Best Practices for Identifying and Eliminating Code Smells
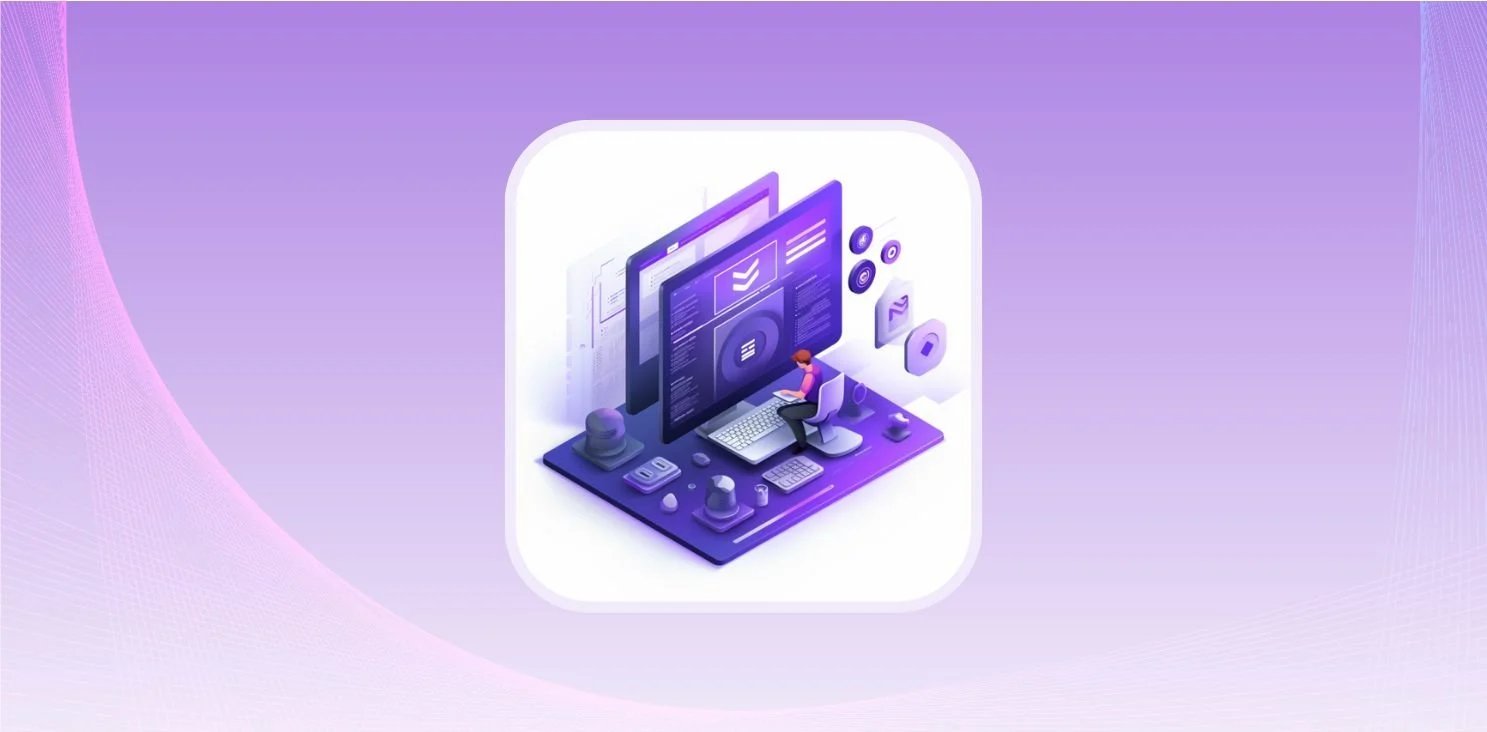
Keeping your codebase clean, efficient, and maintainable starts with awareness. Just as a vigilant car owner investigates strange sounds to catch potential issues before they become significant problems, developers must keep an eye out for "code smells."
Just as peculiar sounds in your car indicate it might need maintenance, code smells alert developers to parts of the code that could be problematic. They are subtle indicators that something could be amiss in your code, acting as warning signs and hinting at areas requiring attention.
Just as a well-maintained car runs smoothly and reliably, a codebase free of smells ensures a smoother development process, fewer bugs, and easier maintenance in the long run.
Understanding what code smells are is only half the battle. To ensure code quality, software development teams must be able to identify code smells effectively and eliminate them once they’ve been located.
Common Code Smells Causes
Code smells are manifestations of poor or misguided programming practices. They often stem from mistakes made by developers during the coding process. While not necessarily bugs, code smells can lead to performance problems and make applications difficult to maintain.
They serve as indicators for potential breaches of code discipline and design principles, often signaling a need for improvement. Some common causes of code smells include:
- Rapid development practices in response to tight deadlines. Pressure to deliver quickly may lead developers to prioritize speed over code quality, resulting in shortcuts or compromises. According to our State of Software Quality report, by far, the single most common challenge during code reviews for development teams is time constraints.
- Inexperienced developers being asked to work on unfamiliar projects or with programming languages they do not know well enough can inadvertently produce code that exhibits poor design choices or violates best practices.
- Overly complex code that’s difficult to understand and maintain.
- Inheriting a legacy codebase with outdated or poorly structured code that’s challenging to maintain.
- Insufficient unit tests or neglecting to test edge cases.
- Failure to adhere to established coding standards or conventions within your team.
By understanding the root causes of code smells, developers can proactively address them and foster a clean, high-quality code culture within their projects.
How to Identify Code Smells
Before you're able to find code smells, you have to know what you’re looking for. Some common indicators of code smells include:
- Long methods containing a large number of lines of code.
- Duplicated (identical or very similar) code segments appearing in multiple places within the codebase that violate the DRY (Don't Repeat Yourself) coding principle and can lead to maintenance challenges and inconsistencies.
- Large classes that contain many methods, attributes, or lines of code can indicate poor cohesion and violate the Single Responsibility Principle (SRP).
- Complex conditional logic, like methods or sections of code with nested if-else statements, switch statements, or multiple levels of indentation, which are hard to follow and test.
- Primitive obsession, which is the overuse of primitive data types (like strings or integers) instead of creating custom domain-specific objects. This often leads to code duplication, lack of type safety, and difficulty understanding the code's intent.
- Feature envy—methods that excessively access attributes or methods of other classes instead of relying on their data and behavior—can indicate a lack of encapsulation and cohesion.
- Large parameter lists that make method calls and invocations cumbersome and error-prone may indicate a violation of the Principle of Least Astonishment (POLA), which usually suggests that the method is doing too much.
Manual code reviews, conducted by peers or experienced developers, can examine codebases line by line, looking for these common code smell indicators. They also allow team members to share insights and best practices, fostering collaboration and collective learning.
Another method for identifying code smells is using automated static code analysis tools. Static code analysis is the process of automatically scanning your codebase for known coding errors and issues, many of which can directly confirm the existence of code smells. By leveraging static code analysis, developers can quickly identify potential issues and prioritize areas for improvement.
In addition to manual reviews and automated tools, developers can utilize complexity metrics to identify code smells. Metrics such as cyclomatic complexity, which measures the number of independent paths through a method, can help pinpoint areas of the code that may be overly complex and prone to issues. By monitoring code complexity metrics over time, developers can identify trends and be proactive in refactoring and simplifying complex code.
How to Eliminate Code Smells with Refactoring
Refactoring is the most effective technique development teams can use to maintain code hygiene and eliminate odorous code. In this process, developers restructure code, making it more concise, cleaner, and efficient while ensuring that the core functionality of the code remains intact.
According to best practices, the optimal time to refactor your code is before a planned release of new features or updates to old ones. If you plan on adding new code to your application, doing some cleanup work on your old code first makes sense to ensure it is added to healthy and functional existing code.
It’s also important to make time for refactoring. It’s often a complex and time-consuming process that shouldn’t be rushed.
Ensuring complete test coverage before refactoring code is also crucial because it helps mitigate the risk of introducing new bugs during the refactoring process. Comprehensive testing verifies that the existing functionality of the code behaves as expected before any changes are made. This allows developers to refactor confidently, knowing they have tests to catch any regressions or unintended consequences of their changes.
Refactoring by Abstraction
Refactoring by abstraction involves isolating complex or duplicated code into separate abstractions, such as classes, interfaces, or methods, to improve modularity and readability.
The first step in this technique is identifying complex or duplicated code segments—ones that often perform similar tasks or contain logic that can be abstracted into reusable components.
You can then create abstraction layers, such as classes, interfaces, or methods, that are introduced to encapsulate the identified code segments. Each abstraction layer is designed to represent a cohesive set of functionality or behavior, promoting modularity and encapsulation.
Once abstraction layers are created, developers refactor existing code to utilize these abstractions instead of duplicating logic. This helps to simplify code, reduce redundancy, and improve code maintainability and extensibility.
Consider the following code snippet, which contains duplicated logic for calculating the area of different shapes:
public class ShapeCalculator {
public double calculateRectangleArea(double length, double width) {
return length * width;
}
public double calculateCircleArea(double radius) {
return Math.PI * radius * radius;
}
// Other methods for calculating areas of different shapes
}
Using the refactoring by abstraction technique, we can refactor the code as follows:
public interface Shape {
double calculateArea();
}
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
public double calculateArea() {
return length * width;
}
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
public class ShapeCalculator {
// Other methods
// Refactored method to use abstraction
public double calculateShapeArea(Shape shape) {
return shape.calculateArea();
}
}
In this refactored version, duplicated logic for calculating areas of different shapes has been abstracted into separate Rectangle and Circle classes implementing the Shape interface. The ShapeCalculator class now utilizes these abstractions to calculate the area of any shape, promoting code reuse and improving maintainability.
Refactoring by Composing Methods
The composing methods technique targets eliminating redundant methods, aiming to streamline code structure and improve readability. One way to do this is by breaking down code into smaller blocks.
Fragmented sections of code are identified within larger methods or functions. These fragmented code blocks are isolated and extracted into separate, cohesive methods.
Another way is to identify and remove unnecessary methods. Developers identify methods that are broken or no longer necessary within the codebase. Calls to these redundant methods are replaced by the actual code within them, after which the original, now-unused methods can be safely deleted.
Consider the following code snippet, which contains a redundant method calculateTotal:
public class ShoppingCart {
private List<Item> items;
// Other methods and fields
// Redundant method
public double calculateTotal() {
double total = 0.0;
for (Item item : items) {
total += item.getPrice();
}
return total;
}
// Other methods
}
Using the composing methods technique, we can refactor the code as follows:
public class ShoppingCart {
private List<Item> items;
// Other methods and fields
// Refactored method
public double getTotal() {
double total = 0.0;
for (Item item : items) {
total += item.getPrice();
}
return total;
}
// Other methods
}
In this refactored version, the redundant calculateTotal method has been replaced with a more descriptive and streamlined getTotal method, simplifying the codebase and improving clarity.
Refactoring by Simplifying Method Calls
This technique involves streamlining and clarifying the process of invoking methods within a codebase. Some common approaches to simplify method calls include:
- Adding or removing method parameters to better align with their purpose or to eliminate unnecessary complexity.
- Renaming methods with clearer, more descriptive names.
- Splitting methods into separate queries (methods that retrieve information) and modifiers (methods that change the state of an object).
- Consolidating related parameters into a single object (parameter object) or parameterizing methods.
- Eliminating methods that solely assign values to object properties.
- Invoking explicit methods or calls instead of passing parameters to a method.
Here's an example demonstrating how we can simplify method calls using some of the mentioned techniques:
public class User {
private String firstName;
private String lastName;
private int age;
public User(String firstName, String lastName, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
// Refactored method to update user's age with explicit method call
public void setAge(int newAge) {
this.age = newAge;
}
// Refactored method call to remove method that assigns object values
// (Assuming the age is updated externally before this call)
public void updateUser(User userToUpdate) {
// Update user's age
userToUpdate.setAge(30); // Explicit method call
}
}
In this refactored version:
- We introduced a setAge method to explicitly update the user's age. This simplifies method calls by providing a clear and descriptive method name.
- We removed the updateAge method, as assigning the age directly within client code is more straightforward and eliminates the need for an intermediary method.
- We demonstrated a simplified method call in the updateUser method, where we directly invoke the setAge method with an explicit parameter value instead of relying on an intermediate method to perform the assignment.
These changes make the codebase more readable, maintainable, and concise.
Make Refactoring Code Smells Part of Your Development Culture
Software teams can collectively identify and address code smells more effectively by fostering a collaborative environment where refactoring is embraced as a recurring activity.
Encourage collaborative code reviews and pair programming sessions to facilitate knowledge sharing and ensure that refactoring efforts are comprehensive and well-informed.
Invest in developer education and training on refactoring techniques to empower team members to eliminate code smells and uphold coding standards proactively.
Don’t forget to add some automation to these more manual best practices. Employ static code analysis tools, like Codacy, to enforce coding standards and automate refactoring processes to further streamline the identification and elimination of code smells, ultimately leading to cleaner, more maintainable codebases.