10 Common Programming Errors and How to Avoid Them
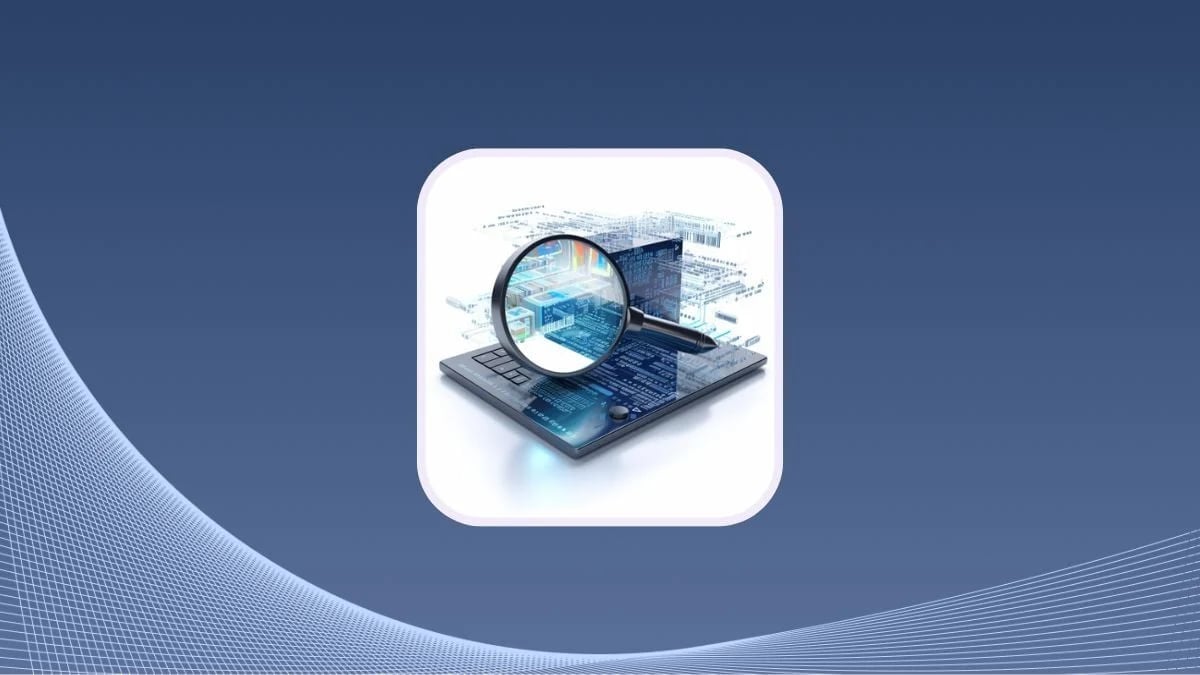
Famed American computer scientist Alan J. Perlis once joked, "There are two ways to write error-free programs; only the third one works."
Writing perfect, error-free code is incredibly challenging, if not impossible. More importantly, writing flawed code is a huge part of the development process, leading to continuous improvement and innovation.
It allows developers to learn, refine their skills, and ultimately produce more robust and efficient code. Each error encountered presents an opportunity for problem-solving and gaining insights into the intricacies of the language and the system you're developing.
Let’s examine 10 common types of errors to understand what they look like, how to spot them, and what you can do to fix and avoid making them in the future.
Syntax Errors
Syntax errors in coding are akin to grammatical mistakes in human language. Just as grammar rules govern the structure of sentences, syntax rules dictate the code structure in programming languages. These rules define how statements, expressions, and elements should be formatted and arranged to create valid and executable code.
Syntax errors in coding occur when the code structure violates the programming language's rules. These errors prevent the compiler or interpreter from correctly interpreting or executing the code.
Some common examples of syntax errors in programming include:
- Missing or mismatched parentheses, brackets, or quotes.
- Forgetting to use semicolons or colons to terminate statements or indicate block structure.
- Incorrect indentation, especially in languages like Python, where indentation is significant.
- Misspelling keywords or identifiers.
- Incorrectly using operators or punctuation.
- Improperly nested control structures or function calls.
- Misusing reserved words or symbols.
- Incorrectly defining function or variable declarations.
- Missing commas or other punctuation in lists or tuples.
- Attempting to use unsupported or invalid characters in identifiers.
Here's an example of a syntax error in Python code:
for i in range(5)
print(i)
This code snippet has a missing colon (:) at the end of the for loop header. In Python, the colon is required to indicate the beginning of the indented code block that belongs to the loop. The absence of the colon results in a syntax error when executing code.
These errors are possible in any language and lead to code that doesn't compile or execute correctly.
Learning the programming language better is the first step to avoid making syntax errors. Familiarize yourself with the syntax rules and conventions of your programming language. Refer to language documentation or tutorials to grasp the correct syntax for different constructs.
Using an Integrated Development Environment (IDE) is another excellent way to decrease syntax errors since most include features like syntax highlighting and real-time error checking.
Static analysis tools can also help you catch and fix syntax rules. These automated tools detect and highlight syntax errors in your code, allowing you to fix these issues before running the code.
Logic Errors
Logic errors occur when the code doesn't behave as expected due to flawed logic or reasoning. Unlike syntax errors, which involve incorrect syntax that prevents code from running, logic errors allow the code to run but produce unintended results.
The fact that the code can run despite these errors makes them some of the most difficult to uncover.
Common examples of logical errors in programming include:
- Flawed logic in conditional (if-else) statements or loops that cause conditions not to be evaluated as expected, leading to incorrect control flow.
- Incorrectly using logical operators (AND, OR, NOT).
- Writing algorithms that solve the wrong problem or using inappropriate algorithms for a given problem.
- Performing operations on variables with incompatible data types, especially when implicit type conversion occurs.
- Writing loops that don't terminate properly.
Here's an example of a logical error in Python code:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
average = total / len(numbers) # Incorrect calculation of average
return average
# Test the function
numbers = [10, 20, 30, 40, 50]
print("Average:", calculate_average(numbers))
In this code, the calculate_average function is intended to calculate the average of a list of numbers. However, there is a logic error in the calculation of the average. Instead of dividing the total by the number of elements in the list (len(numbers)), the code divides by the total number of elements in the list. As a result, the function returns an incorrect average.
Finding and fixing logic errors in programming can be challenging, but there are several recommended methods to help identify and resolve them.
To avoid logic errors, use debugging tools provided by IDEs or standalone debuggers. These tools allow you to go through your code line by line, inspect variables, and track the execution flow, helping you identify where logic errors occur.
Getting coworkers involved can also help. Have a peer review your code to provide fresh insights and catch logic errors that you might have overlooked. Another set of eyes can often spot issues that you may have missed.
Incorporate assert statements into your code to check for conditions that should always be true at specific points. If an assertion fails during execution, it indicates a logic error in your code.
You can also use regression testing methods. Keep a suite of test cases that cover various scenarios and behaviors of your program. Whenever you make changes to your code, rerun these tests to ensure that the changes haven't introduced new logic errors.
Compilation Errors
Compilation errors occur during the process of translating source code into machine-readable instructions by a compiler. These errors occur when the compiler encounters issues that prevent it from successfully converting the source code into executable code and are often caused by other programming errors, like syntax and semantic errors.
They can also be caused by some of the following circumstances:
- When the compiler encounters references to symbols (such as functions, variables, or types) that are declared but not defined in the source code or provided by external libraries or dependencies.
- When there are inconsistencies or mismatches between data types in the source code, like attempting to perform operations on variables of incompatible types or passing arguments of the wrong type to functions.
- Limitations or constraints imposed by the compiler itself, like limitations on the source code size, the complexity of expressions, or the use of unsupported language features or constructs.
- Environmental factors such as compiler settings, system configurations, or platform-specific differences.
Here's an example of a compilation error in C++ code:
#include <iostream>
int main() {
int x = 10;
std::cout << "The value of x is: " << x << std::endl;
// Attempting to use a variable that has not been declared
std::cout << "The value of y is: " << y << std::endl;
return 0;
}
In this example, the code attempts to print the value of a variable y using std::cout, but y has not been declared or defined anywhere in the program. As a result, the compiler will generate a compilation error indicating that y is undeclared or undefined:
error: 'y' was not declared in this scope
std::cout << "The value of y is: " << y << std::endl;
Compilation errors like this one occur when the compiler encounters code that violates the syntax or semantics of the programming language, preventing it from generating executable code. In this case, the error stems from attempting to use a variable (y) that has not been declared or defined in the program's scope.
To avoid compilation errors, start by familiarizing yourself with the syntax rules and conventions of your preferred programming language you're using. Pay close attention to punctuation, keywords, and language constructs to avoid syntax errors.
Use IDEs and text editors with syntax highlighting and error-checking features. Compile your code frequently during development to catch errors early and carefully review compiler error messages and warnings to understand the nature of the errors.
Runtime Errors
Runtime errors, also known as exceptions or execution errors, occur during a program's execution. The manifest when something unexpected happens that prevents the program from continuing normal execution. The compiler does not detect these errors during compilation, but they arise while running the program.
Runtime errors can be particularly frustrating for developers because they can potentially affect end users directly. Unlike compile-time errors, which are caught by the compiler before the program is executed, runtime errors occur during the program's execution and can lead to unexpected crashes, freezes, or incorrect behavior while the program is running.
Runtime errors can occur in various situations, including:
- When the program receives input that it cannot handle or violates its assumptions, for example, trying to parse a non-numeric string as an integer.
- When there are problems with memory allocation, such as attempting to access memory that has not been allocated or accessing a deallocated memory location.
- Performing division by zero—trying to divide a number by zero results in an arithmetic exception, which halts the program's execution.
Here’s an example of a runtime error in Python:
def divide(a, b):
return a / b
# Test the function with invalid input
result = divide(10, 0)
print("Result:", result)
In this code, the divide function attempts to perform a division operation between the parameters a and b. However, when b is set to zero, it leads to a division by zero that results in a runtime error. When you run this code, you'll see the following output:
Traceback (most recent call last):
File "example.py", line 6, in <module>
result = divide(10, 0)
File "example.py", line 2, in divide
return a / b
ZeroDivisionError: division by zero
This output indicates that a ZeroDivisionError occurred during the execution of the divide function at line 2 of the code. The error message provides information about the type of error and the location in the code where it occurred.
Runtime errors can be challenging to diagnose and fix, especially if they occur infrequently or under specific conditions that are difficult to replicate.
To avoid runtime errors, you can start by validating input data to ensure it meets expected criteria and does not cause unexpected behavior or errors during runtime. Perform checks for data type compatibility, range validation, and error handling for invalid input.
Adopt defensive programming techniques by implementing error-handling mechanisms, such as try-except blocks (in languages like Python) or exception-handling constructs (in languages like Java and C++).
Test the program with boundary values, extreme inputs, and edge cases to identify potential runtime errors that may occur under unusual conditions. Consider scenarios such as division by zero, out-of-bounds array access, and integer overflow.
Implement logging and monitoring mechanisms to record runtime errors, exceptions, and unexpected behavior during program execution. Analyze log files and monitoring data to diagnose runtime issues, identify root causes, and track elusive bugs.
Leverage frameworks and community-maintained code, which often undergo extensive testing and refinement by a large user base. You can benefit from the community's collective experience by using these established solutions, reducing the likelihood of encountering common runtime errors. Many frameworks provide built-in error-handling mechanisms, robust error-reporting features, extensive documentation, and community support resources.
Arithmetic Errors
Arithmetic errors are a subset of logic errors that specifically pertain to mistakes or issues related to mathematical calculations or operations within a program. They stem from flawed logical reasoning or assumptions, resulting in incorrect results, program crashes, or unexpected behavior.
These errors can manifest in various ways, including:
- Attempting to divide a number by zero.
- Arithmetic operations involving large numbers can lead to overflow or underflow errors. Overflow is when the calculation result exceeds the maximum representable value for the data type used, while underflow occurs when the result is smaller than the minimum representable value.
- In languages that use floating-point arithmetic, such as Python or Java, arithmetic errors can occur due to the limited precision of floating-point numbers. Operations involving very large or small numbers may result in a loss of precision.
- Converting between different data types or rounding numbers. Truncating decimal values to integers without proper rounding can result in loss of precision or incorrect results.
- Incorrectly using arithmetic operators (+, -, *, /, %).
Here's an example of an arithmetic error in Python:
num1 = 10
num2 = 3
result = num1 / num2 # Incorrect operation, should be multiplication
print("Result:", result)
This code has an incorrect arithmetic operation. While performing a multiplication (num1 * num2), a division operator (/) was used instead.
To mitigate arithmetic errors in coding, developers should validate input data so that it meets the requirements of arithmetic operations. Using appropriate data types and libraries for arithmetic calculations is another way of minimizing precision issues and properly handling large numbers.
Test arithmetic operations thoroughly, including boundary cases and extreme values, to verify correctness and robustness. Remember to follow best practices for mathematical expressions, such as using parentheses to clarify the order of operations and avoiding mixed data types in calculations.
Resource Errors
Resource errors in programming refer to issues related to the allocation, utilization, or availability of system resources such as memory, file handles, network connections, and CPU cycles.
These errors occur when a program exhausts or mismanages available resources, leading to unexpected behavior, performance degradation, or program crashes. Here's how resource errors can occur:
- Failing to release dynamically allocated memory after it's no longer needed, leading to memory leaks.
- Inefficient allocation and management of resources, such as opening too many file handles simultaneously or allocating excessive memory,
- Writing loops that never terminate, either due to logical errors or incorrect loop conditions.
- Failing to handle errors properly, such as neglecting to check return values from resource allocation functions or ignoring exceptions.
- Improper management of concurrent threads or processes, such as excessive thread creation, inadequate synchronization mechanisms, or deadlock conditions.
- Failing to release external resources acquired by the program, such as network sockets, database connections, or system locks.
Here's an example of a resource error in Python:
def open_files():
files = []
for i in range(10000):
file_handle = open('file{}.txt'.format(i), 'w') # Attempting to open too many files
files.append(file_handle)
# Attempting to open too many files
open_files()
In this code, the open_files function attempts to open many files (10,000 in this example) in a loop. However, opening too many files simultaneously can lead to file handle exhaustion, a common resource error. When you run this code, Python will raise a FileNotFoundError or a FileExistsError, indicating that the program encountered an error while opening the files due to resource limitations.
Here's what the output may look like:
Traceback (most recent call last):
File "example.py", line 8, in <module>
open_files()
File "example.py", line 5, in open_files
file_handle = open('file{}.txt'.format(i), 'w') # Attempting to open too many files
OSError: [Errno 24] Too many open files: 'file9999.txt'
This error message shows that the program encountered a Too many open files error while attempting to open one of the files, indicating a resource error related to file handle exhaustion.
Implement proper resource management practices to mitigate resource errors, including releasing resources when no longer needed. Monitor resource usage and set appropriate limits to prevent excessive system resource consumption.
Be efficient with algorithms and data structures to decrease resource consumption and optimize performance. Test the application under realistic load conditions and stress test scenarios to identify and address potential resource limitations or bottlenecks.
Interface Errors
Interface errors often indicate a disconnect or mismatch between how a program is intended to be used (as per its design or specification) and how it is actually used or interacted with by users, other software components, or systems—particularly APIs.
An interface error occurs when there are mismatches, misunderstandings, or incorrect assumptions about how components communicate with each other or how data is exchanged through interfaces.
This discrepancy can stem from various factors, including:
- Developers misinterpreting or misunderstanding the requirements or expectations for how users or other components should interact with the program's interface.
- Incomplete or ambiguous interface specifications that cause developers to make incorrect interface behavior or requirements assumptions.
- Not properly communicating or incorporating new program requirements or usage patterns into the program's interface design.
- Poor communication between stakeholders, such as developers, users, and project managers, that leads to a misalignment of expectations, incomplete requirements documentation, or lack of feedback and clarification during the development process.
While interface errors are more commonly conceptual or architectural issues rather than specific code errors, here’s an example of how a misunderstanding of interface specifications can lead to errors in code usage.
Consider a scenario where two modules or components interact with each other through a defined interface, such as a function call with specified parameters. One module expects a certain data format or range of values for the input parameters, while the other module inadvertently passes invalid or out-of-range values.
Here's some example code in Python:
Module A defines a function calculate_average that expects a list of numbers as input:
# Module A
def calculate_average(numbers):
if not numbers:
return 0
total = sum(numbers)
return total / len(numbers)
Module B, which interacts with Module A, mistakenly passes an empty list to calculate_average:
# Module B
from module_a import calculate_average
data = [] # Empty list
average = calculate_average(data)
print("Average:", average)
In this example, Module B incorrectly passes an empty list data to the calculate_average function from Module A. Module A, however, does not handle the case of an empty list properly and attempts to divide by zero when calculating the average. This results in a runtime error, a ZeroDivisionError, which we've already discussed.
To mitigate interface errors in programming, focus on thoroughly understanding the specifications and documentation of interfaces or APIs they interact with. Validate input parameters and ensure compatibility between interacting components.
Conduct comprehensive testing, including integration testing and interoperability testing, to validate the correctness and reliability of interface interactions. Communicate effectively with other developers or teams involved in software integration to ensure alignment and mutual understanding of interface requirements and expectations.
Linker Errors
A linker programming error occurs during the compilation process's linking phase, typically in languages like C, C++, or other compiled languages. It happens when the compiler attempts to combine multiple object files or libraries into a single executable or shared library but encounters issues with resolving symbols or references between them.
Common situations that lead to linker errors include:
- A compiler encountering references to symbols (functions, variables, or objects) that are declared but not defined in any of the linked object files or libraries.
- A compiler encountering multiple definitions of the same symbol across different object files or libraries. \
- Missing or incompatible library dependencies.
Here's an example of a linker error in C++ code:
// File: main.cpp
#include <iostream>
// Declaration of a function defined in another file
void printMessage();
int main() {
printMessage(); // Calling the function
return 0;
}
// File: functions.cpp
#include <iostream>
// Definition of the function
void printMessage() {
std::cout << "Hello, world!" << std::endl;
If you compile and link these two files separately, you may encounter a linker error because the linker can't find the definition of the printMessage() function in the main.cpp file.
To fix this error, you must ensure that both source files are compiled and linked. You can do this by compiling both files and then connecting them:
g++ -c main.cpp -o main.o
g++ -c functions.cpp -o functions.o
g++ main.o functions.o -o my_program
To resolve linker errors in programming, start by ensuring that all symbols referenced in the code are properly defined and implemented in the source files or linked libraries. Check for duplicate symbol definitions and resolve conflicts by removing or consolidating redundant code.
Verify that all required libraries and dependencies are correctly linked and accessible to the linker. Pay attention to compiler options and flags, ensuring they are consistent and appropriate for the project's requirements.
Semantic Errors
Semantic errors are similar to logic errors in that semantic errors occur when mistakes are made in the program's behavior or logic, but they are not exactly the same. Semantic errors refer to errors in the meaning or interpretation of the code, where the code does not correctly convey the intended functionality or behavior. These errors are more subtle and more complex to detect than syntax or logic errors.
Some examples of semantic errors include:
- Misinterpreting or misunderstanding the requirements or specifications of a program.
- Flaws in algorithm design or implementation.
- Assigning or manipulating variables of incompatible data types.
- Improper usage of external libraries or APIs due to misunderstandings of their functionality or incorrect integration into the codebase.
- Lack of clear and comprehensive documentation, comments, or code annotations.
Here's an example of a semantic error in Python code:
# Example of a Semantic Error
def calculate_circle_area(radius):
return 3.14 * radius * radius # Incorrect formula for calculating circle area
# Calculate the area of a circle with radius 5
radius = 5
area = calculate_circle_area(radius)
print("Area of the circle:", area)
This semantic error may go unnoticed during compilation or execution because the code still runs without raising any syntax errors or runtime exceptions. However, the calculated area will be incorrect due to the flawed formula used in the function, resulting in incorrect results.
To fix this semantic error, the correct formula for calculating the area of a circle (π * radius * radius) should be used in the calculate_circle_area function:
def calculate_circle_area(radius):
return 3.14 * radius * radius # Correct formula for calculating circle area
By using the correct formula, the function will accurately compute the area of a circle based on the given radius, eliminating the semantic error and producing correct results.
Semantic errors can be challenging to identify and debug because they often involve subtle flaws in understanding or interpreting the code's intended functionality.
Using explicit, descriptive variable and function names is a simple yet powerful way to avoid semantic errors. Before writing code, it’s also important to ensure that you have a clear understanding of the requirements. Communicate with stakeholders to clarify any ambiguities and verify that your understanding aligns with their expectations.
Adopt a consistent coding style and adhere to established coding standards and best practices. Consistency reduces cognitive overhead and minimizes the likelihood of misinterpretation or misunderstanding by other developers.
Time Limit Exceeded Errors
A time exceeded error, also known as a timeout error, occurs when a program or operation takes longer to complete than the allotted time limit. This error typically arises in scenarios with constraints on the maximum duration allowed for a specific task, such as network requests, computational algorithms, or system operations.
The following circumstances often cause these errors:
- When a program or operation takes longer to execute than expected or allowed due to various factors, such as inefficient algorithms, resource contention, or large input sizes, leading to extended processing times.
- When a task becomes blocked or waits indefinitely for a resource or event to occur due to synchronization issues, deadlock conditions, or unexpected delays in resource availability.
- When there are delays or timeouts in sending or receiving data over the network due to network congestion, packet loss, or slow response times from remote servers or services.
- Resource exhaustion, like running out of memory, CPU time, or other system resources required to complete a task within the specified time frame.
- Insufficient hardware resources, misconfigured network settings, or unreliable third-party services.
Here's an example of a time exceeded error in Python code using a simple function that performs a computationally intensive operation:
import time
# Function to perform a computationally intensive operation
def compute_factorial(n):
factorial = 1
for i in range(1, n + 1):
factorial *= i
return factorial
# Calculate factorial of a large number (e.g., 10,000)
number = 10000
start_time = time.time()
result = compute_factorial(number)
end_time = time.time()
# Check if the operation exceeded a time limit (e.g., 1 second)
time_limit = 1.0 # 1 second
elapsed_time = end_time - start_time
if elapsed_time > time_limit:
print(f"Time exceeded error: Operation took {elapsed_time} seconds, which exceeded the time limit of {time_limit} seconds.")
else:
print("Factorial:", result)
In this example, the compute_factorial function calculates the factorial of a given number. When calculating the factorial of a large number (e.g., 10,000), the operation may take a significant amount of time to complete.
We measure the elapsed time for the operation using the time to simulate a time exceeded error.time() function before and after the computation. If the elapsed time exceeds a predefined time limit (e.g., 1 second), we consider it a time exceeded error and print an error message indicating that the operation took longer than the allowed time limit.
You can adjust the input number or the time limit to observe how the code behaves under different scenarios. Increasing the input number or decreasing the time limit may increase the likelihood of encountering a time exceeded error.
To address time exceeded errors, focus on improving the efficiency and performance of algorithms to reduce processing times and minimize the likelihood of timeouts. Use timeout mechanisms or timeouts to limit the duration of operations and prevent indefinite blocking or waiting.
Monitor system performance and resource utilization to uncover potential bottlenecks or issues that may lead to time exceeded errors. Implement retry and backoff strategies to handle transient failures or network delays gracefully and mitigate the impact of timeouts.
Finally, timeout parameters and thresholds can be adjusted based on the system or application's specific requirements and characteristics.
Leverage Static Code Analysis Tools to Minimize Programming Errors
Integrating static code analysis tools into your development workflow is one of the best ways to automate the process of reducing programming errors. They help you preemptively identify code smells before they impact production environments. These tools can also save you time. According to our 2024 State of Software Quality report, 58% of developers say not having enough time is the single most common challenge faced during code reviews.
Codacy offers comprehensive analysis capabilities that empower developers to detect and address potential errors early in the development lifecycle. By integrating Codacy into the development workflow, teams can enhance code quality, streamline collaboration, and deliver more reliable and robust software to their users.
Sign up for a free trial today.