OWASP Explained: Secure Coding Best Practices
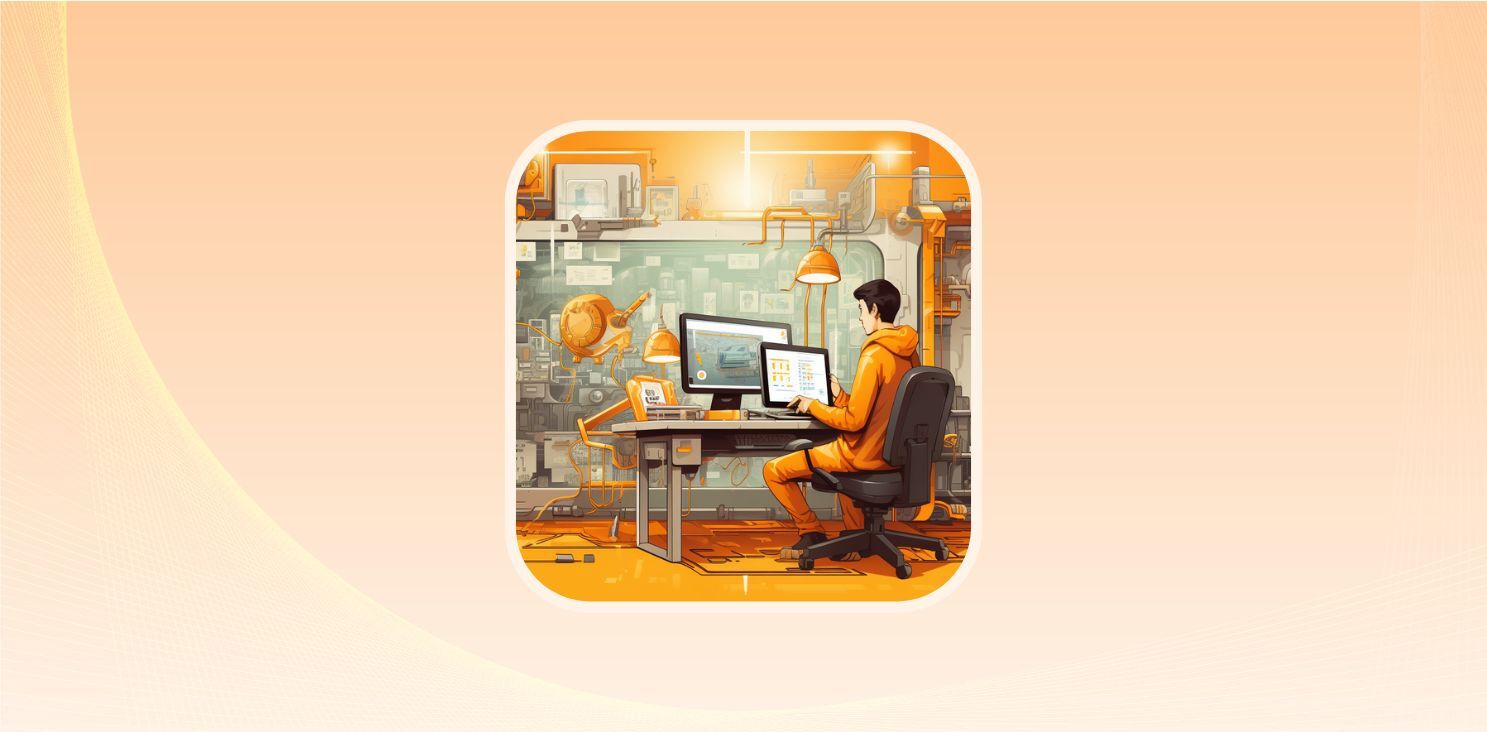
From global finance to daily communication, software underpins every aspect of modern life. That's why a single data breach can shatter user trust, cripple businesses, and even disrupt world peace. Adopting security standards is essential to prevent and mitigate these risks. These frameworks help structure and systemize how you build secure software.
One of the most popular and influential communities on software security standards is the Open Worldwide Application Security Project (OWASP), a global network of experts and practitioners who share their insights and tips on building secure software. Following their recommendations and guidance can help avoid common pitfalls and security vulnerabilities plaguing software products.
In this article, we will explain what the OWASP is, its significance in the software industry, its most popular OWASP Top 10 publication, and how to implement secure coding practices using their recommendations. We also tapped into our network of experts in the field who share their 2 cents on OWASP.
What Is OWASP?
OWASP is a nonprofit organization that provides resources and guidance for developers and security experts to build, test, and maintain secure software applications.
OWASP was founded in 2001 by Mark Curphey, a software security consultant, who saw the need for a collaborative project to document and share web application security knowledge and best practices.
Since 2001, OWASP has grown into a global community of thousands of volunteers contributing to various software security projects and research. These include the popular OWASP Top 10, a list of the most critical web application security risks, and the OWASP Application Security Verification Standard (ASVS) Project, which provides a basis for testing web application security controls and also provides developers with a list of requirements for secure development.
OWASP helps developers, security professionals, and organizations understand potential software threats and adopt security best practices. Note: A big misconception is that security slows teams down—but when done right, it actually streamlines development.
"Integrating OWASP's secure coding practices into development workflows doesn't have to slow teams down—it's all about making security a seamless part of the process. Embedding security checks into CI/CD pipelines, using automated scanning tools, and providing developers with hands-on security training can help catch vulnerabilities early without disrupting productivity." – Stanislav Khilobochenko, VP of Customer Services, Clario.
How to Use OWASP Top 10 for Effective Application Security
The OWASP Top 10 has various uses that are foundational to application security:
- As a training aid on the most common web application vulnerabilities.
- As a starting point when testing web applications.
- To raise awareness of vulnerabilities in applications in general.
- As a set of demonstration topics to guide discussions on security risks.
The OWASP Top 10 provides a structured overview of the most critical web application vulnerabilities, offering both a roadmap and a set of actionable guidelines to help organizations strengthen their application security.
Whether you're a developer, security professional, or part of a broader organization, leveraging the OWASP Top 10 will ensure your web applications are better protected against common and emerging threats.
Here’s how you can effectively use the OWASP Top 10 to enhance your security practices:
Conduct a Risk Assessment
Once you’ve understood the risks, perform a comprehensive risk assessment to identify which OWASP Top 10 vulnerabilities are most relevant to your applications. Consider factors such as:
- Application Architecture: Are there any areas of your application that are susceptible to these risks? For example, an application relying on user inputs may be vulnerable to injection attacks (A1: Injection).
- Data Sensitivity: Does your application handle sensitive data, making it a target for exposure (A3: Sensitive Data Exposure)?
- Authentication Mechanisms: Are there weak authentication processes that could lead to broken authentication vulnerabilities (A2: Broken Authentication)?
Mapping your application’s architecture and data flow against the OWASP Top 10 helps you identify the most critical security gaps that need immediate attention.
Integrate OWASP Top 10 into Development
One of the most effective ways to use the OWASP Top 10 is by integrating it into your software development lifecycle (SDLC). Implement security best practices for each vulnerability category, such as:
- Secure coding practices to prevent injection attacks.
- Encryption protocols to ensure data is properly protected during storage and transmission.
- Two-factor authentication for user access to enhance authentication security.
Embedding security measures related to the OWASP Top 10 during the design and development stages is a critical step to securing applications from the ground up.
Many teams struggle with finding the right moment to introduce security practices without slowing down development. The best way to avoid last-minute security chaos is to shift left—catch issues as early as possible.
"I've been deeply involved in the authentication and security space for years, leading FusionAuth, a company focused on delivering secure, developer-friendly solutions for customer identity management. One effective method for integrating OWASP's secure coding practices without causing disruption is to start with a 'Shift Left' approach by embedding security concerns earlier in the development cycle. At FusionAuth, we implement DevSecOps, which allows us to perform security checks every time code is accepted, reducing last-minute security surprises."— Brian Pontarelli, CEO, FusionAuth.
Use Automated Security Tools
Integrate automated security tools into your CI/CD pipeline to continuously scan for vulnerabilities from the OWASP Top 10.
Tools like Static Application Security Testing (SAST), Dynamic Application Security Testing (DAST), and Interactive Application Security Testing (IAST) can help detect vulnerabilities as code is written and deployed.
These tools can identify vulnerabilities like SQL injection (A1) or cross-site scripting (A7) before they make it to production. Regular automated scans also help catch issues that might be overlooked during manual code reviews.
Manual security checks can be time-consuming, inconsistent, and prone to human error. Teams can automatically detect vulnerabilities before they become major issues by integrating security tools directly into CI/CD pipelines.
"Integrating security tools like OWASP ZAP directly into our Jenkins pipeline helped catch 90% of common vulnerabilities before they hit production. In our early days at Local Data Exchange, we struggled with false positives, but training our CI/CD pipeline to recognize our specific use cases and maintaining a whitelist of approved patterns really helped streamline our security checks." — Joshua Odmark, CIO and Founder, Local Data Exchange.
Perform Regular Security Testing
Don’t just rely on initial security tests. Continuous testing is key to maintaining application security. Schedule regular security assessments, including:
- Penetration Testing: Simulate real-world attacks to uncover weaknesses in your applications that automated tools might miss.
- Vulnerability Scanning: Use automated tools to continuously check for OWASP Top 10 vulnerabilities, especially after each code update or release.
- Security Audits: Conduct periodic audits to ensure your security measures are in line with the latest OWASP guidelines.
By consistently testing your application against OWASP Top 10 risks, you’ll be able to stay ahead of emerging vulnerabilities and patch them.
One expert we spoke to recommended proactive testing strategies, such as real-time code analysis and security workshops:
"Instead of pulling developers into lengthy, generic security seminars, practical code reviews and hands-on workshops tied to actual project code can be more impactful. Development teams should also utilize pre-commit hooks and IDE plug-ins that provide immediate feedback on security issues as they code, making it easier to correct vulnerabilities without derailing productivity." — Patric Edwards, Founder & Principal Software Architect, Cirrus Bridge.
Educate Developers on Secure Coding
Training your development team on the OWASP Top 10 is essential for reducing vulnerabilities in the first place. Developers need to understand the common security pitfalls associated with each risk:
- How to prevent SQL injection (A1) through input validation and parameterized queries.
- Best practices for securing authentication mechanisms (A2) like password hashing and multi-factor authentication.
- The importance of data encryption (A3) both at rest and in transit.
Educating your developers on secure coding practices will help minimize the chances of them introducing OWASP Top 10 vulnerabilities in the codebase.
Security awareness isn't a one-time event—it has to be built into the culture of development teams. Instead of long, infrequent security training, teams benefit more from continuous, bite-sized learning sessions.
"When integrating OWASP's secure coding practices, it's important to educate the team on why each practice matters. I've seen teams run weekly short training sessions, internal or outsourced, making secure coding a standard part of agile ceremonies." — Kevin Gallagher, Owner, wpONcall.
Track and Prioritize Vulnerabilities
Not all vulnerabilities are created equal. Some might have more severe consequences, while others might be less critical. Use a risk-based approach to prioritize the remediation of vulnerabilities based on:
- Impact: How severe would the exploitation of this vulnerability be for your application or users?
- Exploitability: How easy is it for an attacker to exploit this vulnerability?
- Exposure: How exposed is the vulnerable part of your application to potential attackers?
Categorizing and prioritizing vulnerabilities helps ensure that the most critical risks are addressed first, effectively mitigating the highest-impact threats.
Without automated monitoring, teams risk spending time on minor vulnerabilities while missing critical threats.
"We achieved this at Celestial Digital Services by using AI-driven tools for real-time error detection and proactive responses. By integrating these practices with routine code reviews and performance tracking, teams stay ahead in mitigating vulnerabilities without workflow disruption." — Rodney Moreland, Founder, Celestial Digital Services.
Create a Remediation Plan
For each vulnerability identified through the OWASP Top 10, develop a detailed remediation plan that includes:
- Immediate fixes: Quick patches or workarounds for vulnerabilities that can be easily addressed.
- Long-term solutions: Architectural or process changes are required to eliminate certain vulnerabilities in the future.
- Testing and validation: Steps to validate that vulnerabilities have been fixed and no new issues have been introduced.
A clear and structured remediation plan helps ensure vulnerabilities are fixed efficiently and thoroughly.
Stay Updated
The OWASP Top 10 is updated periodically to reflect the latest trends in web application security. Stay informed about changes to the OWASP list, and make sure your security strategy adapts accordingly.
Security threats evolve, so maintaining a proactive security posture requires constant vigilance. Subscribe to OWASP updates, attend security conferences, and engage with the security community to stay up-to-date on the latest risks and mitigation strategies.
The OWASP Top 10 Software Security Vulnerabilities
The OWASP Top 10 is a standard awareness document on software application security for developers and engineers. The latest version, released in 2021, represents a broad consensus about the most critical security risks facing software applications obtained from community research.
OWASP Top 10 Risk (2021) |
Description |
Common Vulnerabilities |
Impact |
Prevention Best Practices |
A01:2021-Broken Access Control |
Improper enforcement of user roles and permissions allows attackers to access restricted resources. |
Privilege escalation, unauthorized access |
Data breaches, system compromise |
Implement least privilege access, enforce strong access controls, use role-based access control (RBAC). |
A02:2021-Cryptographic Failures |
Weaknesses in cryptographic systems, often leading to sensitive data exposure or system compromise. |
Weak encryption, poor key management, insecure algorithms |
Data exposure, data theft, system compromise |
Implement least privilege access, enforce strong access controls, use role-based access control (RBAC). |
A03:2021-Injection |
Malicious input leads to unintended code execution, compromising the system or application. |
SQL injection, command injection, Cross-Site Scripting (XSS) |
Data loss, unauthorized access, remote code execution |
Use parameterized queries, validate and sanitize all user inputs. |
A04:2021-Insecure Design |
Flaws in application design increase security risks, often leaving vulnerabilities open for exploitation. |
Insecure APIs, weak security patterns, lack of threat modeling |
Exploitation of design flaws, system compromise |
Use threat modeling, secure design patterns, and reference architectures. |
A05:2021-Security Misconfiguration |
Incorrect or incomplete configurations that leave systems vulnerable to attacks. |
Default configurations, open ports, unnecessary services |
Unauthorized access, data exposure, exploitation |
Regularly update configurations, disable unnecessary services, implement automated configuration management. |
A06:2021-Vulnerable and Outdated Components |
Outdated or vulnerable libraries, frameworks, and components increase the risk of exploitation. |
Unpatched libraries, old software versions, known CVEs |
Data breaches, malware infections, system crashes |
Regularly update and patch components, use Software Composition Analysis (SCA) tools to identify vulnerabilities. |
A07:2021-Identification and Authentication Failures |
Weak or improper authentication mechanisms allow attackers to impersonate legitimate users. |
Weak password policies, session hijacking, credential stuffing |
Account takeover, unauthorized access |
Implement multi-factor authentication, enforce strong password policies, secure session management. |
A08:2021-Software and Data Integrity Failures |
Assumptions about the integrity of software and data, such as unverified updates or CI/CD pipelines, lead to security risks. |
Malicious code injections, unverified software updates |
Malware infections, unauthorized access, data manipulation |
Verify the integrity of software and updates, use signed software packages, ensure secure CI/CD pipelines. |
A09:2021-Security Logging and Monitoring Failures |
Insufficient logging or lack of monitoring makes it difficult to detect and respond to security incidents. |
Missing logs, inadequate log aggregation, absence of real-time alerts |
Delayed response, inability to detect attacks |
Implement centralized logging, enable real-time monitoring, review logs regularly for suspicious activity. |
A10:2021-Server-Side Request Forgery (SSRF) |
An attacker can manipulate a server to make requests on its behalf, potentially exposing internal services. |
SSRF, URL manipulation, unauthorized internal access |
Internal system compromise, information leakage |
Validate and sanitize input URLs, restrict outgoing requests, implement proper access controls on internal services. |
A01:2021-Broken Access Control
Broken access control is a security risk when web applications fail to properly enforce authorization checks on users' actions and requests. Access control “breaks” when attackers can successfully request access to something they shouldn't have access to.
Broken access control allows unauthorized users to access, modify, or delete sensitive data or resources, perform administrative functions, or take over other users' accounts.
Some of the best protection strategies for preventing broken access control are:
- Grant users the minimum access level required for their tasks. Don't provide administrative access by default.
- Implement role-based access control (RBAC) to assign granular permissions based on user roles and responsibilities.
- Validate and sanitize all user inputs on the server before making access control decisions.
- Use random or encrypted identifiers for resources, such as user profiles, documents, or transactions.
Consider a scenario where users can add and edit comments on a blog post. You want to make sure that they can only edit comments that belong to them:
class User:
def __init__(self, username, role):
self.username = username
self.role = role
class Comment:
def __init__(self, content, author):
self.content = content
self.author = author
class BlogPost:
def __init__(self, title, author):
self.title = title
self.author = author
self.comments = []
def add_comment(self, comment):
self.comments.append(comment)
def can_edit_comment(self, user, comment):
if(user.username == comment.author.username) # User can edit their own comments
return True
else:
return False
Implementing real-world RBAC can be more complex, but you can find robust libraries that are well-maintained and appropriate for your web application's framework.
A02:2021-Cryptographic Failures
A cryptographic failure occurs when a system makes sensitive data accessible to potentially malicious attackers. Cryptographic failures usually result from the improper use or implementation of cryptographic techniques, such as encryption, hashing, signing, or secrets management, in software applications.
The best protection strategy for cryptographic failures is to use cryptography correctly and securely throughout the application. This means:
- Use secure and reliable cryptographic libraries or services that are well-tested, well-reviewed, and well-maintained, like PyCryptodome, Cryptography (Python), JSEncrypt, Forge (JavaScript), Bouncy Castle, and Web3j (Java). Avoid writing your own cryptographic code.
- Store keys securely using hardware security modules (HSMs) or dedicated key management systems.
- Enforce HTTPS for all communication, especially when transmitting sensitive data.
- Regularly rotate keys to minimize the impact of potential compromises. Implement automated key rotation processes where feasible.
A03:2021-Injection
Injection happens when an attacker sends invalid data into an application to make it do something it was not supposed to do. SQL injection is a popular code injection technique. It happens when an attacker inserts malicious SQL code into user input that gets processed by the application and executed on the database.
Some of the best protection strategies for preventing SQL injection are:
- Validate and sanitize all user inputs before sending them to a code interpreter. Use parameterized queries or prepared statements, which separate the query or command structure from the user input.
For example, in a scenario where users can add comments to a blog post, you want to ensure they do not inject malicious SQL statements, which could be harmful to the database. Here, you can make use of parameterized queries using (?) and then supply the values using a safe method:
This way, the user input will not affect the structure of the SQL statement and will prevent SQL injection attacks.
def add_comment(user_id, content):
try:
conn = sqlite3.connect("mydb.sqlite")
cursor = conn.cursor()
# Use a parameterized query
query = "INSERT INTO comments (user_id, content) VALUES (?, ?)"
cursor.execute(query, (user_id, content))
conn.commit()
print(f"Comment added successfully for user '{user_id}'!")
except sqlite3.Error as e:
print(f"Error: {e}")
finally:
conn.close()
# Example usage
if __name__ == "__main__":
user_id = "alice@example.com"
comment_content = "Great article! I enjoyed reading it."
add_comment(user_id, comment_content)
- Regularly update your database software, application frameworks, and libraries to benefit from security patches.
- Limit the database access based on individual user roles and functions.
A04:2021-Insecure Design
Insecure design is a security weakness that stems from the lack of security considerations or principles in the design phase of web applications. Insecure design can introduce flaws or vulnerabilities that are hard or impossible to fix in the later stages of development or deployment.
Preventing insecure design requires a proactive approach throughout the development lifecycle. Here are some key strategies:
- Integrate security considerations from the very beginning, aligning them with functional requirements.
- Utilize static code analysis tools such as Codacy to identify potential security vulnerabilities in your codebase early on.
- Use well-proven and secure design patterns like Defense in Depth, Least Privilege, and Secure Direct Object References.
- Conduct DAST to uncover runtime vulnerabilities and weaknesses in your application's behavior.
- Automate deployment processes to minimize human error
A05:2021-Security Misconfiguration
Security misconfiguration is a security weakness that occurs when an application or its components are not configured correctly or securely, leaving them vulnerable to attacks.
Plain and simple, any technical issue across any component that may arise from the app's configuration settings and not bad code is classified as a security misconfiguration vulnerability.
As a developer, you play a critical role in preventing them by implementing these key strategies:
- Limit access to configuration files and interfaces to authorized personnel only.
- Utilize tools like Chef, Puppet, or Ansible to automate configuration deployment and management across environments.
- Regularly review and update cloud service configurations to adhere to security best practices.
- Document configuration settings clearly and maintain detailed records.
A06:2021-Vulnerable and Outdated Components
Vulnerable and outdated components are security weaknesses resulting from using web application components, such as libraries, modules, plugins, or APIs, that have known vulnerabilities or are no longer supported or maintained.
To prevent attacks from vulnerable and outdated components, follow these tips:
- Employ tools like Maven, npm, or NuGet to automatically track and manage dependencies, simplifying vulnerability identification and updates.
- Obtain components from official sources or trusted repositories to minimize the risk of malware or malicious code.
- Create a comprehensive list of all software components used in your application, including their versions, dependencies, and origin sources. This provides a clear picture of your attack surface.
A07:2021-Identification and Authentication Failures
Identification and authentication failures are security weaknesses that occur when an application does not properly verify or validate the identity or credentials of users or entities. Broken authentication allows hackers to bypass authentication controls, steal someone's identity to log in, or break other security mechanisms.
Here are some key strategies to prevent these failures:
- Utilize rate limiting on login attempts to thwart automated attacks. Consider CAPTCHAs or risk-based authentication for suspicious activity.
- Enforce strong password policies, including minimum length, complexity, and regular rotation. Encourage multi-factor authentication (MFA) for added security.
- Secure APIs with strong authentication and authorization mechanisms like OAuth or API keys.
- Monitor login attempts and system activity for suspicious behavior. Logs can help identify and respond to potential attacks.
A08:2021-Software and Data Integrity Failures
Software and data integrity failures relate to code and infrastructure that do not correctly protect or verify the integrity or authenticity of software or data. This vulnerability can lead to severe consequences, including data corruption, loss of customer trust, and regulatory non-compliance.
Preventing software and data integrity failures is crucial for maintaining the reliability and trustworthiness of systems and information. Here are some strategies to mitigate the risk of such failures:
- Enforce strict access controls to limit access to sensitive data and critical system components.
- Encrypt sensitive data stored on disks or databases to prevent unauthorized access and tampering.
- Establish a comprehensive backup and recovery plan to ensure data availability and integrity in case of incidents.
A09:2021-Security Logging and Monitoring Failures
Security logging and monitoring failures cover the lack of proper logging and monitoring mechanisms in web applications, which can prevent or delay the detection and response to security incidents. Failing to log crucial events like login attempts, access control changes, and server errors leaves you blind to potential attacks.
Here are some tips to prevent security logging and monitoring failures:
- Capture essential activities like logins, failed attempts, file changes, database queries, and API calls.
- Leverage centralized log management systems such as Elasticsearch and Splunk for efficient analysis, storage, and search capabilities.
- Utilize tools to analyze logs for suspicious activity based on user behavior, access patterns, and known attack signatures.
A10:2021-Server-Side Request Forgery
Server-side request forgery (SSRF) is a web security vulnerability that allows an attacker to cause the server-side application to make requests to an unintended location. In a typical SSRF attack:
- An attacker sends a malicious request containing a URL or an instruction for the server to a vulnerable application.
- The server trusts the request and tries to access the specified resource, which could be internal or external.
- The attacker gains unauthorized access to the resource and can steal, modify, or compromise data or systems.
SSRF attacks are dangerous because they are used to bypass security controls and access resources that the attacker shouldn't be able to reach. This can lead to security incidents such as data breaches and service disruptions.
To prevent SSRF, developers should:
- Validate and sanitize user input before constructing or executing server-side requests.
- Maintain a whitelist of allowed URLs or domains the application can access, preventing requests to unauthorized or internal resources.
- Monitor your application's outbound traffic for suspicious requests, looking for unusual destinations, protocols, or data patterns.
The code snippet below demonstrates a vulnerability to a full SSRF attack. It directly constructs a URL using untrusted input from an HTTP request parameter.
By setting the target value to “bad.com#,” the resulting URL becomes “https://bad.com#.example.com/data/.” The snippet also provides a solution by allowing the user to select a known fixed string instead.
import requests
from flask import Flask, request
app = Flask(__name__)
@app.route("/full_ssrf")
def full_ssrf():
target = request.args["target"]
# Vulnerable: User input directly constructs the URL
resp = requests.get("https://" + target + ".example.com/data/")
# Secure: Use a fixed string based on user input
subdomain = "europe" if target == "EU" else "world"
resp = requests.get("https://" + subdomain + ".example.com/data/")
How Codacy Can Help Prevent OWASP Top 10 Vulnerabilities
Codacy can help prevent OWASP Top 10 vulnerabilities by providing automated code analysis, quality, and security tools that scan over 40 programming languages, such as JavaScript, Python, Java, C#, and PHP. Codacy can help developers:
- Identify and fix common security risks, such as injection, broken access control, or cryptographic failures, using static application security testing (SAST) and software composition analysis (SCA) techniques.
- Monitor and improve security performance and compliance using code coverage, code quality, and security dashboards and reports.
- Integrate security into the development and deployment lifecycle using Codacy's integrations with GitHub, GitLab, Bitbucket, Jenkins, and other tools.
Using Codacy, you can reduce the time and effort required to prevent OWASP Top 10 vulnerabilities and focus on delivering secure and robust web applications.
Learn more about how Codacy can help you prevent OWASP's Top 10 vulnerabilities by signing up for a free trial.
OWASP FAQs
1. What are the OWASP Top 10 vulnerabilities?
The OWASP Top 10 identifies the most critical security risks in web applications, such as injection attacks, broken authentication, and security misconfigurations. Organizations use it as a baseline to improve security and reduce risk.
2. What are OWASP guidelines for secure coding?
OWASP provides structured best practices for secure coding, focusing on input validation, authentication, encryption, and secure API handling. The key is integrating security early, rather than fixing issues later.
"The best way to integrate OWASP guidelines is to embed security best practices into the development pipeline early. Organizations that enforce secure coding standards at every stage of development see far fewer security breaches." – Rengie Wisper, CMO, G-BRIS.
3. How can organizations integrate OWASP security practices without slowing down development?
Many teams worry that security will slow them down, but the opposite is true when it’s built into development workflows. A phased approach helps—starting with input validation, access controls, and automated security checks.
"A phased approach to secure coding is the key. Start small—validate inputs, introduce authentication safeguards, and gradually expand. Secure development doesn't have to be disruptive when done incrementally." – Philip Stoelman, Founder & CEO, Network Republic.
4. Why is security training important for developers?
Security isn’t just a checklist—it’s a mindset. Developers need to recognize vulnerabilities before they become real threats. Ongoing training keeps teams updated on new attack methods and best practices.
"Training shouldn't be an annual checkbox exercise. Continuous, scenario-based training integrated into daily workflows is what truly makes a difference. Developers need real-world examples to understand how attackers think." – Paul Eidner, COO, InboxAlly.
5. How can CI/CD pipelines be configured to automatically detect vulnerabilities?
Automated security catches issues early. A good CI/CD pipeline should include static code analysis (SAST), runtime testing (DAST), and dependency scanning. These tools flag vulnerabilities before code is deployed.
"Security testing should be a continuous, automated process. CI/CD pipelines should include static analysis (SAST), dynamic analysis (DAST), and dependency scanning at multiple stages to detect security flaws before deployment."– Shane McEvoy, MD, Flycast Media.
6. What are the biggest challenges organizations face when implementing secure coding practices?
The biggest challenge is adoption. Many teams see security as extra work. The key to overcoming this is making security effortless by using automation, embedding security champions, and simplifying workflows.
"Security adoption struggles often stem from resistance to change. The key is making security effortless—embedding security champions into teams, using automated security tools, and tying security improvements to real business outcomes." – Chris Roy, Product & Marketing Director, Reclaim247.