Integration with ESLint JavaScript Linter
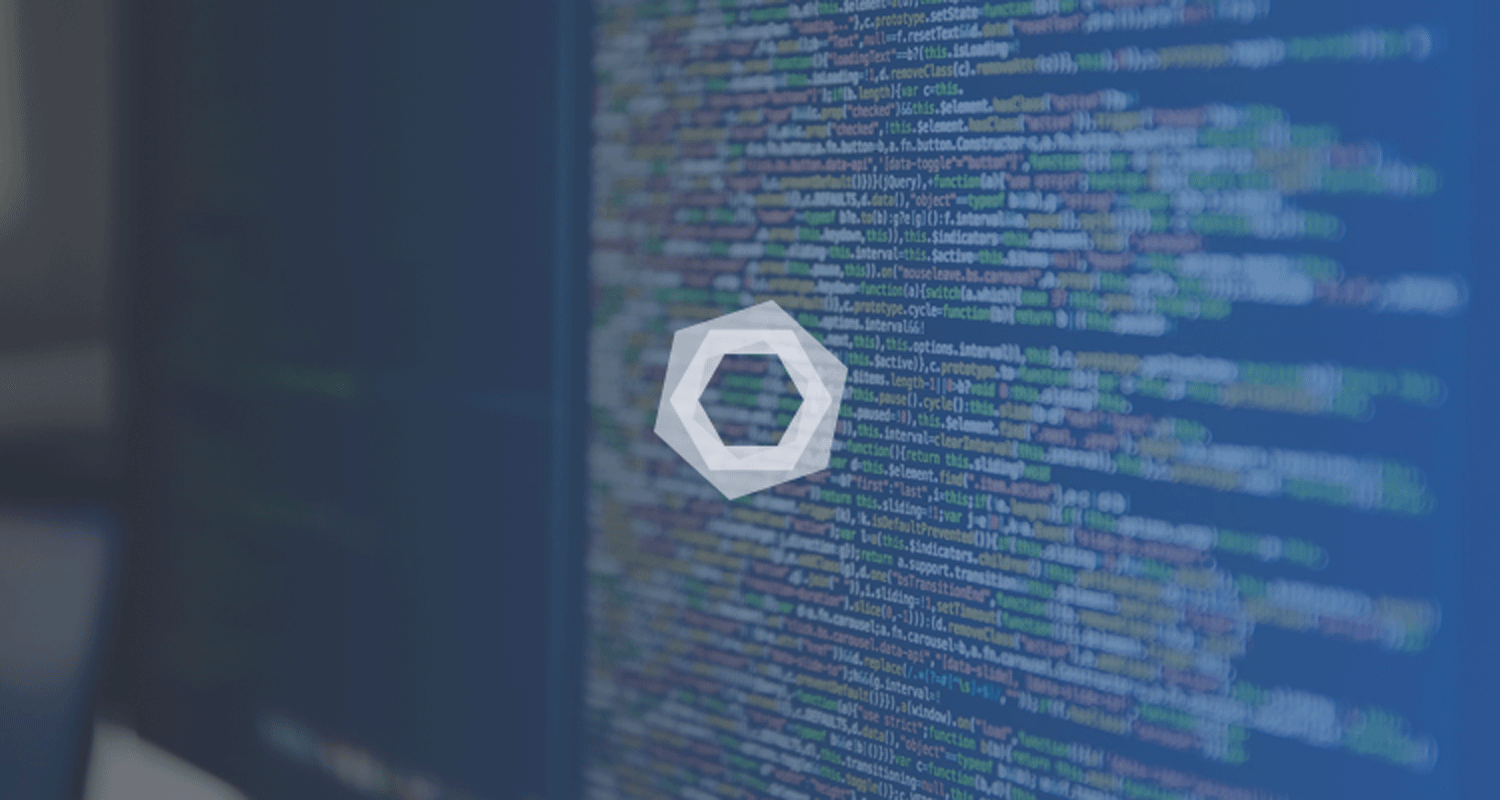
This past year we’ve seen an incredible growth coming from ESLint, a linter on steroids for ECMAScript, JSX and JavaScript code. It quickly became the go-to JavaScript linter for the programming language’s community. The work of Nicholas and the numerous contributors is really impressive.
The original philosophy behind the tool is something that speaks to us greatly as it is completely pluggable. ESLint makes it very easy to write your own plugins and share them with the community. You can find several examples on NPM.
Today I’m happy to announce that ESLint rules are now supported on Codacy. Here are the rules taken from the rules list that you can now enforce on Codacy. Enable them on your projects and share your feedback with us!
ESLint Rules
Possible Errors
The following rules point out areas where you might have made mistakes.
- comma-dangle — disallow or enforce trailing commas (recommended)
- no-cond-assign — disallow assignment in conditional expressions (recommended)
- no-console — disallow use of console in the node environment (recommended)
- no-constant-condition — disallow use of constant expressions in conditions (recommended)
- no-control-regex — disallow control characters in regular expressions (recommended)
- no-debugger — disallow use of debugger (recommended)
- no-dupe-args — disallow duplicate arguments in functions (recommended)
- no-dupe-keys — disallow duplicate keys when creating object literals (recommended)
- no-duplicate-case — disallow a duplicate case label. (recommended)
- no-empty-character-class — disallow the use of empty character classes in regular expressions (recommended)
- no-empty — disallow empty statements (recommended)
- no-ex-assign — disallow assigning to the exception in a catch block (recommended)
- no-extra-boolean-cast — disallow double-negation boolean casts in a boolean context (recommended)
- no-extra-parens — disallow unnecessary parentheses
- no-extra-semi — disallow unnecessary semicolons (recommended) (fixable)
- no-func-assign — disallow overwriting functions written as function declarations (recommended)
- no-inner-declarations — disallow function or variable declarations in nested blocks (recommended)
- no-invalid-regexp — disallow invalid regular expression strings in the RegExp constructor (recommended)
- no-irregular-whitespace — disallow irregular whitespace outside of strings and comments (recommended)
- no-negated-in-lhs — disallow negation of the left operand of an in expression (recommended)
- no-obj-calls — disallow the use of object properties of the global object (Math and JSON) as functions (recommended)
- no-regex-spaces — disallow multiple spaces in a regular expression literal (recommended)
- no-sparse-arrays — disallow sparse arrays (recommended)
- no-unexpected-multiline — Avoid code that looks like two expressions but is actually one
- no-unreachable — disallow unreachable statements after a return, throw, continue, or break statement (recommended)
- use-isnan — disallow comparisons with the value NaN (recommended)
- valid-jsdoc — Ensure JSDoc comments are valid
- valid-typeof — Ensure that the results of typeof are compared against a valid string (recommended)
Best Practices
These are rules designed to prevent you from making mistakes. They either prescribe a better way of doing something or help you avoid footguns.
- accessor-pairs — Enforces getter/setter pairs in objects
- block-scoped-var — treat var statements as if they were block scoped
- complexity — specify the maximum cyclomatic complexity allowed in a program
- consistent-return — require return statements to either always or never specify values
- curly — specify curly brace conventions for all control statements
- default-case — require default case in switch statements
- dot-location — enforces consistent newlines before or after dots
- dot-notation — encourages use of dot notation whenever possible
- eqeqeq — require the use of === and !== (fixable)
- guard-for-in — make sure for-in loops have an if statement
- no-alert — disallow the use of alert, confirm, and prompt
- no-caller — disallow use of arguments.caller or arguments.callee
- no-case-declarations — disallow lexical declarations in case clauses
- no-div-regex — disallow division operators explicitly at beginning of regular expression
- no-else-return — disallow else after a return in an if
- no-empty-label — disallow use of labels for anything other than loops and switches
- no-empty-pattern — disallow use of empty destructuring patterns
- no-eq-null — disallow comparisons to null without a type-checking operator
- no-eval — disallow use of eval()
- no-extend-native — disallow adding to native types
- no-extra-bind — disallow unnecessary function binding
- no-fallthrough — disallow fallthrough of case statements (recommended)
- no-floating-decimal — disallow the use of leading or trailing decimal points in numeric literals
- no-implicit-coercion — disallow the type conversions with shorter notations
- no-implied-eval — disallow use of eval()-like methods
- no-invalid-this — disallow this keywords outside of classes or class-like objects
- no-iterator — disallow usage of __iterator__ property
- no-labels — disallow use of labeled statements
- no-lone-blocks — disallow unnecessary nested blocks
- no-loop-func — disallow creation of functions within loops
- no-magic-numbers — disallow the use of magic numbers
- no-multi-spaces — disallow use of multiple spaces (fixable)
- no-multi-str — disallow use of multiline strings
- no-native-reassign — disallow reassignments of native objects
- no-new-func — disallow use of new operator for Function object
- no-new-wrappers — disallows creating new instances of String,Number, and Boolean
- no-new — disallow use of the new operator when not part of an assignment or comparison
- no-octal-escape — disallow use of octal escape sequences in string literals, such as var foo = “Copyright 251”;
- no-octal — disallow use of octal literals (recommended)
- no-param-reassign — disallow reassignment of function parameters
- no-process-env — disallow use of process.env
- no-proto — disallow usage of __proto__ property
- no-redeclare — disallow declaring the same variable more than once (recommended)
- no-return-assign — disallow use of assignment in return statement
- no-script-url — disallow use of javascript: urls.
- no-self-compare — disallow comparisons where both sides are exactly the same
- no-sequences — disallow use of the comma operator
- no-throw-literal — restrict what can be thrown as an exception
- no-unused-expressions — disallow usage of expressions in statement position
- no-useless-call — disallow unnecessary .call() and .apply()
- no-useless-concat — disallow unnecessary concatenation of literals or template literals
- no-void — disallow use of the void operator
- no-warning-comments — disallow usage of configurable warning terms in comments — e.g. TODO or FIXME
- no-with — disallow use of the with statement
- radix — require use of the second argument for parseInt()
- vars-on-top — require declaration of all vars at the top of their containing scope
- wrap-iife — require immediate function invocation to be wrapped in parentheses
- yoda — require or disallow Yoda conditions
Strict Mode
These rules relate to using strict mode.
- strict — controls location of Use Strict Directives
Variables
These rules have to do with variable declarations.
- init-declarations — enforce or disallow variable initializations at definition
- no-catch-shadow — disallow the catch clause parameter name being the same as a variable in the outer scope
- no-delete-var — disallow deletion of variables (recommended)
- no-label-var — disallow labels that share a name with a variable
- no-shadow-restricted-names — disallow shadowing of names such as arguments
- no-shadow — disallow declaration of variables already declared in the outer scope
- no-undef-init — disallow use of undefined when initializing variables
- no-undef — disallow use of undeclared variables unless mentioned in a /*global */ block (recommended)
- no-undefined — disallow use of undefined variable
- no-unused-vars — disallow declaration of variables that are not used in the code (recommended)
- no-use-before-define — disallow use of variables before they are defined
Node.js and CommonJS
These rules are specific to JavaScript running on Node.js or using CommonJS in the browser.
- callback-return — enforce return after a callback
- global-require — enforce require() on top-level module scope
- handle-callback-err — enforce error handling in callbacks
- no-mixed-requires — disallow mixing regular variable and require declarations
- no-new-require — disallow use of new operator with the require function
- no-path-concat — disallow string concatenation with __dirname and __filename
- no-process-exit — disallow process.exit()
- no-restricted-modules — restrict usage of specified node modules
- no-sync — disallow use of synchronous methods
Stylistic Issues
These rules are purely matters of style and are quite subjective.
- array-bracket-spacing — enforce spacing inside array brackets (fixable)
- block-spacing — disallow or enforce spaces inside of single line blocks (fixable)
- brace-style — enforce one true brace style
- camelcase — require camel case names
- comma-spacing — enforce spacing before and after comma (fixable)
- comma-style — enforce one true comma style
- computed-property-spacing — require or disallow padding inside computed properties (fixable)
- consistent-this — enforce consistent naming when capturing the current execution context
- eol-last — enforce newline at the end of file, with no multiple empty lines (fixable)
- func-names — require function expressions to have a name
- func-style — enforce use of function declarations or expressions
- id-length — this option enforces minimum and maximum identifier lengths (variable names, property names etc.)
- id-match — require identifiers to match the provided regular expression
- indent — specify tab or space width for your code (fixable)
- jsx-quotes — specify whether double or single quotes should be used in JSX attributes
- key-spacing — enforce spacing between keys and values in object literal properties
- linebreak-style — disallow mixed ‘LF’ and ‘CRLF’ as linebreaks
- lines-around-comment — enforce empty lines around comments
- max-depth — specify the maximum depth that blocks can be nested
- max-len — specify the maximum length of a line in your program
- max-nested-callbacks — specify the maximum depth callbacks can be nested
- max-params — limits the number of parameters that can be used in the function declaration.
- max-statements — specify the maximum number of statement allowed in a function
- new-cap — require a capital letter for constructors
- new-parens — disallow the omission of parentheses when invoking a constructor with no arguments
- newline-after-var — require or disallow an empty newline after variable declarations
- no-array-constructor — disallow use of the Array constructor
- no-bitwise — disallow use of bitwise operators
- no-continue — disallow use of the continue statement
- no-inline-comments — disallow comments inline after code
- no-lonely-if — disallow if as the only statement in an else block
- no-mixed-spaces-and-tabs — disallow mixed spaces and tabs for indentation (recommended)
- no-multiple-empty-lines — disallow multiple empty lines
- no-negated-condition — disallow negated conditions
- no-nested-ternary — disallow nested ternary expressions
- no-new-object — disallow the use of the Object constructor
- no-plusplus — disallow use of unary operators, ++ and —
- no-restricted-syntax — disallow use of certain syntax in code
- no-spaced-func — disallow space between function identifier and application (fixable)
- no-ternary — disallow the use of ternary operators
- no-trailing-spaces — disallow trailing whitespace at the end of lines (fixable)
- no-underscore-dangle — disallow dangling underscores in identifiers
- no-unneeded-ternary — disallow the use of ternary operators when a simpler alternative exists
- object-curly-spacing — require or disallow padding inside curly braces (fixable)
- one-var — require or disallow one variable declaration per function
- operator-assignment — require assignment operator shorthand where possible or prohibit it entirely
- operator-linebreak — enforce operators to be placed before or after line breaks
- padded-blocks — enforce padding within blocks
- quote-props — require quotes around object literal property names
- quotes — specify whether backticks, double or single quotes should be used (fixable)
- require-jsdoc — Require JSDoc comment
- semi-spacing — enforce spacing before and after semicolons
- semi — require or disallow use of semicolons instead of ASI (fixable)
- sort-vars — sort variables within the same declaration block
- space-after-keywords — require a space after certain keywords (fixable)
- space-before-blocks — require or disallow a space before blocks (fixable)
- space-before-function-paren — require or disallow a space before function opening parentheses (fixable)
- space-before-keywords — require a space before certain keywords (fixable)
- space-in-parens — require or disallow spaces inside parentheses
- space-infix-ops — require spaces around operators (fixable)
- space-return-throw-case — require a space after return, throw, and case (fixable)
- space-unary-ops — require or disallow spaces before/after unary operators (fixable)
- spaced-comment — require or disallow a space immediately following the // or /* in a comment
- wrap-regex — require regex literals to be wrapped in parentheses
ECMAScript 6
These rules are only relevant to ES6 environments.
- arrow-body-style — require braces in arrow function body
- arrow-parens — require parentheses in arrow function arguments
- arrow-spacing — require space before/after arrow function’s arrow (fixable)
- constructor-super — verify calls of super() in constructors
- generator-star-spacing — enforce spacing around the * in generator functions (fixable)
- no-arrow-condition — disallow arrow functions where a condition is expected
- no-class-assign — disallow modifying variables of class declarations
- no-const-assign — disallow modifying variables that are declared using const
- no-dupe-class-members — disallow duplicate name in class members
- no-this-before-super — disallow use of this/super before calling super() in constructors.
- no-var — require let or const instead of var
- object-shorthand — require method and property shorthand syntax for object literals
- prefer-arrow-callback — suggest using arrow functions as callbacks
- prefer-const — suggest using const declaration for variables that are never modified after declared
- prefer-reflect — suggest using Reflect methods where applicable
- prefer-spread — suggest using the spread operator instead of .apply().
- prefer-template — suggest using template literals instead of strings concatenation
- require-yield — disallow generator functions that do not have yield
Edit: We just published an ebook: “The Ultimate Guide to Code Review” based on a survey of 680+ developers. Enjoy!
For more on ESLint from us check out our ESLint archive
About Codacy
Codacy is used by thousands of developers to analyze billions of lines of code every day!
Getting started is easy – and free! Just use your GitHub, Bitbucket or Google account to sign up.