A Deep Dive into Static Code Analysis Tools
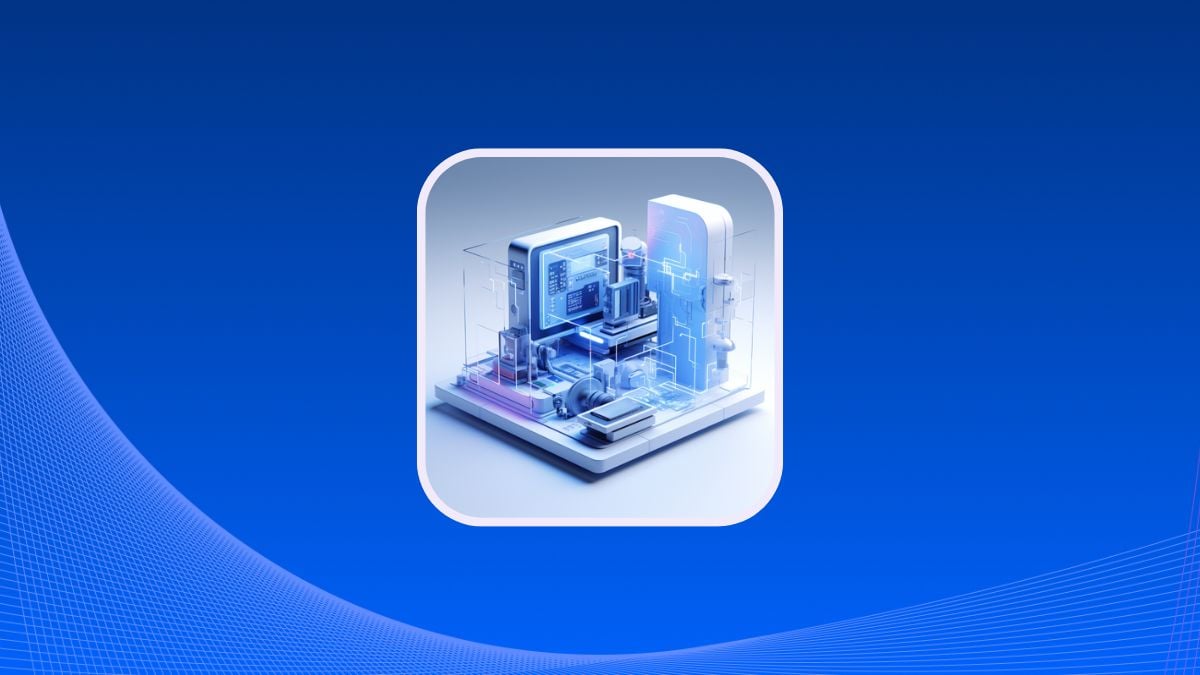
Static code analysis is a crucial aspect of modern software development. At its core, it involves examining the source code of a program to identify potential vulnerabilities, errors, or deviations from prescribed coding standards. Static code analysis offers immediate feedback as it directly operates on the source code, enabling developers to address concerns during the development phase.
But the benefits go way beyond finding style errors. Static code analysis is really about improving the quality and security of your product and your team's abilities. Using static code analysis tools, they become more aware of the vulnerabilities, errors, and deviations and become better developers.
We’ve already written everything you need to know about static code analysis. In this article, we want to concentrate on developers' tools to perform static analysis. Static code analysis tools are critical to modern software development. In an era where software plays a pivotal role in numerous industries, ensuring code reliability, security, and efficiency is paramount.
Manual reviews alone are no longer sufficient as software projects grow in complexity and size. Static code analysis tools offer an automated way to inspect code, providing developers with insights into potential issues, often well before the code runs.
What Static Code Analysis Tools Do
Static code analysis tools bring many advantages to the software development process.
Bug Detection
One of the primary utilities of static code analysis tools is their ability to pinpoint errors and bugs at the nascent stages of development. By examining the code in its static state, these tools offer an early warning system, alerting developers to potential pitfalls before they transform into complex problems.
The breadth of their rule sets ensures that the code undergoes an exhaustive check, casting a wide net to capture many potential issues. This saves troubleshooting time down the line and leads to more stable initial builds.
Code Quality
Code quality is paramount for the longevity and scalability of software projects. Static code analysis tools play a pivotal role by ensuring the codebase adheres to predefined coding standards. This automated adherence check reduces manual review time and guarantees uniformity.
Moreover, these tools elevate the quality of the code by enforcing recognized programming best practices. This translates to code that's syntactically correct and optimized for performance and readability.
Security
In an age where security breaches make headlines, ensuring software security is more crucial than ever. Static code analysis tools stand as vigilant guards in this domain. They scrutinize code for familiar vulnerabilities such as SQL injections, cross-site scripting, or buffer overflows.
By flagging these security threats in the development phase, developers can be proactive against potential exploits. Additionally, in sectors where software needs to align with strict security benchmarks, these tools ensure that the code complies, serving as an essential audit mechanism.
Maintenance
A consistent codebase is a maintainable codebase. Static code analysis tools are instrumental in fostering code consistency. They streamline the structure and quality of the code, making it more transparent and understandable for any developer working on it, be it today or in the future.
Furthermore, these tools play a significant role in curtailing technical debt by facilitating the early rectification of issues. This proactive approach means the code remains agile and less burdened by legacy issues, leading to more straightforward updates and iterations.
Types of Static Code Analysis Tools
Static analysis tools is an umbrella term for a vast array of different subtypes of tools. The most common are linters. Linters are designed to analyze source code for potential errors, bugs, stylistic issues, and violations of a particular programming convention or coding standard. The term "lint" was originally the name of a tool that checked C code, but now "linting" refers to the process in general, regardless of language.
Static code analysis is a vast field, and various tools have been developed to focus on specific aspects of code quality, maintainability, and security. Apart from linters, there are several other subtypes of static analysis tools:
- Bug finders. These tools specifically target potential bugs in the code. While there's overlap with linters, bug finders often focus on more complex issues that can lead to runtime errors or unexpected behavior. An example is SpotBugs for Java.
- Security scanners. They focus on identifying vulnerabilities in the code that might be exploited for malicious purposes. This includes checking for patterns that cause security breaches like SQL injection, buffer overflows, or cross-site scripting. Examples include Trivy, which scans across multiple languages. Secret scanners also fall under this category. These look for any API keys or secrets left in the code before pushing to remote repositories. An example is Checkov, which identifies secrets and detects AWS credentials.
- Type checkers. These tools, especially in dynamically typed languages, enforce type constraints or identify potential type-related issues before runtime. A popular example is mypy for Python.
- Complexity analyzers. They measure various complexity metrics of code, like cyclomatic complexity, which can give insights into the maintainability and potential error-proneness of the software. Tools like Radon for Python can help with this.
- Dependency checkers. They examine the dependencies your project relies on and can highlight outdated libraries, libraries with known vulnerabilities, or licensing conflicts. Trivy again can perform this role.
- Duplicate code detectors. These tools identify blocks of code duplicated across the codebase, which can indicate poor maintainability and a potential source of bugs. CPD (Copy/Paste Detector) from PMD.
It's important to understand that while each tool offers unique insights, no single tool can capture all potential issues. Typically, multiple static analysis tools are employed in a mature software development process to ensure comprehensive coverage of potential codebase problems.
This is one of the problems Codacy solves. Codacy runs a selection of these tools across your code, looking for bugs, vulnerabilities, duplicate code, complexity, and security issues to improve your code's quality significantly.
Static code analysis tools are more than just automated reviewers. They are strategic allies in the software development process, bolstering code reliability, fortifying security, and championing best practices, culminating in superior software products and optimized development operations.
Popular Static Code Analysis Tools
Every programming language has its suite of static analysis tools. Some, such as CPD or Trivy, work across multiple languages. Others, such as PHP_CodeSniffer, only operate on one language (Can you guess this one?)
You can find the entire list of static code analysis tools Codacy uses in our documentation, but here are some of the most-used languages:
- For C/C++:
- Clang-Tidy: checks for style violations, interface misuse, or C and C++ code bugs.
- Cppcheck: Detects bugs and focuses on detecting undefined behavior and dangerous coding constructs
- For Java:
- PMD: An open-source cross-language tool that scans Java code for potential problems like bugs, dead code, suboptimal code, and overcomplicated constructs.
- SpotBugs: Targets bug patterns in Java code, providing a detailed analysis to prevent potential issues.
- For Python:
- For JavaScript:
Let’s show an example of one of these tools in action: Pylint. You can install Pylint like any other Python library:
pip install pylint
We’re going to run some buggy code with quite a few errors through this tool:
#buggy-code.py
class ArithmeticOperations:
def __init__(self,x,y):
self.x=x
self.y=y
def add(self): return self.x+self.y
def subtract(self,x,y): return x-y
def multiply(self): self.x * self.y
def division(self):
if self.y == 0:
return "Can't divide by zero!"
return self.x / self.y
def modulo(self):
return x%y
def power(self, x, y): x ** y
def print_results(obj):
results = {
'Add': obj.add(),
'Subtract': obj.subtract(obj.x,obj.y),
'Multiply': obj.multiply(),
'Division': obj.division(),
'Modulo': obj.modulo(),
'Power': obj.power(obj.x, obj.y)
}
for operation, result in results.items():
print(operation + ":", result)
if __name__ == "__main__":
arithmetic_obj = ArithmeticOperations(10, 5)
print_results(arithmetic_obj)
To run, all you need to do is:
pylint buggy-code.py
Let’s see what Pylint makes of it.
************* Module buggy-code
buggy-code.py:13:0: W0311: Bad indentation. Found 6 spaces, expected 8 (bad-indentation)
buggy-code.py:14:0: W0311: Bad indentation. Found 8 spaces, expected 12 (bad-indentation)
buggy-code.py:15:0: W0311: Bad indentation. Found 6 spaces, expected 8 (bad-indentation)
buggy-code.py:17:21: C0303: Trailing whitespace (trailing-whitespace)
buggy-code.py:1:0: C0114: Missing module docstring (missing-module-docstring)
buggy-code.py:1:0: C0103: Module name "buggy-code" doesn't conform to snake_case naming style (invalid-name)
buggy-code.py:1:0: C0115: Missing class docstring (missing-class-docstring)
buggy-code.py:6:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:6:19: C0321: More than one statement on a single line (multiple-statements)
buggy-code.py:8:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:8:28: C0321: More than one statement on a single line (multiple-statements)
buggy-code.py:10:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:10:24: W0104: Statement seems to have no effect (pointless-statement)
buggy-code.py:10:24: C0321: More than one statement on a single line (multiple-statements)
buggy-code.py:12:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:17:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:18:15: E0602: Undefined variable 'x' (undefined-variable)
buggy-code.py:18:17: E0602: Undefined variable 'y' (undefined-variable)
buggy-code.py:20:4: C0116: Missing function or method docstring (missing-function-docstring)
buggy-code.py:20:27: W0104: Statement seems to have no effect (pointless-statement)
buggy-code.py:20:27: C0321: More than one statement on a single line (multiple-statements)
buggy-code.py:22:0: C0116: Missing function or method docstring (missing-function-docstring)
-----------------------------------
Your code has been rated at 0.00/10
Oh boy. 0 out of 10. Quite some code. Pylint has found styling issues (Bad indentation), documentation errors, pointless statements, and undefined variables.
This shows that static code analysis isn’t just about fixing code. It is about providing a better developer experience. The developers working on this code after you need those docstrings to understand the code better. Static code analysis tools, therefore, help you recognize not just errors in your code but also errors in your coding.
How Static Analysis Tools Work
Each of the types of tools above will work differently. However, there are general underlying mechanics for how these tools operate. They involve multiple complex algorithms and techniques.
First, before any meaningful analysis can be done, the tool parses the code. The source code must be translated into a format easier for the tool to analyze. This involves converting the code into an Abstract Syntax Tree (AST) or another intermediate representation. The AST represents the hierarchical structure of the source code (AST is also used to compile code). Each node in the tree corresponds to a construct in the source code, such as a variable declaration, an assignment, a loop, and so forth.
Once the AST is generated, the tool conducts semantic analysis to gather more context about the code. This phase is crucial for understanding variables' types, scopes, and possible values.
Then, tools will look at the flow of the code. Control Flow Graphs (CFGs) are constructed to represent the flow of the program. CFGs help in understanding the possible paths the program can take. Nodes in a CFG represent basic blocks (a straight-line sequence of code without any jumps), and the edges represent jumps (like those due to decision-making constructs).
Data Flow Analysis focuses on determining the possible values variables can take at different points in the code. For example, through data flow analysis, a tool might decide that a particular variable might be uninitialized before it's used, leading to a potential bug.
Then, the tools will use rules and patterns to detect code smells and bugs:
- Pattern matching. Many static analysis tools employ pattern matching to detect known problematic code patterns. These patterns are often based on well-documented bugs or vulnerabilities. For instance, if a tool sees a pattern where user input is directly used in an SQL statement, it could flag it as a potential SQL injection vulnerability.
- Rule-based checks. Most tools come with a predefined set of rules to check the code. These rules can be based on coding standards, best practices, or known vulnerabilities. Examples include checking if certain functions (like strcpy in C) are used, which might indicate potential buffer overflows.
- Type checking. Even in dynamically typed languages, static analysis tools can perform a form of type inference to determine the possible types of variables and ensure they are used correctly.
Once the analysis is complete, the tool compiles its findings and generates a report detailing the potential issues, their severity, and often suggestions for resolution.
The Core Features to Expect in Static Code Analysis Tools
Static code analysis tools have features designed to provide thorough code assessments and streamline the development process. While specific features can vary depending on the tool, several core functionalities are standard across many static code analysis platforms:
Rule-Based Analysis:
-
- Predefined Rules: Most tools contain built-in rules that detect common coding errors, vulnerabilities, or deviations from coding standards.
- Severity Levels: Detected issues are often categorized by severity levels, such as critical, major, minor, or informational, allowing developers to prioritize fixes.
- Predefined Rules: Most tools contain built-in rules that detect common coding errors, vulnerabilities, or deviations from coding standards.
Custom Rules:
-
- Customizability: While the predefined rules cover many scenarios, teams might have specific needs. Many tools allow users to define custom rules tailored to a project's or organization's requirements.
- Rule Management: Tools often provide interfaces or configurations for managing, enabling, or disabling specific rules based on project needs.
- Detailed Reports: Post-analysis, tools generate detailed reports outlining detected issues, their locations, and often suggested fixes or references to understand the problem.
- Visualization: Some tools provide dashboards or visualization features that offer an overview of the code's health, trends over time, or areas needing attention.
- CI/CD Pipelines: Many static code analysis tools can seamlessly integrate into Continuous Integration and Continuous Deployment (CI/CD) pipelines, ensuring code checks occur automatically during build or deployment stages.
- Version Control Systems: Integration with platforms like GitHub, GitLab, or Bitbucket allows for code analysis to be part of pull or merge request checks.
- Customizability: While the predefined rules cover many scenarios, teams might have specific needs. Many tools allow users to define custom rules tailored to a project's or organization's requirements.
That last feature, integrations, is core to how these tools are used. As we've done above, you can’t run every file through all your tools one by one. Software development teams can push hundreds of files with thousands of lines of code daily. Static tool analysis requires automation.
You do this in two ways. First, as the above states, you integrate these checks into your CI/CD pipeline. When a Pull Request is created, or code is merged, these tools run to ensure the new code doesn’t include any vulnerabilities or security issues and is up to the organization's quality standards.
Second, you can use a code quality platform like Codacy to manage all your tools. This is important because a) you will run multiple tools–security scanners, bug finders, complexity analyzers–on each PR, and b) each tool requires maintenance. These tools constantly evolve with new rules as new vulnerabilities are discovered, or new standards are set. A code quality platform like Codacy manages all this for you.
The Limitations of Static Code Analysis Tools
While static code analysis tools offer many benefits and advanced features, they have limitations and challenges. Understanding these constraints is essential to maximize their utility and address potential pitfalls.
The most common issue is false positives (or negatives). Tools might flag code segments as problematic even when they are not, leading to wasted time in verifying and addressing non-existent issues. Conversely, tools might miss actual issues, leading to a false sense of security. This second issue is particularly true if you aren’t updating your tools to the latest version incorporating the newest security threats.
A second issue is performance. Large codebases can take significant time to scan, primarily if a deep analysis is conducted. This can introduce delays in development workflows or CI/CD pipelines. Comprehensive analysis can also be resource-intensive, affecting the performance of other development tasks if run concurrently. You will pay for resource-hungry tooling if you run your CI/CD pipelines on remote clusters. Again, this can be mitigated using a platform that intelligently scans your code.
Finally, as we’ve elucidated above, integrating all these tools is complex. Some tools require intricate configurations to tailor to a project's specific needs, which can be time-consuming. Then you have rule management. Maintaining, updating, or customizing rule sets can be complex, especially for larger teams or projects with evolving requirements. A platform approach makes this more manageable.
There are some best practices with static analysis tools that you can use to help with some of these issues:
- Prioritize issues. Always prioritize resolving critical vulnerabilities and errors. These can have significant impacts on security or functionality. To address them methodically, organize issues based on severity, complexity, and impact.
- Regularly update rule sets. Software ecosystems, languages, and vulnerabilities evolve. Ensure that your rule sets are up-to-date to capture the latest issues. Also, over time, some rules may become obsolete or less relevant. Periodically review and refine rules to maintain analysis relevance.
- Manage false positives. Before committing resources to fix reported issues, validate them to ensure they are genuine. If a specific rule consistently leads to false positives, consider tweaking its configuration or disabling it temporarily.
- Establish consistent configurations. Ensure the development team uses consistent configurations to maintain uniform code quality standards. Store configuration files in version control systems to track changes and ensure synchronization across the group.
- Balance depth vs. speed. Not every scan needs to be exhaustive. Use deeper scans for comprehensive reviews and lighter scans for quick checks. Consider tools or modes that analyze only changed code sections to improve speed, especially for frequent checks.
By adhering to these best practices, teams can harness the full potential of static code analysis, ensuring improved code quality, enhanced security, and efficient development workflows.
Static Code Analysis Tools Are a Must for Software Development Teams
Static code analysis has firmly established itself as an invaluable tool in the arsenal of software development teams. Its ability to systematically scrutinize source code without execution enables developers to proactively identify and rectify potential issues, thereby enhancing software projects' overall quality, security, and maintainability.
Over the years, the capabilities of static code analysis tools have significantly expanded. Today, they detect standard coding errors and incorporate advanced features driven by innovations like machine learning to offer predictive analysis and intelligent recommendations. The seamless integration of these tools into development environments and CI/CD pipelines further streamlines the development process, ensuring consistent code quality throughout the software development lifecycle.
However, as with any tool, the efficacy of static code analysis depends on its judicious application. Organizations can maximize the benefits derived from static code analysis by understanding its limitations, integrating it thoughtfully into development processes, and adhering to established best practices.
While the landscape of software development continually evolves, the importance of code quality remains paramount. Static code analysis, with its growing capabilities and features, will continue to play a pivotal role in ensuring that code functions as intended and stands the test of time in terms of reliability and security.
Codacy is used by thousands of developers to analyze billions of lines of code every day!
Getting started is easy and free! Just use your GitHub, Bitbucket, or Google account to sign up for a free 14-day trial today.