11 Common Java Vulnerabilities and How to Avoid Them
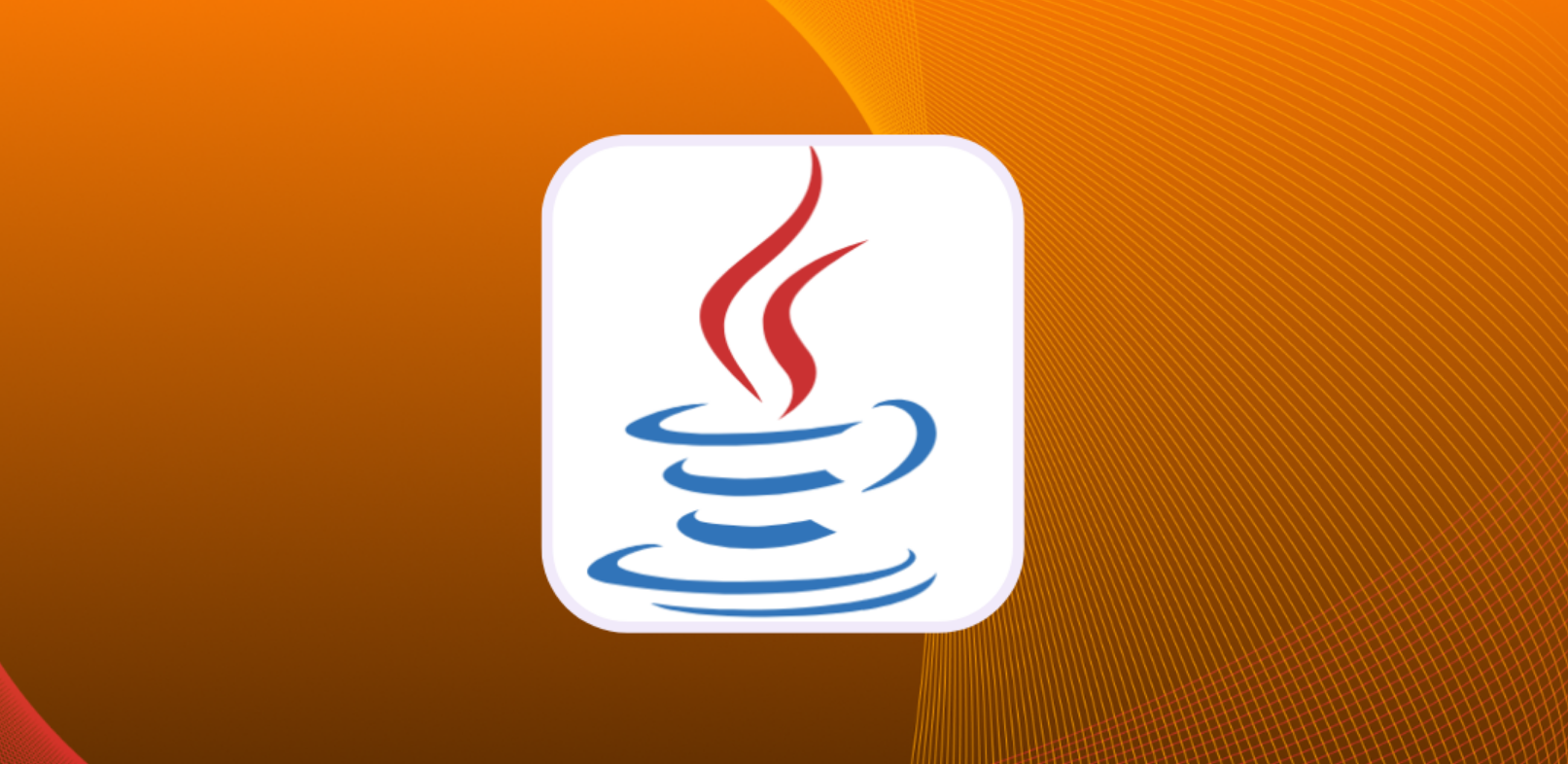
Java is a cornerstone of modern software development, powering everything from enterprise-level applications to Android apps and large-scale data processing systems.
According to the TIOBE Index for June 2024, Java is a top-five most popular programming language. Its platform independence, robust performance, and extensive library support have made it a preferred choice for developers worldwide.
However, Java’s widespread use makes it a prime target for security vulnerabilities. Cyberattacks are becoming increasingly sophisticated, and software vulnerabilities can lead to data breaches, monetary losses, and reputational damage.
If your application runs on Java, ensuring its security is a technical necessity and a fundamental aspect of responsible software development. Let’s explore 11 common security vulnerabilities in Java applications, highlighting how they arise, their potential impact, and, most importantly, how they can be mitigated.
1. Injection Attacks
Injection attacks are a broad class of security vulnerabilities that occur when an attacker can inject malicious input into a program, causing it to execute unintended commands or access unauthorized data. Java applications can be particularly vulnerable to several types of injection attacks, including:
- SQL injection: Injecting malicious SQL queries through user inputs to manipulate database operations. Statista states SQL injections were the most common web application critical vulnerability found globally in 2023.
- LDAP injection: Exploiting unvalidated user input in LDAP queries to access or modify directory services.
- Command injection: Injecting arbitrary commands into a system shell, leading to unauthorized command execution.
- XPath injection: Manipulating XPath queries used to navigate XML documents.
- NoSQL injection: Targeting NoSQL databases by injecting malicious code into database queries.
Injection attacks typically occur due to improper handling of user inputs. When inputs are directly combined into queries or commands without proper validation or sanitization, attackers can craft malicious inputs that alter the application's intended behavior.
For example, in an SQL injection attack, the attacker inserts a malicious SQL query through a user input field, which the database then executes. This can lead to a complete database compromise.
Command injections inject system commands via input fields passed to the operating system's command shell. This can lead to unauthorized command execution, allowing attackers to take control of the server.
Some best practices for preventing injection attacks include:
- Input validation and sanitization: Ensure all user inputs are appropriately validated and sanitized. Only allow expected input formats and reject anything that deviates from those expectations.
- Parameterized queries: Use parameterized queries (prepared statements) to separate query logic from data. This ensures user inputs are treated as data, not executable code.
- ORM frameworks: Object-relational mapping (ORM) frameworks often provide built-in protection against injection attacks.
- Stored procedures: Use them instead of dynamic queries whenever possible, as they can help isolate user input from query execution.
- Least privilege: Apply the least privilege principle to database accounts and other services, ensuring they have only the necessary permissions.
Example of Java Code Vulnerable to SQL Injection
public User getUserByUsername(String username) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
User user = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
stmt = conn.createStatement();
String sql = "SELECT * FROM users WHERE username = '" + username + "'";
rs = stmt.executeQuery(sql);
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Close resources
}
return user;
}
An attacker could exploit this code by entering a malicious username such as ' OR '1'='1, causing the query to return all users:
SELECT * FROM users WHERE username = '' OR '1'='1';
By using a PreparedStatement, you separate the query structure from the data, ensuring that user input is treated as data and not as part of the SQL command. This effectively prevents SQL injection attacks.
public User getUserByUsername(String username) {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
User user = null;
try {
conn = DriverManager.getConnection(DB_URL, DB_USER, DB_PASSWORD);
String sql = "SELECT * FROM users WHERE username = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, username);
rs = pstmt.executeQuery();
if (rs.next()) {
user = new User();
user.setId(rs.getInt("id"));
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// Close resources
}
return user;
}
2. Cross-Site Scripting (XSS)
XSS can allow attackers to inject malicious scripts into pages that other users view. Scripts are executed in the context of the user's browser, allowing attackers to steal sensitive information, hijack user sessions, or perform actions on behalf of the user. Several types of common cross-site scripting attacks are:
- Stored XSS: A malicious script is permanently stored on the target server, like a database, and is delivered to users whenever they request the stored data.
- Reflected XSS: A malicious script is reflected off a web server, typically in error messages or search results, and is immediately returned to the user who submitted the input.
- DOM-based XSS: A malicious script is executed by modifying the DOM (Document Object Model) environment in the user's browser.
XSS attacks occur when an application has untrusted data in a page without proper validation or escaping. This can happen through user input fields, such as forms, search bars, and comment sections, where users can enter data.
Additionally, URL parameters, data passed through the URL query string, and dynamic content generation—content generated based on user input without proper sanitization—can be sources of XSS vulnerabilities. When this untrusted data is rendered in the browser without adequate sanitization, the browser executes the injected script with the same privileges as the legitimate content, leading to various malicious outcomes.
Preventing XSS is all about ensuring that all user inputs are properly validated, sanitized, and escaped. A few best practices for this include:
- Input validation: Validating all user inputs to ensure they conform to expected formats and reject any inputs that contain potentially malicious content.
- Output encoding: Encoding all user inputs before rendering them in the browser. This ensures that special characters are treated as data rather than executable code. Use context-specific encoding functions:
- HTML encoding for data to be placed in HTML content.
- JavaScript encoding for data to be placed in JavaScript contexts.
- URL encoding for data to be placed in URLs.
- Content security policy (CSP): Implement a CSP to restrict from which source content can be loaded and executed. This can mitigate the impact by preventing the execution of unauthorized scripts.
- HTTP-only cookies: Set cookies to HTTP-only to prevent client-side scripts from accessing them, reducing the risk of session hijacking via XSS.
Example of Java Code Vulnerable to XSS
@WebServlet("/greeting")
public class GreetingServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Hello, " + name + "!</h1>");
out.println("</body></html>");
}
}
If an attacker submits a malicious input such as <script>alert('XSS');</script>, it will be executed in the user’s browser:
<html><body>
<h1>Hello, <script>alert('XSS');</script>!</h1>
</body></html>
By encoding the user input before including it in the HTML response, you ensure that special characters are treated as plain text, preventing the execution of any injected scripts. Using dedicated libraries like OWASP's Java Encoder library is recommended for more robust encoding practices.
@WebServlet("/greeting")
public class GreetingServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String name = request.getParameter("name");
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Hello, " + encodeForHTML(name) + "!</h1>");
out.println("</body></html>");
}
private String encodeForHTML(String input) {
if (input == null) {
return null;
}
return input.replace("&", "&")
.replace("<", "<")
.replace(">", ">")
.replace("\"", """)
.replace("'", "'")
.replace("/", "/");
}
}
3. Cross-Site Request Forgery (CSRF)
Cross-site request forgery (CSRF) forces an end user to perform unwanted actions on an application where they are currently authenticated.
By exploiting a web application's trust in the user's browser, an attacker can trick the user into making requests that perform actions such as changing account details, making purchases, or even transferring funds. These requests are executed with the same permissions as the authenticated user, making CSRF a powerful and dangerous attack vector.
These attacks occur when a malicious website or other medium causes a user’s browser to perform unwanted actions on a trusted site. Since browsers automatically include credentials such as session cookies with each request to a web application, the attacker can leverage these credentials to perform actions without consent.
For example, an attacker might embed a malicious link or script in a web page, email, or even a forum post, which, when clicked or executed by the user, sends a request to a target application with the user's session information, resulting in unauthorized actions being carried out.
Preventing CSRF involves implementing several security measures to ensure that requests are legitimate and intentional, including:
- Anti-CSRF tokens: Use anti-CSRF tokens unique to each session and request. These tokens should be included in forms and verified on the server side before processing state-changing requests. Since the attacker cannot predict or obtain the token, they cannot forge valid requests.
- SameSite cookie attribute: Set the SameSite attribute for cookies to Strict or Lax to prevent browsers from sending cookies along with cross-site requests. This limits attackers' ability to perform actions using the user's session.
- Referer and Origin header validation: Validate the Referer and Origin headers to ensure requests come from trusted sources. This helps to confirm that the request originated from your site.
- Double submit cookies: Combine CSRF tokens stored in cookies with those sent as request parameters. The server and client must match the tokens to verify the request's legitimacy.
- User interaction validation: To confirm the user's intent, require additional user interaction for sensitive actions, such as re-entering passwords or completing CAPTCHA challenges.
Example of Java Code Vulnerable to CSRF
@WebServlet("/transfer")
public class TransferServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String amount = request.getParameter("amount");
String recipient = request.getParameter("recipient");
// Perform the transfer
// ...
response.getWriter().println("Transfer successful");
}
}
An attacker could exploit this code by crafting a malicious form on a different website:
<form action="http://vulnerable-website.com/transfer" method="POST">
<input type="hidden" name="amount" value="1000">
<input type="hidden" name="recipient" value="attacker_account">
<input type="submit" value="Click me">
</form>
When the user clicks the submit button, their browser sends the request to the vulnerable website, performing the unauthorized transfer.
To fix the above code using anti-CSRF tokens, you must first generate a token:
@WebServlet("/transfer")
public class TransferServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String csrfToken = generateCSRFToken();
request.getSession().setAttribute("csrfToken", csrfToken);
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<form action='/transfer' method='POST'>");
out.println("<input type='hidden' name='csrfToken' value='" + csrfToken + "'>");
out.println("Amount: <input type='text' name='amount'><br>");
out.println("Recipient: <input type='text' name='recipient'><br>");
out.println("<input type='submit' value='Transfer'>");
out.println("</form>");
}
private String generateCSRFToken() {
return UUID.randomUUID().toString();
}
}
You must then validate the token.
@WebServlet("/transfer")
public class TransferServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String csrfToken = request.getParameter("csrfToken");
String sessionToken = (String)
request.getSession().getAttribute("csrfToken");
request.getSession().getAttribute("csrfToken");
response.setStatus(HttpServletResponse.SC_FORBIDDEN);
response.getWriter().println("CSRF validation failed");
return;
}
String amount = request.getParameter("amount");
String recipient = request.getParameter("recipient");
// Perform the transfer
// ...
response.getWriter().println("Transfer successful");
}
}
By generating a unique CSRF token for each session and including it in the form, you can validate the token on the server side to ensure the request is legitimate. This prevents attackers from being able to perform actions on behalf of authenticated users.
4. Insecure Deserialization
Insecure deserialization occurs when untrusted data instantiates objects during the deserialization process. Here's what that means: When a program reads a file or a stream of data and converts it back into usable objects (like turning a saved game state back into a playable game), that's called deserialization. Instantiating is the act of creating these objects from the saved data.
If an attacker manipulates the data being deserialized, insecure deserialization leads to attacks such as remote code execution, denial of service, and authentication bypass. This type of attack takes advantage of the application's reliance on data that is presumed to be trustworthy, leading to unintended and often harmful behavior when the data is manipulated.
Insecure deserialization happens when an application accepts serialized objects from untrusted sources and deserializes them without proper validation. Attackers can exploit this by modifying serialized data to include malicious payloads.
When the application deserializes this data, it can lead to arbitrary code execution or other unintended actions. For example, suppose an application allows user input to be serialized and deserialized without proper checks. In that case, an attacker can craft a malicious serialized object that, when deserialized, executes harmful code or alters application logic.
Some best practices for careful handling and validation of serialized data include:
- Avoiding deserialization of untrusted data: Ensure that only trusted, authenticated sources can provide serialized data for deserialization.
- Using safe serialization libraries and frameworks: Ones that provide safer serialization mechanisms and have built-in protections against insecure deserialization.
- Implementing integrity checks: Use digital signatures, hashes, or encryption to verify the integrity and authenticity of serialized data before deserializing it to ensure no malicious parties have tampered with it.
- Performing input validation: Validate and sanitize input data before deserialization to ensure that the data conforms to expected formats and does not contain unexpected or harmful content.
Example of Java Code Vulnerable to Insecure Deserialization
import java.io.*;
public class VulnerableDeserialization {
public static void main(String[] args) throws IOException, ClassNotFoundException {
byte[] serializedData = receiveDataFromUntrustedSource();
ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(serializedData));
Object deserializedObject = ois.readObject();
ois.close();
// Use the deserialized object
System.out.println("Deserialized object: " + deserializedObject);
}
private static byte[] receiveDataFromUntrustedSource() {
// Simulate receiving serialized data from an untrusted source
return new byte[]{ /* serialized data */ };
}
}
Utilize libraries such as Jackson or GSON for serialization and deserialization, which offer more control and security features compared to standard Java serialization.
import com.fasterxml.jackson.databind.ObjectMapper;
public class SecureDeserializationWithJackson {
public static void main(String[] args) throws IOException {
byte[] serializedData = receiveDataFromTrustedSource();
ObjectMapper mapper = new ObjectMapper();
MyObject deserializedObject = mapper.readValue(serializedData, MyObject.class);
// Use the deserialized object
System.out.println("Deserialized object: " + deserializedObject);
}
private static byte[] receiveDataFromTrustedSource() {
// Simulate receiving serialized data from a trusted source
return new byte[]{ /* serialized data */ };
}
}
class MyObject {
// Class definition
}
By validating the integrity and authenticity of serialized data and using secure libraries, you can mitigate the risk of insecure deserialization and protect our applications from potential exploitation.
5. Trust Boundary Violations
Trust boundary violations occur when an application fails to properly distinguish between trusted and untrusted data, allowing untrusted data to influence trusted operations. This can lead to unauthorized access, data tampering, or code injection. A trust boundary is an imaginary line separating different trust levels within an application or system. Data crossing this boundary should be appropriately validated and sanitized to ensure it does not carry malicious content or unauthorized instructions.
Trust boundary violations usually happen when an application accepts input from untrusted sources (e.g., user input, external APIs, or third-party services) and uses it directly where it assumes the data is safe. Examples include:
- Accepting user input and using it in database queries without validation.
- Using data from external APIs to make decisions without verification.
- Passing untrusted data to critical system functions or configurations. For instance, if a web application uses a user's input to determine access levels or permissions without proper checks, it might inadvertently grant elevated privileges to unauthorized users.
To prevent trust boundary violations, input from untrusted sources must always be validated and sanitized. Ensure that the data conforms to expected formats and values.
You can use whitelists to ensure that only expected, safe data is accepted. Reject any data that does not match the predefined safe criteria. Separate the processing of trusted and untrusted data by segregating duties to avoid mixing data from different trust levels without proper validation.
Example of Java Code Vulnerable to Trust Boundary Violations
public class UserRoleManager {
public void assignRole(String username, String role) {
// Assume user roles are stored in a database
Database db = getDatabaseConnection();
db.executeQuery("UPDATE users SET role = '" + role + "' WHERE username = '" + username + "'");
}
}
public class UserController {
public void handleRequest(HttpServletRequest request, HttpServletResponse response) throws IOException {
String username = request.getParameter("username");
String role = request.getParameter("role");
UserRoleManager roleManager = new UserRoleManager();
roleManager.assignRole(username, role);
response.getWriter().println("Role assigned successfully");
}
}
In this code, user input (username and role) is directly used to construct a database query without any validation. This can lead to SQL injection or unauthorized role assignments if an attacker manipulates the input values.
Here’s how to fix the code by validating and sanitizing the input:
public class UserRoleManager {
public void assignRole(String username, String role) throws SQLException {
// Assume user roles are stored in a database
Database db = getDatabaseConnection();
String query = "UPDATE users SET role = ? WHERE username = ?";
PreparedStatement statement = db.prepareStatement(query);
statement.setString(1, role);
statement.setString(2, username);
statement.executeUpdate();
}
}
public class UserController {
public void handleRequest(HttpServletRequest request, HttpServletResponse response) throws IOException {
String username = request.getParameter("username");
String role = request.getParameter("role");
if (!isValidUsername(username) || !isValidRole(role)) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST, "Invalid input");
return;
}
try {
UserRoleManager roleManager = new UserRoleManager();
roleManager.assignRole(username, role);
response.getWriter().println("Role assigned successfully");
} catch (SQLException e) {
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "Database error");
}
}
private boolean isValidUsername(String username) {
return username != null && username.matches("[a-zA-Z0-9._-]+");
}
private boolean isValidRole(String role) {
return role != null && role.matches("[a-zA-Z0-9._-]+");
}
}
This code uses prepared statements to prevent SQL injection and includes input validation methods (isValidUsername and isValidRole) to ensure the inputs conform to expected patterns.
6. Broken Authentication
Broken authentication occurs when an application's authentication mechanisms are improperly implemented, allowing attackers to gain access to accounts or systems. This can lead to account takeover, privilege escalation, and unauthorized access to sensitive information.
Broken authentication can result from poor password management, inadequate session handling, weak authentication methods, or flaws in the login process.
To prevent broken authentication vulnerabilities, strong password policies are necessary:
- Use lockout mechanisms after failed login attempts.
- Use CAPTCHAs.
- Implement and enforce multi-factor authentication (MFA) to add an extra layer of security. Ensure MFA tokens are transmitted and stored securely.
- Store passwords using strong, salted hashing algorithms like bcrypt, PBKDF2, or Argon2. Never store passwords in plain text.
Example of Java Code Vulnerable to Broken Authentication
public class LoginServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
// Simulate checking credentials against a database
if (checkCredentials(username, password)) {
request.getSession().setAttribute("user", username);
response.getWriter().println("Login successful");
} else {
response.getWriter().println("Invalid username or password");
}
}
private boolean checkCredentials(String username, String password) {
// Dummy method: Insecurely compares plain text password
return "admin".equals(username) && "password123".equals(password);
}
}
In this code, the checkCredentials method insecurely compares plain text passwords, and there is no protection against brute force attacks or session hijacking. Additionally, storing and using plain text passwords is a major security flaw.
Here’s how to fix the code by implementing stronger authentication mechanisms:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.HashMap;
import java.util.Map;
public class LoginServlet extends HttpServlet {
private static final Map<String, String> userStore = new HashMap<>();
static {
// Pre-store users with hashed passwords
userStore.put("admin", hashPassword("password123"));
}
protected void doPost(HttpServletRequest request, HttpServletResponse
response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (checkCredentials(username, password)) {
request.getSession().setAttribute("user", username);
response.getWriter().println("Login successful");
} else {
response.getWriter().println("Invalid username or password");
}
}
private boolean checkCredentials(String username, String password) {
// Check if the username exists and the hashed password matches
String storedPasswordHash = userStore.get(username);
return storedPasswordHash != null &&
storedPasswordHash.equals(hashPassword(password));
}
private static String hashPassword(String password) {
try {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hash = md.digest(password.getBytes());
StringBuilder hexString = new StringBuilder(2 * hash.length);
for (byte b : hash) {
hexString.append(String.format("%02x", b));
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
}
This code improves security by hashing passwords using SHA-256 before storing and comparing them. It ensures that passwords are not stored or compared in plain text, reducing the risk of password exposure. However, it's important to note that in production, more robust hashing algorithms, like the aforementioned bcrypt, PBKDF2, or Argon2, should be used to further enhance security.
7. Improper Access Control
Improper access control occurs when an application does not properly restrict access to resources or functionalities based on user roles or permissions. This can allow access to sensitive data, perform restricted actions, or escalate privileges.
Improper access control typically happens when Java developers fail to enforce security policies consistently across the application. This can result from hardcoded credentials, missing authorization checks, or incorrect role configurations.
The most common preventive measures include implementing role-based access control (RBAC) and using a centralized access control mechanism to manage permissions consistently across the application.
In this code, any user can view any other user's profile by providing a userId parameter. No checks exist to ensure the current user has the necessary permissions to view the specified profile.
Example of Java Code Vulnerable to Access Control
public void viewUserProfile(HttpServletRequest request, HttpServletResponse response) throws IOException {
String userId = request.getParameter("userId");
User user = getUserFromDatabase(userId);
response.getWriter().println("User Profile: " + user.getProfile());
In this code, any user can view any other user's profile by providing a userId parameter. There are no checks to ensure that the current user has the necessary permissions to view the specified profile.
Here’s how to fix the code by adding access control checks:
public void viewUserProfile(HttpServletRequest request, HttpServletResponse response) throws IOException {
String userId = request.getParameter("userId");
User currentUser = getCurrentUser(request);
if (!currentUser.hasPermission("VIEW_USER_PROFILE") ||
!currentUser.getId().equals(userId)) {
response.sendError(HttpServletResponse.SC_FORBIDDEN, "Access Denied");
return;
}
User user = getUserFromDatabase(userId);
response.getWriter().println("User Profile: " + user.getProfile());
}
This code retrieves the current user and checks if they have the "VIEW_USER_PROFILE" permission and if the userId matches the current user's ID. If either check fails, the server responds with a "403 Forbidden" error. These access control checks ensure that users can only view their own profiles and have the necessary permissions to do so.
8. Directory Traversal
Directory traversal, also known as path traversal, allows an attacker to access directories and files stored outside the web root folder. By manipulating variables that reference files, such as ../ sequences, an attacker can traverse the directory structure to access restricted files and directories.
Directory traversal occurs when an application fails to sanitize user inputs that specify file paths properly. Attackers can send crafted requests that include path traversal sequences (../). For example, an application might concatenate user input with a base directory path, allowing attackers to navigate to unintended directories.
To prevent directory traversal, ensure user inputs do not contain dangerous sequences like ../. Use regular expressions or libraries to validate file paths. You should also restrict file operations to absolute paths and predefined directories and Implement strict access control checks to verify user permissions before accessing files.
Example of Java Code Vulnerable to Directory Traversal
public void readFile(HttpServletRequest request, HttpServletResponse response) throws IOException {
String fileName = request.getParameter("fileName");
File file = new File("/secure-dir/" + fileName);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
response.getWriter().println(line);
}
reader.close(
}
In this code, the fileName parameter from the HTTP request is directly appended to the base directory path (/secure-dir/). If the fileName parameter contains ../ sequences, an attacker can navigate to parent directories and potentially access sensitive files outside the intended directory. To fix the code, validate the file path:
public void readFile(HttpServletRequest request, HttpServletResponse response) throws IOException {
String fileName = request.getParameter("fileName");
if (!isValidFileName(fileName)) {
response.sendError(HttpServletResponse.SC_BAD_REQUEST, "Invalid file name");
return;
}
File file = new File("/secure-dir/" + fileName);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
response.getWriter().println(line);
}
reader.close();
}
private boolean isValidFileName(String fileName) {
return fileName != null && !fileName.contains("..") &&
fileName.matches("[a-zA-Z0-9._-]+");
}
This code includes a validation method, isValidFileName, which ensures the file name does not contain dangerous sequences like ../ and only includes allowed characters. By validating the file name, the application can prevent directory traversal attacks.
9. XML External Entity (XXE) Attacks
An XML External Entity (XXE) attack occurs when an application processes XML input containing a reference to an external entity. This can provide access to sensitive data or even the execution of malicious code. XXE attacks exploit the ability of XML parsers to process external entities, which can be manipulated to access local files or network resources.
These attacks can occur if you use XML parsers that do not turn off external entity processing by default, allowing the inclusion of external entities in the XML input. Another common cause is accepting XML input from untrusted sources without proper validation or sanitization.
To avoid this vulnerability, configure XML parsers to disable the processing of external entities.
Use secure and updated XML parsers that have built-in protections against XXE attacks. Libraries like StAX (Streaming API for XML) and JAXB (Java Architecture for XML Binding) can be configured for secure XML processing.
Validate and sanitize XML input to ensure that expected formats are adhered to and the input doesn't contain unexpected external entity references. Run Java projects with the minimum necessary permissions to limit the potential impact of an XXE attack. For example, if the application doesn't need to access the file system, ensure it doesn't have the necessary permissions.
Example of Java Code Vulnerable to XXE Attacks
Here’s how to configure XML parsers to disable the processing of external entities in Java:
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setFeature("http://apache.org/xml/features/disallow-doctype-decl", true);
dbf.setFeature("http://xml.org/sax/features/external-general-entities", false);
dbf.setFeature("http://xml.org/sax/features/external-parameter-entities", false);
dbf.setFeature("http://apache.org/xml/features/nonvalidating/load-external-dtd", false);
dbf.setXIncludeAware(false);
dbf.setExpandEntityReferences(false);
10. Encryption Issues
Encryption issues are one of the most severe security vulnerabilities an application can have. These vulnerabilities arise when encryption and hashing are not correctly implemented, leading to potential data leaks and authentication bypass through session spoofing. Proper encryption is crucial for securing sensitive information and ensuring data integrity and confidentiality.
Common mistakes Java developers make when implementing encryption include:
- Using weak algorithms: Employing outdated or weak encryption algorithms, such as MD5 or SHA-1, which are no longer considered secure.
- Using the wrong algorithm: Choosing an inappropriate algorithm for a specific task, such as using a hashing algorithm for encryption or vice versa.
- Creating custom algorithms: Developing custom encryption algorithms instead of well-established, tested, and proven ones.
- Generating weak random numbers: Using weak or predictable sources of randomness for cryptographic operations, such as key generation or nonce creation.
In terms of prevention, everything is in the algorithms, so use strong and proven ones instead of customer algorithms. Always use well-established and widely accepted encryption algorithms, such as AES (Advanced Encryption Standard) for symmetric encryption and RSA (Rivest-Shamir-Adleman) for asymmetric encryption. Ensure algorithms are implemented correctly and updated to the latest standards to avoid known vulnerabilities.
Select the algorithm based on the specific security requirement, such as using hashing algorithms (e.g., SHA-256) for data integrity checks and encryption algorithms for data confidentiality. Understand each algorithm's purpose and use case before integrating it into the Java application.
Example of Java Code Vulnerable to Encryption Issues
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class WeakEncryptionExample {
public static void main(String[] args) throws Exception {
// Generate a DES key
KeyGenerator keyGen = KeyGenerator.getInstance("DES");
SecretKey secretKey = keyGen.generateKey();
// Create a Cipher instance for DES
Cipher cipher = Cipher.getInstance("DES");
// Encrypt data
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] plaintext = "SensitiveData".getBytes();
byte[] ciphertext = cipher.doFinal(plaintext);
System.out.println("Encrypted data: " + new String(ciphertext));
}
}
In this example, the code uses the Data Encryption Standard (DES) algorithm to encrypt data. DES is considered weak by today's security standards due to its small key size (56 bits), making it susceptible to brute-force attacks.
To mitigate this issue, we can replace the DES algorithm with the more secure Advanced Encryption Standard (AES) algorithm, which supports larger key sizes (128, 192, and 256 bits) and is widely accepted as secure.
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class StrongEncryptionExample {
public static void main(String[] args) throws Exception {
// Generate an AES key
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(256); // Specify key size (128, 192, or 256 bits)
SecretKey secretKey = keyGen.generateKey();
// Create a Cipher instance for AES
Cipher cipher = Cipher.getInstance("AES");
// Encrypt data
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] plaintext = "SensitiveData".getBytes();
byte[] ciphertext = cipher.doFinal(plaintext);
System.out.println("Encrypted data: " + new String(ciphertext));
}
}
11. Improper Certificate Validation
Improper certificate validation is a security vulnerability that occurs when a Java application doesn't properly validate the certificates used to establish secure communication channels, such as HTTPS. This can lead to man-in-the-middle (MITM) attacks, where an attacker intercepts and potentially alters the data transmitted between the client and server.
One of the most common mistakes developers make when implementing certificate validation is trusting all certificates. Another is ignoring hostname verification. Failing to verify that the certificate’s hostname matches the server’s hostname could allow an attacker to present a valid certificate for a different hostname, successfully intercepting the communication.
Implementing custom trust managers that skip validation steps or trust all certificates is another common misstep, as is using expired, outdated, or insecure certificates.
To avoid these vulnerabilities, Java developers should always validate the certificate chain to ensure it is issued by a trusted Certificate Authority (CA). Use the default trust manager provided by Java, which performs proper certificate validation.
Ensure that the certificate’s Common Name (CN) or Subject Alternative Name (SAN) matches the server’s hostname by using hostname verification methods provided by Java’s SSL/TLS libraries. Use certificates with robust encryption algorithms, and remember to update them regularly before they expire.
Example of Java Code Vulnerable to Improper Certificate Validation
import javax.net.ssl.*;
import java.security.cert.X509Certificate;
public class InsecureSSLConnection {
public static void main(String[] args) throws Exception {
// Create an insecure trust manager that trusts all certificates
TrustManager[] trustAllCerts = new TrustManager[]{
new X509TrustManager() {
public X509Certificate[] getAcceptedIssuers() {
return null;
}
public void checkClientTrusted(X509Certificate[] certs, String authType) {
}
public void checkServerTrusted(X509Certificate[] certs, String authType) {
}
}
};
// Initialize SSL context with the insecure trust manager
SSLContext sc = SSLContext.getInstance("TLS");
sc.init(null, trustAllCerts, new java.security.SecureRandom());
HttpsURLConnection.setDe
In this example, the code creates a custom TrustManager that trusts all certificates, bypassing critical validation steps. This leaves the application vulnerable to man-in-the-middle (MITM) attacks, as it will accept any certificate presented by a server, even if it is fraudulent.
You can mitigate this issue by enforcing proper certificate validation.
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManagerFactory;
import java.io.InputStream;
import java.security.KeyStore;
public class SecureSSLConnection {
public static void main(String[] args) throws Exception {
// Load the default trust store
KeyStore trustStore = KeyStore.getInstance(KeyStore.getDefaultType());
try (InputStream trustStoreStream =
SecureSSLConnection.class.getResourceAsStream("/path/to/truststore")) {
if (trustStoreStream != null) {
trustStore.load(trustStoreStream,
"truststore-password".toCharArray());
} else {
throw new IllegalArgumentException("Truststore file not found");
}
}
// Initialize the TrustManagerFactory with the loaded trust store
TrustManagerFactory tmf =
TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
tmf.init(trustStore);
// Initialize SSL context with the TrustManagerFactory
SSLContext sc = SSLContext.getInstance("TLS");
sc.init(null, tmf.getTrustManagers(), new
java.security.SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
// Now all HTTPS connections will use this secure trust manager
System.out.println("Secure SSL connection established.");
}
}
Automate Vulnerability Identification with Static Code Analysis Tools
Securing Java applications is a multifaceted challenge that requires diligence, knowledge, and the right tools. One of the most effective ways to identify and mitigate these Java vulnerabilities early in the development process is through static code analysis tools. These tools can automatically scan your codebase, detect security issues, and offer actionable insights to help you resolve them.
By integrating static code analysis into your Java development workflow, you can catch many of these Java vulnerabilities before they make it into production, significantly reducing your application's attack surface.
Codacy offers a comprehensive static code analysis platform that supports over 40 programming languages, including Java. With Codacy, you can continuously monitor your code for Java vulnerabilities, code quality metrics, and adherence to coding standards and best practices. The platform provides detailed reports and AI-aided suggestions for fixes, making it easier for development teams to maintain secure, high-quality code.
Codacy also offers SAST, DAST, and penetration testing tools, a complete suite of security features that can help you identify common vulnerabilities and exposures (CVEs) before they cause problems for your development team.
To see how Codacy works, book a demo with one of our experts today or sign up for a free 14-day trial.