other class or that there's an inappropriate relationship between the classes. It can lead to tightly coupled code when one class relies heavily on another's data and methods. Changes in one often necessitate changes in the other, leading to a ripple effect.
Accessing another class's data directly can break the principle of encapsulation, exposing internal details that should be hidden. Tight inter-class dependencies can make it challenging to refactor or redesign system parts without widespread changes.
Here, the get_full_address method is in the User class but calling data from the Address class:
class Address:
def __init__(self, street, city):
self.street = street
self.city = city
class User:
def __init__(self, name, address):
self.name = name
self.address = address
def get_full_address(self):
return f"{self.address.street}, {self.address.city}"
Move the method to the class it's envious of.
class Address:
def __init__(self, street, city):
self.street = street
self.city = city
def get_full_address(self):
return f"{self.street}, {self.city}"
5. Data Clumps
This is when you often see the same group of variables passed around together in various parts of the codebase. These clumps indicate that the data is related and might benefit from its structure or class.
When related data isn't grouped, it's easier to accidentally update or move part of it without the others, leading to potential inconsistencies. Ignoring data clumps can mean overlooking valuable abstractions that could simplify the system's design. Handling data clumps also often involves repetitive logic for validation, transformation, or other operations.
Here, we have several address-related variables that are all commonly used together:
def user_info(name, street, city, state, postal_code):
pass
Grouping them can centralize and reduce redundancy. Group the related variables into a cohesive structure or class. This not only improves readability but also makes the code more maintainable.
class Address:
def __init__(self, street, city, state, postal_code):
self.street = street
self.city = city
self.state = state
self.postal_code = postal_code
def user_info(name, address):
pass
The Consequences of Code Smells
Each of the individual code smells above has specific consequences. But code smells in your codebase have overarching effects as well.
First, it makes code hard to understand and maintain. They increase cognitive load, as code smells, by their very nature, complicate code structures. They introduce patterns that aren't intuitive, making developers spend more mental energy decoding the logic. They also increase technical debt. Like accruing financial debt, allowing code smells to persist means that there will be a "payment" due in the future—in this case, the time and effort required for refactoring.
Because of the increased complexity and technical debt, code smells also mean new team members face a steeper learning curve when encountering a codebase entirely of smells. It takes longer for them to become productive contributors.
Second, code smells increase the likelihood of bugs. Code smells often indicate more profound problems. While the smell might not be a bug, it can be a symptom of underlying issues or flawed logic. Code that's full of smells tends to be less resilient to changes. Even minor adjustments can lead to unexpected behavior or breakages elsewhere. When code isn't clear—when its intent is obscured by convoluted logic or structures—it's easy for developers to make assumptions that lead to errors.
Finally, they slow down development speed. Code that's hard to understand naturally takes longer to debug. Developers must first unravel the tangled logic before they can address the issue at hand. Introducing new features or adjusting a codebase riddled with smells becomes a daunting task. It's like trying to add a room to a house with a shaky foundation.
In team settings, code smells can lead to miscommunications. Developers might have conflicting interpretations when code doesn't convey its purpose or function, leading to integration problems or duplicated efforts.
These consequences underscore the importance of recognizing and addressing code smells promptly. While it might seem reasonable to ignore them in the short term, the long-term costs, in terms of productivity, code quality, and team morale, can be significant.
Common Anti-Patterns
Each anti-pattern provides valuable lessons in what to avoid when striving for clean, maintainable code. By understanding and recognizing these patterns, developers can take proactive measures to prevent them and enhance the quality of their software projects.
1. Golden Hammer
A Golden hammer is when you assume that a particular tool or method, which might have worked well in one scenario, is the right solution for various unrelated tasks.
Here’s an example of using list comprehension for every iteration task, even when inappropriate:
data = [1, 2, 3, 4, 5]
doubled_data = [x*2 for x in data] # Appropriate use
print_first_element = [print(x) for x in data if x == data[0]] # Inappropriate use
While list comprehensions are powerful in Python, using them for side effects (like printing) is not their intended use. The second list comprehension is an example of misusing a tool because it worked well elsewhere.
2. Cargo Cult Programming
Developers might implement patterns or methods because they're popular or have been seen in other projects without understanding their purpose or appropriateness.
# Using decorators without understanding them
def my_decorator(func):
return func
@my_decorator
def my_function():
print("Function called!")
In this example, a decorator `my_decorator` is used that does absolutely nothing. The developer might have seen decorators used elsewhere and added one without understanding its purpose.
3. Analysis Paralysis
Overthinking or over-analyzing a problem to the point where no action is taken.
While this is more of a development process issue than a coding one, a hypothetical scenario might be a developer spending weeks deciding on the best way to structure a database without ever starting it.
In situations like these, while planning and design are essential, there's also value in iterative development. Sometimes, you must decide, see how it works in practice, and adjust as necessary.
4. God Object
An object that controls too many aspects of a system, leading to a lack of modularity and an over-complicated structure.
class SystemControl:
def __init__(self, data):
self.data = data
def save_data(self):
pass
def process_data(self):
pass
def display_data(self):
pass
def log_errors(self):
pass
# ... many more methods
The SystemControl class does too much: data storage, processing, display, error logging, etc. This makes it hard to modify one functionality without affecting others. A modular approach with separate classes or functions for different responsibilities would be more straightforward and maintainable.
5. Spaghetti Code
Code that lacks a clear structure is often characterized by multiple nested conditions, loops, and lack of modularity, making it hard to read and maintain.
def process(data):
if data:
for d in data:
if d > 10:
if d < 50:
# Do something
pass
else:
# Do something else
pass
# ... more nested conditions
# ... more loops and conditions
The nested conditions and loops within the `process` function make it hard to follow the logic and pinpoint specific functionality. It becomes challenging to modify or debug this type of code without introducing new issues.
The Consequences of Anti-Patterns
The consequences of anti-patterns are not just short-lived issues; they can have long-term impacts on the health and viability of software projects. Recognizing and addressing anti-patterns early on is crucial for maintaining code quality and ensuring the sustainable growth and adaptability of software systems.
A primary consequence of anti-patterns is the inefficient solutions they elicit. You get performance bottlenecks through using inappropriate designs or algorithms that lead to slow execution times, especially noticeable in systems that need to handle large data sets or operate in real time.
You also need more resources, as anti-patterns can lead to the unnecessary consumption of memory, CPU cycles, or network bandwidth. For instance, a "God Object" might hold more data in memory than required, leading to wasted RAM.
This leads to increased costs where inefficient solutions result in financial implications, especially in cloud-based architectures where you pay for resources consumed. Using an anti-pattern might mean using (and paying for) more server power than you need.
For front-line developers, anti-patterns lead to code that takes time to adapt or extend. There is a lack of modularity in anti-patterns like the "God Object," which centralizes functionality, making it hard to make isolated changes without affecting other parts of the system.
You get technical debt accumulation as code becomes more intertwined and less clear. Addressing one issue or adding a feature can necessitate extensive refactoring or even complete rewrites. Because you are dealing with tech debt all the time, the organization also sees decreased agility, making it difficult for companies to respond to new market needs or opportunities.
Instead of leveraging existing, tested solutions, developers spend time crafting custom solutions that, in the end, offer no real benefit over established methods. By not researching and using established patterns and practices, developers miss out on the collective wisdom of the developer community.
Custom solutions haven't been through the rigorous testing and scrutiny that well-established patterns or libraries have undergone. As such, they're more prone to contain overlooked bugs or vulnerabilities.
Fragrant Code and Positive Patterns
Codebases are going to have the above problems. It is a certainty. Developers have to push code and are always looking for ‘efficiencies.’ That these short-term efficiencies lead to long-term inefficiencies is one of development's ironic paradoxes, where the drive for immediacy can inadvertently pave the way for future complexities.
So, every development team has to find ways to fight code smells and anti-patterns. Using code quality tools allows you to proactively identify and address these issues, ensuring a cleaner, more maintainable codebase while fostering a culture of continuous improvement and best practices.
But the goal of code quality is beyond mere rectification. It's about fostering a culture of continuous learning, where developers not only dodge harmful practices but also embrace positive patterns and principles. When our code is high quality, imbued with clarity and purpose, it doesn't just perform tasks—it tells a story, one of thoughtful craftsmanship and dedication to quality.
If you're looking for a tool that automates the process of keeping your code clean and secure as you write it, start your free Codacy trial today.