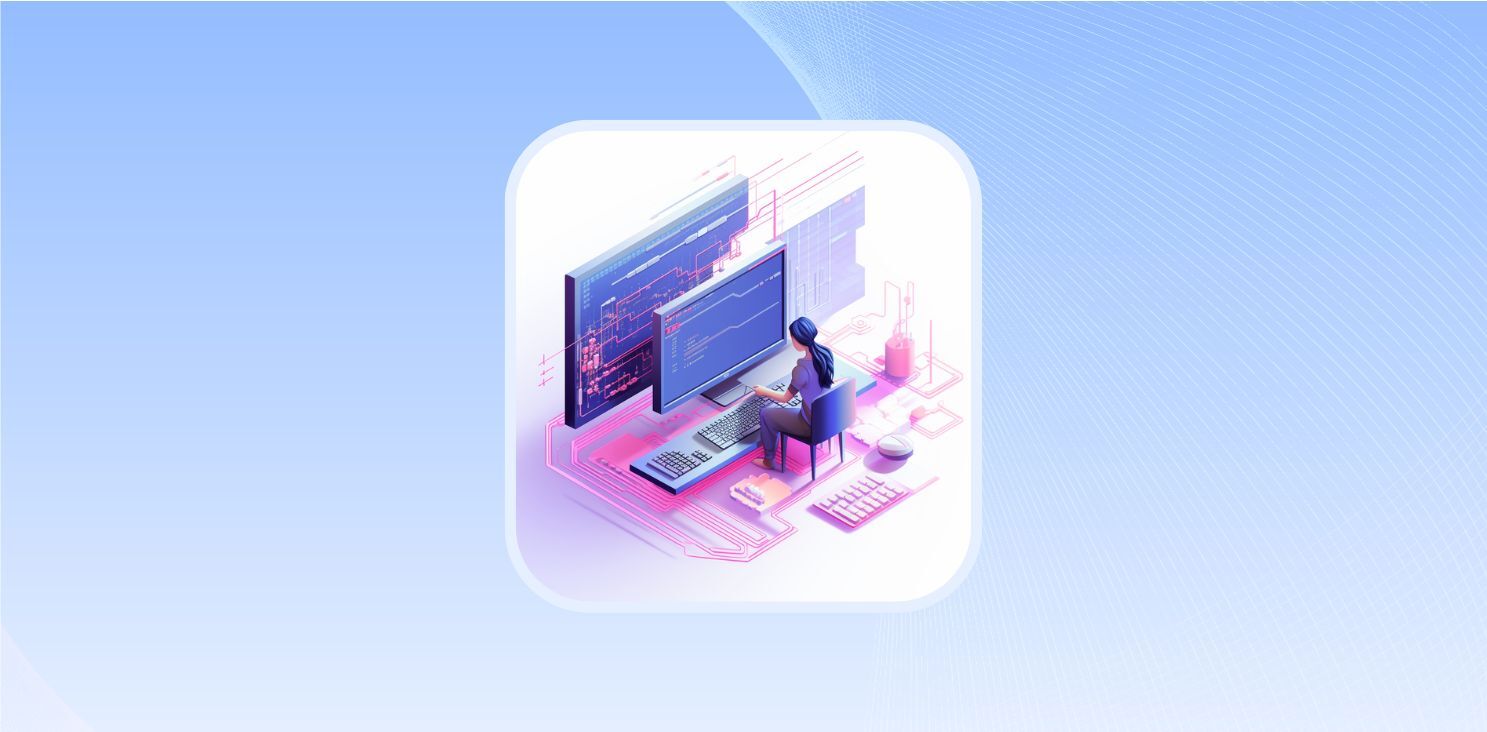
When you write code, you shouldn't just focus on making it work. You should also aim to make it readable, understandable, and maintainable for future readers and collaborators. This approach to software development is known as writing clean code.
Clean code principles are guidelines and best practices designed to enhance software systems' quality, maintainability, and scalability. By adhering to clean code principles, developers can create code that is not only functional but also readable, extensible, and easy to collaborate on.
This article overviews these clean code principles and offers a comprehensive, detailed guide to writing clean code.
Basic Principles of Clean Code
Clean code practices facilitate collaboration, reduce technical debt, and lead to more efficient and successful software development projects. Here are several key principles that characterize clean code:
1. Well-Structured Code
Clean code is well-structured and follows established design principles and patterns. It is split into logical functions, methods, classes, and modules, each with a single responsibility. This modular approach promotes code reusability, testability, and maintainability.
// Problematic:
void processPaymentAndGenerateInvoice() {
// Process payment
// Generate invoice
}
// Clean:
void processPayment() { ... }
void generateInvoice() { ... }
One of the most widely adopted principles for achieving well-structured code is the SOLID principle, which stands for:
- Single Responsibility Principle: A class or module should have only one responsibility.
- Open/Closed Principle: Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle: Child classes must be substitutable for their parent types.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules; both should depend on abstractions.
Following these principles helps create flexible, extensible code that's easier to maintain over time.
2. Properly Named Elements
Naming is one of the most critical aspects of writing clean code. It’s important that variable, function, and class names are clear, meaningful, and follow consistent naming conventions. Well-named entities make the code self-documenting and easier to understand.
// Problematic:
int a = 10; // What does 'a' represent?
void func1() { ... } // What does 'func1' do?
// Clean:
int accountBalance = 10; // Clearly indicates the purpose
void processPayment() { ... } // Describes the action
3. Proper Formatting and Indentation
Consistent formatting and indentation make the code structure more apparent, making it easier to navigate and understand.
Most programming languages and frameworks have established style guides or conventions for formatting and indentation. For example, in Python, the PEP 8 style guide provides recommendations for code formatting, such as using four spaces for indentation, limiting line lengths to 79 characters, and following specific rules for whitespace and naming conventions.
4. Easy to Read and Follow
Clean code should be easy to read and follow, with a clear and logical flow. This can be achieved by breaking down complex logic into smaller, more manageable functions and avoiding nested conditionals or loops that make the code harder to understand.
// Problematic:
if (condition1 && (condition2 || condition3) && condition4) {
// Complex logic here
}
// Clean:
if (isConditionMet()) {
// Simple and readable logic here
}
5. Proper Documentation
While clean code should be self-documenting, proper documentation is still essential for providing context, explaining complex algorithms or design decisions, and facilitating collaboration among team members.
Documentation should be concise, clear, and focused on explaining the "why" rather than the "what." See an example below:
def calculate_order_total(order):
"""
Calculate the total cost of an order, including applicable discounts.
Args:
order (Order): The order object containing order items and customer information.
Returns:
float: The total cost of the order after applying discounts.
"""
total_price = sum(item.price for item in order.items)
discount_rate = 0.1 if order.customer.is_premium else 0.0
return total_price * (1 - discount_rate)
Additionally, higher-level documentation, such as README files or design documents, can provide an overview of the project, its architecture, and any important decisions or considerations.
Best Practices for Writing Clean Code
Writing clean code is a skill that requires deliberate effort and guidance, and this section offers a step-by-step roadmap to help developers avoid common coding errors and write cleaner, more maintainable code:
1. Plan Before You Program
Before diving into coding, planning and designing your program is essential. The deeper you flesh out your program's architecture, requirements, and design, the cleaner your code will automatically become. Simple techniques like sketching diagrams, creating flowcharts, or writing pseudocode can help you in structuring your thoughts and make better design decisions upfront.
2. Embrace SOLID Principles
Adhering to the SOLID principles discussed earlier can create code that is modular, extensible, and easier to maintain.
3. Use Test-Driven Development (TDD)
Test-driven development (TDD) is a software development process in which you write tests before writing the actual code. This approach helps ensure your code is testable, maintainable, and meets the desired requirements. If you find writing tests tedious, complicated, or annoying, it may be a sign that your code is poorly designed and needs refactoring.
4. Understand Secure Coding Standards
Familiarize yourself with secure coding standards such as OWASP Top 10, CWE/SANS Top 25, and industry-specific guidelines. Adhering to these standards ensures that your code follows best practices in security, reducing the likelihood of introducing vulnerabilities and promoting cleaner code architecture.
5. Learn About Security Vulnerabilities
Educate yourself about common security vulnerabilities such as SQL injection, cross-site scripting (XSS), and authentication flaws. Understanding how these vulnerabilities occur and their impact on applications enables you to write code that mitigates these risks, leading to cleaner and more resilient software.
6. Leverage SAST and DAST Tools
Integrate Static Application Security Testing (SAST) and Dynamic Application Security Testing (DAST) tools into your development process. SAST tools analyze code for vulnerabilities during development, helping you identify and fix security issues early. DAST tools, on the other hand, simulate real-world attacks on running applications and provide insights into runtime vulnerabilities. By incorporating these tools, you enhance code security and promote cleaner code by proactively addressing potential security flaws.
7. Document Your Code Effectively
Document your code's purpose, preconditions, postconditions, and invariants through header comments for functions and classes. This helps clarify the code's intent and serves as a design review mechanism.
8. Be Intentional With Naming
Naming is a crucial aspect of writing clean code. Choose descriptive and meaningful names for variables, functions, and classes. Avoid ambiguous or misleading names, which can make your code harder to understand and maintain.
9. Practice DRY (Don't Repeat Yourself)
Eliminate code duplication by refactoring common code into reusable functions or modules. If you have two nearly identical functions, consider pulling the shared parts into a new helper function.
10. Use Small, Focused Functions
Strive to write small, focused functions that perform a single task. If a function becomes too long or complex, consider breaking it into smaller, more manageable functions.
11. Refactor Continuously
Refactoring your code continuously helps you to improve its design, remove technical debt, and keep it clean and maintainable. Look for opportunities to simplify complex logic, eliminate code duplication, and improve naming and organization.
12. Embrace Code Reviews
Participate in code reviews with your team members or peers. Code reviews can help identify potential issues, code smells, and areas for improvement. They also promote knowledge sharing and collaboration, improving code quality and consistency.
Write Clean Code With Codacy
Clean code is not just about making your code work; it's about creating code that is easy to understand, modify, and extend. By investing time and effort into writing clean code from the start, you can save countless hours of frustration and technical debt down the line.
Codacy can help you identify and fix code quality issues, security vulnerabilities, and code style violations. Integrating Codacy into your development workflow enables you to receive real-time notifications about the state of your codebase. Try it out today.