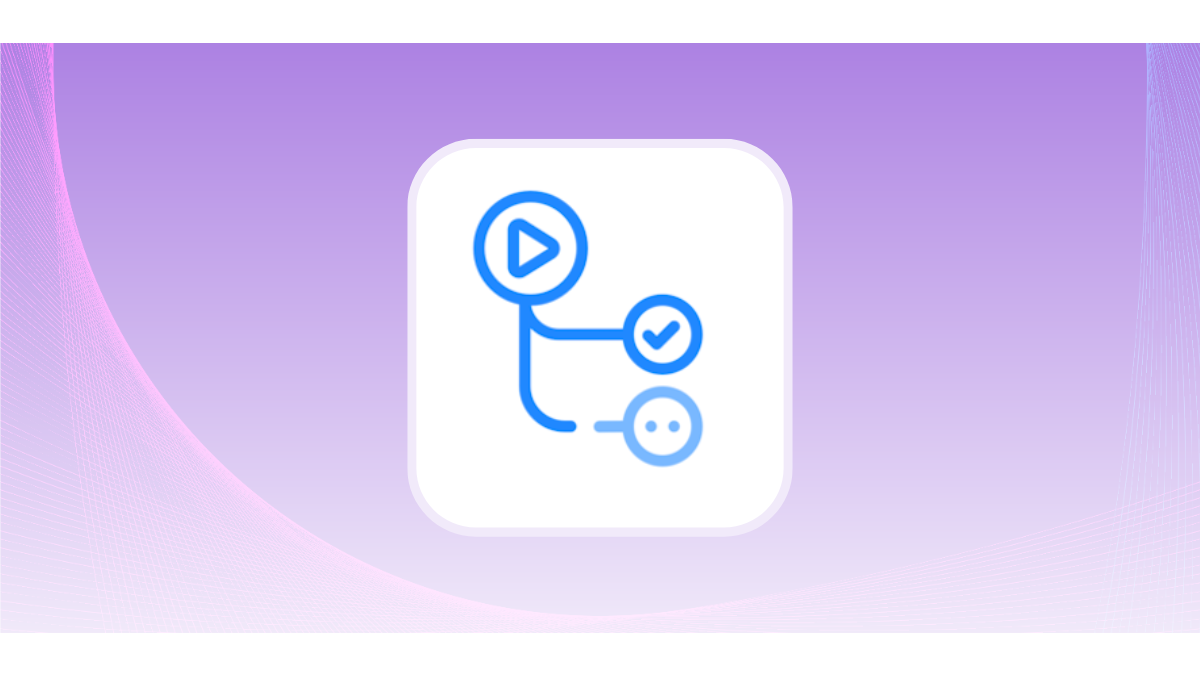
As technology constantly improves, so does the process of shipping software. Gone are the days of having to manually build, test, and deploy code when you need to make a change. Nowadays, this process is automated with the simple click of a button.
GitHub Actions are automated workflows that allow you to define custom Continuous Integration/Continuous Deployment) (CICD) processes directly within your GitHub repository, such as running tests, publishing packages, or deploying an application to production. These workflows can be triggered by various events, such as when you push, pull, or merge to a repository.
In this article, we will explain how to set up GitHub Actions, explore their functionality, and provide a guide on how to test them on GitHub and locally using the act library. With a deep understanding of GitHub Actions, you’ll be able to ship your software more easily than ever before.
How Does GitHub Actions Work?
GitHub Actions operates by first defining workflows, which serve as blueprints outlining the specific steps to be executed for a given task/process. These workflows are triggered automatically by events like code pushes, pull requests, or issue creation.
For example, you can create a workflow to run unit tests when new code is pushed to the repository. Then, another workflow can deploy the code to production when code is merged to the release branch.
Each workflow consists of one or more jobs that run either sequentially or in parallel, depending on your needs. Each job consists of a series of steps that execute either custom scripts or pre-built actions. Pre-built actions are reusable scripts that simplify common tasks, such as setting up environments, installing dependencies, and running tests.
To handle the job execution, GitHub offers virtual machines called "runners" with various operating systems and architectures. The runner checks out your code, runs the specified actions on the specified machine, and reports the results back to GitHub.
Steps to Setup a GitHub Action in Python
To demonstrate how to set up a GitHub Action, we will use a simple Python project that has a main.py file and a test.py file.
The main.py file contains a function that returns the sum of two numbers. The test.py file contains a unit test for the function.
The project structure looks like this:
GitHubActions
├── main.py
└── test.py
The main.py file contains the following code:
def add(x, y):
return x + y
The test.py file contains the following code to test the add function in the main.py file using the unittest library:
import unittest
from main import add
class TestAdd(unittest.TestCase):
def test_add(self):
self.assertEqual(add(1, 2), 3)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(0, 0), 0)
if __name__ == '__main__':
unittest.main()
Now, we can create a repository and set up a GitHub Action for that repository.
1. Create a GitHub Repository for the Project
Go to github.com and log in with your credentials. Create a new repository and push your code to the main branch of this repository using the following commands from your command line:
git init
git add .
git commit -m "first commit"
git remote add origin https://github.com/{yourrepo}/test.git
git branch -M main
git push -u origin main
2. Configure GitHub Action
To create a GitHub Action for this project, we need to create a .github/workflows directory in the root of the repository and add a YAML file with a name of our choice, such as python-ci.yml.
The YAML file defines the workflow configuration, such as the name, the trigger, the jobs, the steps, and the actions. Here is an example of a workflow file that runs the unit tests on every push and pull request to our repository:
# This workflow will install Python dependencies and run tests
name: Python CI
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Python
uses: actions/setup-python@v3
with:
python-version: 3.9
architecture: "x64"
- name: Install dependencies
run: |
python -m pip install --upgrade pip
- name: Run tests
run: |
python test.py
Let’s break down the workflow file:
- The name field gives a descriptive name to the workflow, which will be displayed on the GitHub Actions tab of the repository.
- The on field specifies the events that trigger the workflow. In this case, we use an array to specify that the workflow runs on every push and pull request to any branch.
- The jobs field defines the jobs that run as part of the workflow. Each job has a unique identifier, such as a test and a set of properties. In this case, we only have one job, but we can add more if needed.
- The runs-on field specifies the type of runner to use for the job. In this example, we use ubuntu-latest, which is a version of ubuntu provided by GitHub. We can also use other options, such as windows-latest, macos-latest, or a self-hosted runner.
- The steps field defines the steps that run in the job. Each step can run a command or an action.
- The first step uses the actions/checkout@v3 action, which checks out the repository on the runner. The @v3 specifies the version of the action to use.
- The second step uses the actions/setup-python@v3 action, which sets up the Python environment on the runner. In this case, we use the python-version parameter to specify the version of Python to use, which is 3.9.
- The third step runs a command to install the dependencies for the project using pip.
- The fourth step runs a command to run the tests using the test.py file.
- The first step uses the actions/checkout@v3 action, which checks out the repository on the runner. The @v3 specifies the version of the action to use.
3. Test the GitHub Action on GitHub
To test the GitHub Action, all we need to do is push or pull our latest changes to the GitHub repository, and the action will be triggered automatically. To do that, we can run the following commands:
git add .
git commit -m "second commit"
git push -u origin main
Navigate to the action tab on GitHub and check if the action ran successfully.
4. Testing the GitHub Action Locally Using the Act Library
While GitHub Actions are great for automating your workflows, sometimes you may want to test them locally before pushing them to GitHub. This can save you time and resources and help you debug any issues.
One way to test GitHub Actions locally is to use the act library, which is a command-line tool that allows you to run your workflows on your machine.
To use the act library, you need to have Docker installed and running on your machine. You also need to install the act library, which you can do using one of the methods described here.
For example, on macOS, you can use the following command:
brew install act
Once you have installed the act library, you can use it to run your workflows locally. To do so, you need to navigate to the root of your repository, where the .github/workflows directory is located, and run the act command.
act
This will run the default workflow file, which is the first one found in the .github/workflows directory, using the default event, which is push. You can also specify a different event using the command:
act pull_request
This will run the python-ci.yml workflow file using the pull_request event. If you are running this example using a Mac with the Apple M series chip, you need to specify the container architecture for the runner. You do this by running the command:
act --container-architecture linux/amd64
This will use the ubuntu-latest image from Docker, which is based on the linux/amd 64 container architecture, as a runner to run the GitHub Action locally.
Run the command and check if the GitHub Action runs successfully locally.
Using the act library can help quickly test your GitHub Actions without launching them on GitHub every time.
Write High-Quality Code Using GitHub Actions and Codacy
Codacy is a code review automation tool that helps teams write high-quality code in over 40 languages, such as PHP, JavaScript, Python, Java, and Ruby.
With Codacy, you can customize your code quality standards, code patterns, and quality settings to avoid code issues.
The Codacy GitHub Action supports the following scenarios:
- Analysis with default settings: Checks your code for issues on every commit and pull request and fails the workflow if it finds any.
- Integration with GitHub code scanning: Analyzes your commits and pull requests and uploads the results to GitHub, which displays the identified issues under your repository's Security tab.
- Integration with Codacy for client-side tools: Uses one of Codacy’s client-side tools to analyze your code and sends the results to Codacy. This will show the issues in UI dashboards and can also update the status of your pull requests.
Find out more about how you and your team can leverage Codacy to improve your code quality by visiting the Codacy GitHub Actions marketplace.