The Ultimate Code Review Checklist For Developers
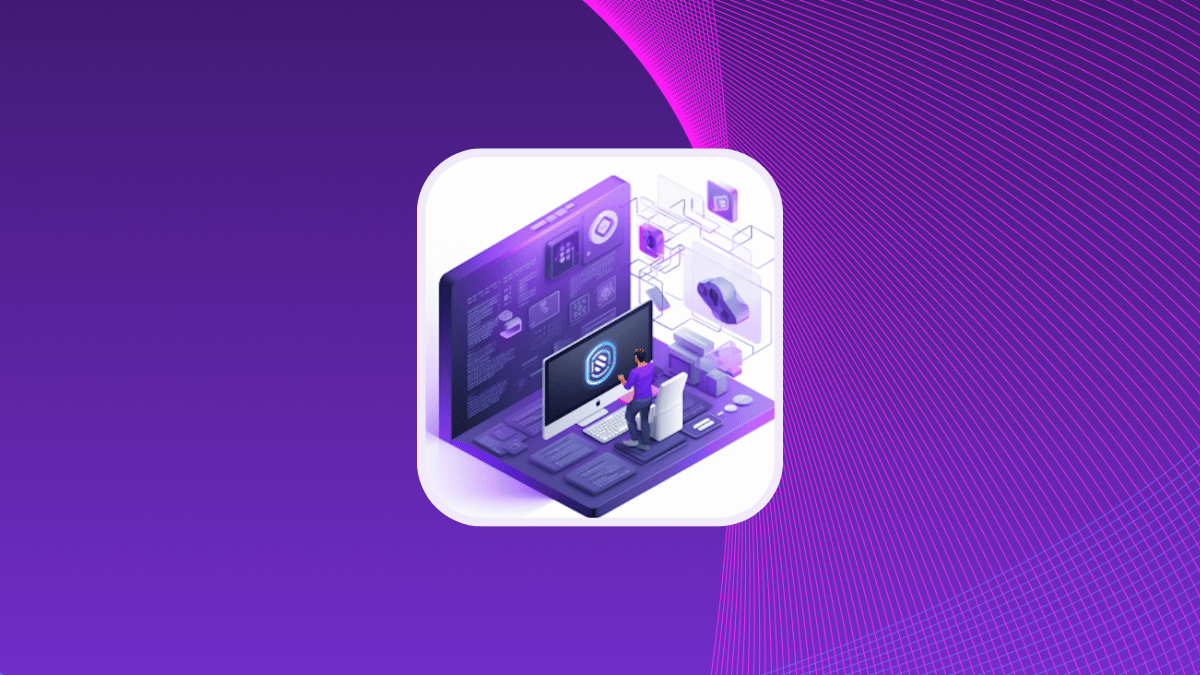
Deploying code without reviewing it first is like drinking from a dirty stream and hoping to stay healthy. Sooner or later, you'll have to deal with the consequences of not verifying what's entering your body, or in the context of software, your production environment.
Code reviews form the bedrock of the “clean code” philosophy. Every healthy codebase is a product of a stringent code review process. By conducting code reviews, software developers and teams can keep an eye on the main branch and ensure consistency among everyone involved in the code creation process.
One way to improve the effectiveness of code reviews is by using a checklist to guide reviewers on what to look for during the process. If your team wants to optimize its code review processes, then understanding some best practices and how to implement them with an application security testing (AST) solution like Codacy is pivotal.
Why Code Reviews Are Essential
No software developer is immune to mistakes — not even developers with years or decades of experience. Anyone can mistakenly access the wrong variable or fail to validate the user input, which can inadvertently lead to costly software defects in production.
Code reviews allow software teams to find defects the original author might have missed without exposing the code to customers. Here are some reasons why code reviews are essential:
- Identifies issues on time: Code reviews are often blocking in nature, meaning the new code must be reviewed and approved before being released to customers (i.e., the pull request approach). This allows code reviewers to detect and fix issues in code before they snowball out of control and wreak havoc in production.
- Allows teams to create and maintain consistency in coding style: In a team of developers, each developer might follow a different set of conventions and practices when writing code. Code reviews allow teams to define and enforce coding standards, which ensures consistency in coding style and a smoother collaboration process. This is even more important in large teams and projects.
- Enables teams to optimize code for performance: Sometimes the code accomplishes its intended task, but does so inefficiently. In such cases, code reviews allow the more experienced developers to optimize the code while offering the less experienced ones a chance to learn and improve their skills.
- Supports knowledge transfer: The collaborative nature of code reviews facilitates knowledge transfer by allowing less experienced developers to learn more reliable coding techniques and best practices from their more experienced counterparts.
Code reviews help teams maintain consistency in the codebase by enforcing policies and norms. This makes it easier to share code and allows anyone to work on any part of the code without much friction.
The Ultimate Code Review Checklist
A comprehensive code review involves examining various aspects of your software, not just the correctness of the code. Here’s a checklist of things to check for when reviewing source code.
1. Check The Code’s Logic
One of the main objectives of code reviews is to verify that the code does what it was written to do. Check that the code behaves as expected when given different parameters and that all edge cases are covered.
For example, consider this JavaScript function that calculates the sum of all even numbers in an array:
function sumEvenNumbers(arr) { |
To check if this function works correctly, we test it with different inputs, including edge cases like empty arrays, arrays with only odd or even numbers, and arrays with negative numbers.
// Test case 1: Normal case with mixed even and odd numbers |
The results are consistent with our expectations, which you should aim for in tests like these. Incorporating log statements and error handling will help troubleshoot issues if they arise.
2. Error Handling And Logging
Anyone writing code must be prepared for potential errors, whether they anticipate them or not. During review, check that the code includes proper error handling and logging mechanisms, as these help when debugging and troubleshooting.
Going back to our first example, let’s modify the sumEvenNumber function to include error logging with error messages that are clear, descriptive, and actionable:
function sumEvenNumbers(arr) { |
Based on the code above, here’s what’s going to happen in different scenarios:
- Non-array input: The function throws an error if the input is not an array.
- Non-numeric elements: If any element in the array is not a number, the function throws an error.
- Empty array: Although not an error, it's important to ensure that the function handles empty arrays gracefully (it will return 0 in this case, which is correct).
3. Readability And Maintainability
Clean code is easier to understand and maintain. During reviews, check that the code is well-formatted and follows “clean code” principles like proper indentation, consistent naming style, and appropriate use of comments to explain parts of the code that may not be obvious.
For instance, take a look at the code below:
function sumEvenNumbers(arr) { |
While this function does its job of summing all even numbers, it’s verbose and hard to read. Here’s a better version:
function sumEvenNumbers(arr) { |
We reduced the code from nine to just four lines by incorporating modern language constructs like filter() and reduce(), making the code easier to read and less verbose.
4. Code Structure and Design
Check that the new code aligns with the project’s overall architecture and design. This ensures that it integrates smoothly into the codebase and that the project’s structure remains consistent.
Here are some best practices for organizing software code:
- Dependency Inversion (DIP): This principle states that a given class should not depend directly on another class but on an abstraction (interface) of this class.
- Separation of Concerns (SoC): Organize the application into distinct layers (e.g., UI, business logic, data) so logic and presentation stay separate.
- Single Responsibility Principle (SRP): Each module/class should do one thing and do it well.
- Modularity: Organize code into small, self-contained modules to increase reusability; group related files logically (e.g., by feature, not file type).
- Open/Closed Principle (OCP): Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
5. Review For Security Vulnerabilities
Vulnerabilities often result from poor coding practices, insufficient security measures, human error, and insecure third-party libraries. For instance, this Node.js server code has several exploitable vulnerabilities:
const express = require('express'); |
Manually checking for vulnerabilities in your code can be tedious, except if your application is small and comprises a few lines of code. Security testing is best done automatically using a code review tool like Codacy, which looks for vulnerabilities in your code repositories, pull requests, and even IDE as you write code (via IDE extensions).
6. Performance
Code reviews should check not just if the code works, but also how well it performs. A piece of code might complete its task, but if the code is inefficient, it can cause unnecessary complexity, waste resources, and run slowly.
For instance, the code below is poorly optimized because it reads files synchronously inside a loop using fs.readFileSync(), which blocks the pro gram execution until it completes reading a file:
const fs = require('fs'); |
A more efficient approach would be to read the files asynchronously, so it doesn't block the execution of the program:
const fs = require('fs').promises; |
You can resolve quality issues like this by incorporating an application security testing (AST) tool that also checks for code quality, like Codacy.
7. Test Coverage
Test coverage shows if our test cases cover the application code and measures the extent to which the code is exercised during test execution. This technique is essential for uncovering potential bugs and vulnerabilities.
During code reviews, ensure that the code has undergone rigorous testing, including unit tests, integration tests, code coverage, cross-browser tests, and responsiveness tests. The tests should be passing and up-to-date.
8. Proper Use Of Dependencies
When you integrate insecure third-party code into your software, its vulnerabilities become a part of your application and can serve as an attack vector for cybercriminals. For this reason, you must review all libraries, frameworks, or components and ensure that any dependencies are managed correctly and up-to-date.
Software composition analysis (SCA) is an important security measure that scans the entire software supply chain to find and fix vulnerabilities. You can automate SCA testing using a code review tool like Codacy.
9. Adherence To Coding Standards
Coding standards are collections of coding rules, guidelines, and best practices to ensure consistent code quality, compliance with language standards, and software security.
Always check that the new code follows the standards for the organization, project, or programming language. Use linters and other static analysis tools to identify issues with code style, including formatting and syntax problems, such as variable names, use of brackets and quotation marks, tabs versus spaces, etc.
10. Documentation
Check that the code is well-documented, and that comments are included where necessary (to explain parts of the code that may not be obvious). Typically, your comments should be brief. However, thorough comments can be occasionally used to explain complex functionality.
For example:
/** |
Conclusion
The primary goal of using a code review checklist is to ensure consistency among team members who are involved in the code creation and review process. A good checklist provides newcomers and experienced developers with clear guidance on the factors to consider during the process.
Codacy specializes in automating and standardizing code reviews to assist developers in handling vast amounts of code. It provides technological tools for measuring, managing, and improving software quality and security measures, alongside integrations that streamline the code review process.
Get started with Codacy today for free and take your first step toward writing clean and secure code.