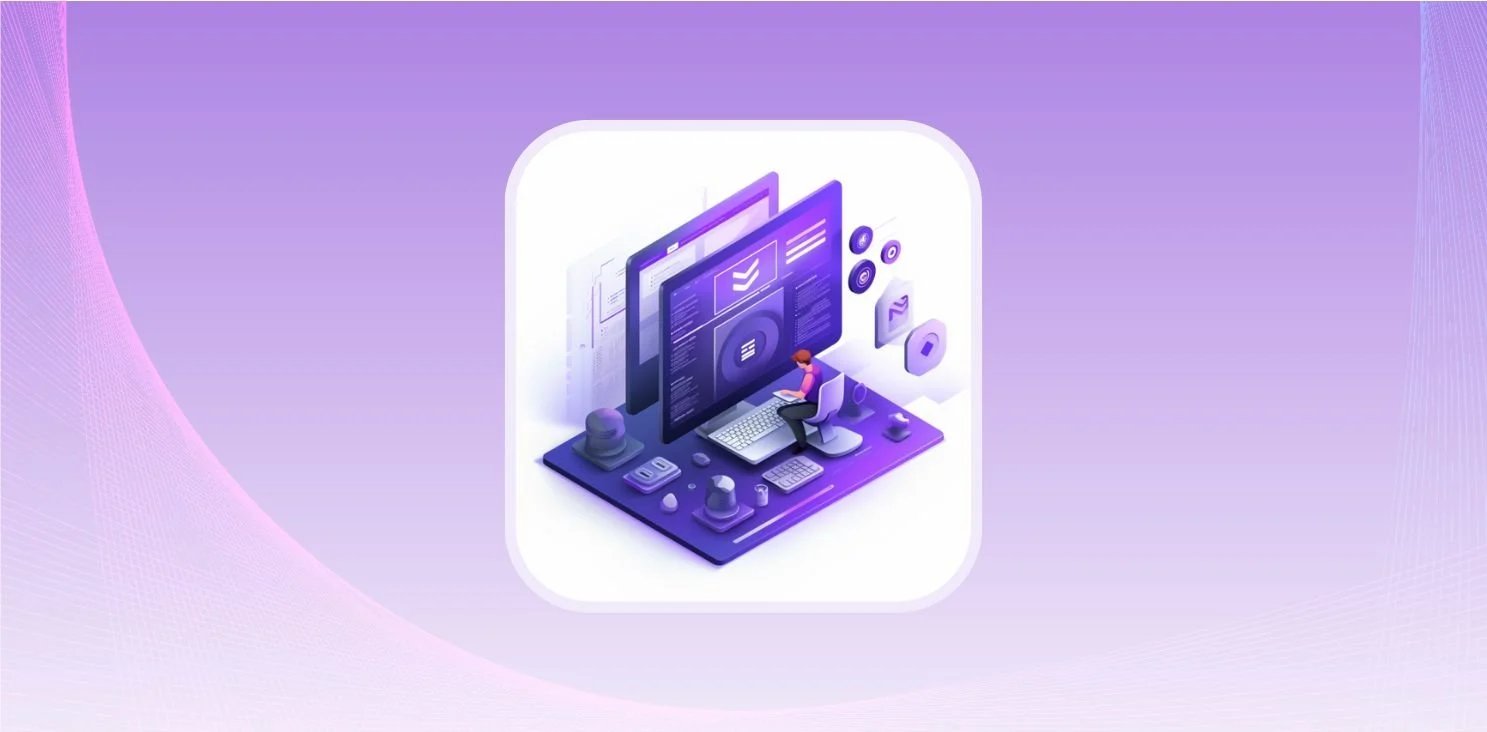
Remember the days of buggy apps? The crashes, the confusion, the updates that felt like downgrades? Those were the days of "ship it as long as it works." But times have changed.
Low-quality software isn't just an annoyance; it's a disaster waiting to happen. Security breaches, endless bug fixes, and frustrated users—these are the hidden costs of neglecting code quality.
While functionality remains crucial, today's developers know that producing high-quality code is not just a luxury but a necessity.
How do you measure code quality? What are the key metrics and practices that separate bad code from good code? In this article, we'll delve into the essential metrics of code quality, providing practical tips and examples to build software that's functional, sustainable, and a joy to work with.
What Is Code Quality?
Code quality is the degree to which a codebase meets the standards and expectations of its developers, users, and stakeholders.
A codebase with predominantly low-quality code can result in an inefficient use of resources and exposure to software attacks from malicious actors. The effects of low-quality code damage the reputation and trust of the software and its developers, ultimately leading to customer dissatisfaction and loss of revenue.
On the other hand, a high-quality codebase prioritizes best practices, which helps promote the efficient use of resources. High-quality code is also easier to read, understand, and modify, making it less prone to errors and more adaptable to changes.
High code quality directly influences the reliability and performance of software, significantly impacting the end-user experience.
Investing in code quality is an investment in the long-term success of software.
Top 7 Code Quality Metrics to Track
Code quality metrics are an attempt to approximate the value of a codebase as either good or bad. Tracking code quality helps identify potential issues early in development, preventing them from becoming critical problems later.
It also helps enhance the codebase's overall structure, making collaboration easier for teams. Here are seven essential metrics to track.
1. Cyclomatic Complexity
Cyclomatic complexity measures how complex a code is based on the number of decision points (linearly independent paths) in the program. Simply, it counts the number of decision paths through your code.
The more control flow (if/else) statements used in a method, the higher the code complexity value. Functions with a high cyclomatic complexity are more difficult to test and more likely to have defects.
def max(a, b, c):
if a > b:
if a > c:
return a
else:
return c
else:
if b > c:
return b
else:
return c
The code snippet above calculates the maximum of three numbers by comparing them using if/else statements. The cyclomatic complexity of the code snippet above is five. There are four decision points (two if statements nested inside each other twice) and one additional path (the exit path after all comparisons).
As you will observe, having too many if/else statements makes the code unnecessarily complex and challenging to read. Also, changes in one of the conditionals can affect the logic of the rest of the code.
We can improve the code quality by reducing the cyclomatic complexity to one using the built-in Python max function, as shown below.
def max(a, b, c):
return max(a, b, c)
You can automate the tracking and measurement of the cyclomatic complexity of your codebase using static code analysis tools like Codacy. With Codacy, you can identify complex functions and refactor them for better readability and maintainability.
2. Code Churn
This metric measures the code added, modified, or deleted over time. It indicates how stable or volatile the codebase is and how much effort teams spend on maintaining or changing the code.
A high code churn means the code is constantly changing, which may imply that the code is poorly designed, the requirements are unclear, or the developers are not following best practices.
Tracking the code churn metric helps developers identify and address the root causes of the frequent changes, improving the code quality.
For example, consider this Git log of a project:
commit 1: Added feature A (100 lines added, 0 lines deleted)
commit 2: Fixed bug in feature A (10 lines added, 10 lines deleted)
commit 3: Refactored feature A (50 lines added, 50 lines deleted)
commit 4: Reverted feature A (100 lines added, 100 lines deleted)
commit 5: Added feature B (200 lines added, 0 lines deleted)
This project has a relatively high code churn because it has 460 lines added and 160 lines deleted in five commits. This stat may indicate that feature A was not well-planned, tested, or implemented and that the project is unstable and prone to errors.
Track your code churn and set a baseline number for your organization. From there, you can find ways to improve the development process by writing clearer user stories, writing unit tests, and performing code reviews before merging.
3. Code Coverage
Code coverage quantifies the percentage of your codebase covered by automated tests. It helps you assess how well your test suite covers your source code and identifies the areas that need more testing. Additionally, when code changes are made, code coverage reports help ensure that any modifications introduced do not unknowingly introduce new issues.
A code repository is considered to have high code coverage if the percentage of coverable lines covered by automated tests in the repository is higher than a predefined threshold. Aim for near-complete test coverage (think 80% and above) for ultimate peace of mind.
There are several types of code coverage tracking metrics, such as function coverage, statement coverage, branch coverage, condition coverage, and line coverage. Codacy uses line coverage, which measures the percentage of coverable lines of code covered by automated tests.
Generally, higher code coverage means higher confidence in the reliability and functionality of your code and a higher chance of identifying bugs before they get to production. Lower code coverage increases the risk of bugs and errors, especially in critical areas.
Some popular code coverage tools by programming languages include Java: JaCoCo, Cobertura; JavaScript: Istanbul, jscoverage; and Python: pytest-cov, Coverage.py.
You can also use code coverage tools to generate reports and visualize the code coverage data, which can help you improve your testing strategy and prioritize your testing efforts.
4. Code Security
The code security metric measures how resistant the code is to attacks and risks. It indicates how well the code protects the data and functionality of the software from unauthorized access, modification, or destruction.
Code security is essential for software reliability, as it helps prevent breaches, errors, and failures that can compromise the integrity, availability, and confidentiality of the software and its users. Poor code security can result in severe consequences, such as data loss, reputation damage, legal liability, and even fatal accidents. Our 2024 State of Software Quality report found that teams are taking security seriously. According to our survey, 84% of development teams conduct regular security audits, and almost 89% have a dedicated security team or person.
High code security means that the code follows the best practices and standards for software security and secure coding, such as the OWASP Top 10, and that it is free of vulnerabilities and flaws, such as SQL injection, broken authentication, sensitive data exposure, cross-site scripting, and insecure deserialization, that can be exploited by malicious actors.
You can employ automated code security tools to scan code for potential vulnerabilities early in the development process, generate reports, and visualize the security data, which can help you improve your security strategy and prioritize your security efforts.
By keeping track of your code’s security scope, you can ensure that your software is secure and compliant with security requirements and regulations.
5. Code Documentation
This metric measures the amount and quality of the documentation accompanying the code. It indicates how well-documented the code is and how easy it is for other developers or users to understand and use it.
Writing code documentation is a key part of software development, as it makes the code clear, easy to maintain, and collaborative for team members. Code documentation explains the logic, functionality, and usage of the code, which is important for comprehending, updating, and enhancing software projects.
High code documentation (>80%) means that the code has consistent comments and comprehensive and up-to-date documentation that covers the purpose, functionality, and usage of the code. This can significantly enhance the project’s understandability and maintainability, as well as facilitate knowledge transfer and troubleshooting.
On the other hand, low or zero-code documentation means that the code lacks sufficient comments and documentation that explain the logic and design of the code. This can lead to several problems, such as:
- Difficulty in reading and comprehending the code, especially if it is complex or poorly written.
- Increased risk of errors and bugs as the code may not behave as expected or may have hidden dependencies or side effects.
- Reduced productivity and efficiency as developers may spend more time trying to figure out the code than working on new features or improvements.
- Impaired collaboration and communication as developers may not be able to share or reuse the code easily or may have conflicting or inconsistent interpretations of the code.
- Loss of knowledge and expertise as developers may leave the project or the organization without documenting their work, leaving behind a legacy of unmaintainable code.
Static code analysis tools like Codacy can help track methods and classes that don’t have the correct comment annotations, helping you identify areas that need better comments. Codacy also provides code quality metrics and suggestions to improve your code documentation and readability. By using Codacy, you can ensure that your code is well-documented and follows best practices for code documentation.
6. Code Duplication
This metric measures the amount of code repeated or copied in different parts of the codebase. It indicates how well the code follows the DRY (Don't Repeat Yourself) principle and how efficient it is. A high code duplication means the code has many redundant or unnecessary parts, increasing the code size, complexity, and maintenance effort.
Example:
Before:
def calculate_book_price(quantity, price):
return quantity * price
def calculate_laptop_price(quantity, price):
return quantity * price
After:
def calculate_product_price(product_quantity, product_price):
return product_quantity * product_price
Using tools like Codacy, you can put a percentage on the number of duplicate codes that exist in at least two different places of the source code of your repository and fix them, improving the code quality.
7. Code Bug Issues
Code bug issues is a metric that measures the number of bugs or defects found in the code per unit of code size (such as lines of code or function points). It indicates how reliable and error-free the code is.
Some of the issues checked by this metric include:
- Code style: Code formatting and syntax problems, such as variable name style and enforcing the use of brackets and quotation marks
- Error-prone: Code that may hide bugs and language keywords that should be used with caution, such as the operator == in JavaScript
- Performance: Code that can have performance problems
- Compatibility: Mainly for frontend code, compatibility problems across different browser versions
- Unused code: Unused variables and methods
- Security: All security problems
A high code bug density means that the code has many bugs or defects, affecting the code's functionality, performance, and security. By tracking the code bug density, developers can monitor the quality of their code and prioritize the issues that need to be resolved.
Track Your Code Quality with Codacy
While learning about your code's quality is crucial, automating the measurements of these metrics is the most important. That's where tools like Codacy shine.
Codacy is a static code analysis tool that helps you improve your code quality by providing useful metrics and feedback. Set up your code quality goals. Codacy will scan your entire repository and assign grades to your branches and files based on a weighted average of four code quality metrics: issues, complexity, duplication, and coverage. These grades range from A to F and help you quickly identify and prioritize the areas of your code that need improvement.
Start your journey toward shipping high-quality code with Codacy today! Get started for free.