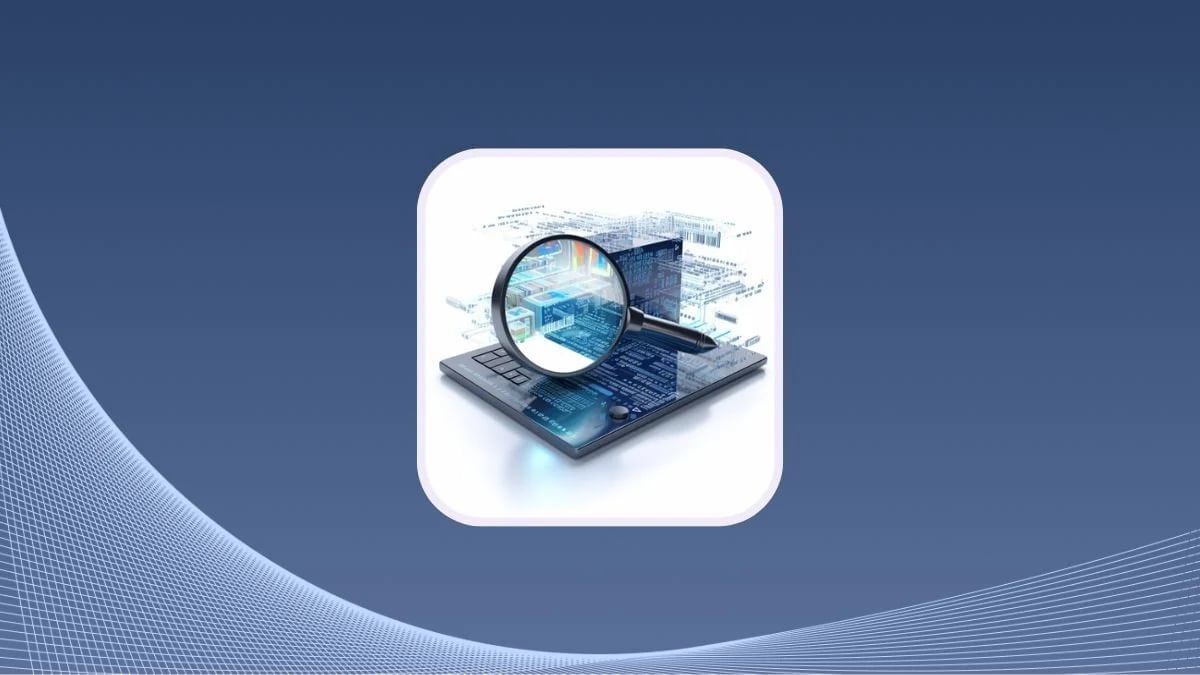
Just as financial debt can cripple individuals and organizations, technical debt—the accumulation of suboptimal software development practices—can hinder growth and innovation at your company. According to McKinsey, CIOs estimated that 10-20% of the technology budget intended for new products gets redirected toward resolving issues associated with technical debt.
However, challenges exist In aligning code quality initiatives with business objectives. According to our 2024 State of Software Quality report, 42% of development teams say illustrating how investment in code quality aligns with overarching business goals is a primary challenge in getting buy-in from upper management.
Understanding technical debt helps developers and engineering leaders improve code quality and system reliability by balancing feature development and maintenance.
We chatted with experts to better explain technical debt, the types of technical debt that exist, how technical debt is accrued, and how to reduce and manage technical debt in your organization.
What Is Technical Debt?
Technical debt is the accumulated future cost of suboptimal design decisions, outdated code, and incomplete features in a software project. It is the outcome of choices made during software development that favor speed and efficiency over long-term maintainability, security, and flexibility.
Technical debt can appear in various forms, such as poorly written code, outdated libraries, lack of proper documentation, or nonexistent test coverage. Like financial debt, technical debt accrues interest over time and can have serious consequences, such as exposing the product to security risks, increasing development costs, and lowering the team’s morale.
However, quantifying technical debt can be challenging. But it is extremely vital to align with non-technical stakeholders.
“At ETTE, we've used data analytics to translate technical debt into business impacts, showcasing potential costs of not addressing issues like outdated software that can lead to security vulnerabilities. Demonstrating this through clear metrics helps stakeholders see the tangible effects on operational efficiency and risk.”- Lawrence Guyot, President, ETTE.
The 4 Types of Technical Debt
Software development expert Martin Fowler proposed a framework to categorize technical debt into four distinct quadrants based on the intentionality and awareness of the decision that led to the debt.
This framework empowers organizations to effectively assess and manage technical debt by sorting it into high-risk and low-risk categories. While the impact of technical debt varies, some kinds of tech debt are worse than others, and tech debt can even be beneficial if managed wisely.
1. Deliberate and Reckless
This technical debt arises from intentional decisions made with full awareness of the potential consequences. Developers or teams deliberately ignore good design practices or coding standards to ship products faster. This debt is often associated with tight deadlines, pressure to release features quickly, or a lack of planning and resources.
For example, developers may skip writing unit tests, documentation, or comments, or hard code sensitive connection values in the code to save time or meet deadlines.
# Using hard-coded values for postgres database connection
import psycopg2
def connect_to_database():
connection = psycopg2.connect(
host="localhost",
database="mydb",
user="postgres",
password="password"
)
return connection
In this example, the database connection credentials are directly embedded within the code. This is a security risk, as anyone with access to the code could potentially use these credentials to access the database. A more secure approach would be to store the connection credentials in a configuration file or environment variable and then read them from there when connecting to the database.
This type of technical debt is hazardous, as it can lead to high complexity, low cohesion, and high defect rates in the resulting software product. At some point, it needs to be fixed.
A more common example along these lines might be omitting error handling. A developer just wants to get a function up and running and then add the logic but with no error handling.
def read_and_process_file(filename):
# Opening the file without any error handling
with open(filename, 'r') as file:
data = file.readlines()
# Processing the data assuming everything went well
total = 0
for line in data:
# Assuming each line in the file is a valid number
number = int(line.strip())
total += number
return f"Processing Complete. Total Sum: {total}"
# Example usage
result = read_and_process_file("data.txt")
print(result)
In this example, the open function can raise a FileNotFoundError if the specified file does not exist or an IOError if there's an issue with reading the file. The int function can raise a ValueError if a line is not a valid number. However, these exceptions are not caught, so the function will fail and terminate the program if such issues occur.
This is poor UX for an end user as the function will fail poorly. It is also bad for the development team as each of these error paths has to be added in at some point.
def read_and_process_file(filename):
try:
# Attempting to open and read the file
with open(filename, 'r') as file:
data = file.readlines()
except FileNotFoundError:
# Handling the case where the file does not exist
return f"Error: File '{filename}' not found."
except IOError:
# Handling other I/O errors
return f"Error: An error occurred while reading '{filename}'."
total = 0
for line in data:
try:
# Attempting to convert each line to an integer
number = int(line.strip())
total += number
except ValueError:
# Handling lines that are not valid numbers
return f"Error: Invalid number found in the file."
return f"Processing Complete. Total Sum: {total}"
# Example usage
result = read_and_process_file("data.txt")
print(result)
In this improved version, the function uses try-except blocks to handle both file-related errors (such as FileNotFoundError and IOError) and data processing errors (ValueError from invalid number conversion). This makes the function more robust and able to handle different types of errors gracefully, providing clear error messages instead of crashing.
2. Deliberate and Prudent
This is when developers consciously incur technical debt as a trade-off for delivering value faster or adapting to changing requirements or technologies.
For example, a team may use a third-party library or framework with known limitations to foster rapid development and implement a minimal viable product (MVP). This MVP is then used to test the market or get customer feedback.
This type of debt can be beneficial, as it can help developers learn, experiment, or validate their assumptions—as long as they pay off the debt as soon as possible.
3. Inadvertent and Reckless
In this scenario, developers unintentionally introduce technical debt due to a lack of knowledge, skills, or experience.
For example, developers may use outdated or incompatible technologies, frameworks, or libraries or apply inappropriate design patterns, architectures, or algorithms without realizing the implications or alternatives.
# Applying inappropriate design patterns without understanding the trade-offs
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def get_user_data(self):
# This method retrieves user data from a database
# ...
# This method also performs business logic and calculations
# ...
return user_data
In this example, the get_user_data method violates the single-responsibility principle (SRP), which states that a method should have only one reason to change. This method has two reasons to change:
- Changing how user data is retrieved from the database
- Changing the business logic on user data
This makes the method hard to maintain and understand and prone to bugs. A better design would be to split the data retrieval and business logic into two separate methods following the SRP.
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def retrieve_user_data(self):
# This method retrieves user data from a database
# ...
return user_data
def process_user_data(self, user_data):
# This method performs business logic and calculations on the user data
# ...
This type of debt is very harmful, as it can lead to poor performance, scalability, reliability, or security in the software.
4. Inadvertent and Prudent
Inadvertent and prudent technical debt happens when a knowledgeable team applies best practices during software development but inadvertently generates technical debt by failing to update/evolve the codebase. This technical debt is often due to the evolution or emergence of new requirements, technologies, or best practices.
For example, developers may use a design or library that was optimal at the time but becomes obsolete or suboptimal later due to new features, functionalities, or standards.
This type of debt is inevitable, as it is impossible to predict the future, but it can be manageable if teams refactor, update, or migrate their code regularly.
What Causes Technical Debt?
Technical debt is an inevitable consequence of being in business, as any software project will incur some degree of suboptimal design decisions, outdated code, or incomplete features. However, some of the most common causes of technical debt are:
Rapidly Changing Business Requirements
When developers must adapt to changing requirements rapidly, they might implement quick solutions or workarounds. This results in a codebase that accommodates immediate needs but lacks the elegance and scalability required for long-term sustainability.
For example, a simple modification, like transitioning a feature from single-user to multi-user functionality, can introduce unforeseen code complexities. If rushed, such changes can inadvertently cause technical debt to accumulate over time.
Additionally, these rapid changes often prevent the team from thoroughly considering the implications of their design choices, leading to a patchwork of solutions that can be difficult to refactor later.
Lack of Technical Knowledge
When team members are not well-versed in the latest technologies or coding standards, they may unknowingly introduce suboptimal solutions. This can lead to inefficient code structures and outdated libraries, making it harder for others to understand and maintain the code.
Furthermore, the lack of expertise can result in missing out on more efficient, scalable, or secure coding practices that are standard in the industry, thereby increasing the technical debt through a series of small but significant inefficiencies.
Lack of mentorship or proper documentation can prevent developers from learning best practices and understanding the system in a broader context. Without adequate knowledge sharing, new team members may struggle to understand the system's design or their role in maintaining it, increasing the risk of introducing errors or inconsistencies in the codebase.
Poor Development Processes and Inadequate Testing
Poorly conducted or skipped code reviews, relying solely on manual testing rather than using test automation, and lack of proper code documentation are all signs of poor development processes and can lead to technical debt.
These issues can be compounded over time as the absence of systematic reviews and testing makes the codebase progressively more fragile and error-prone. The lack of documentation also makes it challenging for new team members to understand the existing code, potentially leading to further suboptimal decisions and implementations.
Unrealistic Deadlines and Resource Constraints
External pressures, such as tight deadlines and limited resources, often force teams to prioritize speed over quality. Under these conditions, developers may sacrifice long-term maintainability and take shortcuts to deliver a product or feature quickly.
Over time, these shortcuts add up, and as more rushed fixes are added to the codebase, the overall code quality declines, creating a significant debt that will need to be paid off through refactoring or redesigning later.
Teams are sometimes understaffed or lack the resources to address technical debt adequately. Without a sufficient budget or personnel, teams may struggle to implement necessary refactoring, conduct thorough testing, or allocate enough time for designing scalable solutions. As a result, technical debt accumulates as quick fixes take priority over long-term improvements.
Poor Architecture and Design Decisions
A weak architectural foundation or poor design choices can lead to a codebase that is difficult to maintain and scale, creating technical debt that compounds over time.
Implementing simple features or maintaining the system can be more challenging when software is overengineered (i.e., designed with unnecessary complexity). On the other hand, underengineering, where the system is designed too simply, can lead to scaling problems when it grows.
Another issue is the lack of modularization. In tightly coupled systems, changes to one part of the codebase can have widespread impacts on other parts, making it difficult and costly to make changes (because any modification requires extensive testing and potentially reworking other parts of the system).
The codebase becomes difficult to extend when the initial code design does not account for future growth. Systems that work well at a small scale may struggle to handle increased load or complexity without significant refactoring.
Dependencies on Third-Party Tools and Services
Reliance on third-party tools and services can introduce technical debt, particularly when those dependencies become outdated or difficult to maintain. In such cases, developers may be forced to maintain them in-house or switch to an alternative.
Also, relying too heavily on a single vendor can create vendor lock-in, making it difficult to switch to a different solution if the vendor changes its offering, increases prices, or discontinues the service. This creates technical debt as teams must either adapt to new constraints or invest in migrating to a new solution.
Organizational and Cultural Factors
Organizational issues, such as poor leadership, communication breakdowns, and a lack of prioritization, can contribute to the accumulation of technical debt.
When leadership prioritizes new features over code quality or refactoring, technical debt is often ignored. This lack of focus on maintaining a clean, efficient codebase can lead to the accumulation of issues that become harder and more expensive to address later.
Misalignment between different teams—such as developers, product managers, and stakeholders—can lead to unclear or changing requirements, resulting in wasted effort and rework. These communication breakdowns often create technical debt, as developers may need to make compromises or rework portions of the system to meet unclear or evolving expectations.
It can help if you frame technical debt discussions around business metrics like customer churn and team productivity rather than code complexity scores.
“At FuseBase, I learned to frame technical debt discussions around business metrics like customer churn and team productivity rather than code complexity scores. We track how long it takes to onboard new developers or implement feature requests, using these metrics to justify debt cleanup sprints to our investors. Our team uses a mix of automated tools like CodeClimate and manual review processes, but I've found the most valuable insights come from regular developer surveys about which parts of our codebase slow them down the most.” – Paul Sher, CEO, FuseBase.
Is Technical Debt Bad?
While technical debt is typically viewed as a negative consequence of software development, it is not inherently bad. In fact, it can sometimes be a strategic decision that allows teams to prioritize speed or meet short-term business demands.
Technical debt arises when shortcuts are taken in development, and its impact can vary depending on how well it is managed. When tracked and appropriately addressed, technical debt can be a tool for balancing immediate needs with long-term goals.
Harmful Aspects of Technical Debt
Technical debt can be beneficial in the short run. However, it can snowball into major problems if not addressed on time. Here are some of its adverse effects.
Increased Maintenance Costs
As technical debt accumulates, maintaining and extending the codebase becomes more challenging. Teams need to invest more time and effort in debugging, refactoring, and patching issues that arise from outdated or inefficient code, detracting from time that could’ve been invested in shipping new features.
Higher Risk of Bugs and Security Vulnerabilities
Poorly maintained code is more prone to errors and security vulnerabilities. As technical debt builds up, it becomes harder to detect and fix bugs, which increases the likelihood of new issues arising. Additionally, systems that are not updated regularly may be exposed to known security risks, putting the entire application at risk of breaches or failures.
Slower Development Over Time
Accumulated technical debt can slow down development as the codebase becomes more complex. Due to the tangled nature of the existing code, new features become more challenging to implement and require more time to understand and modify. As a result, development cycles extend, making it harder to innovate or respond quickly to new business needs.
Developer Frustration and Turnover
Developers working with messy, poorly structured code often experience frustration. When the codebase becomes difficult to maintain or understand, morale decreases. If technical debt continues to increase, developers may become disillusioned with their work, which can result in higher turnover rates. Losing experienced talent is costly and can further exacerbate the technical debt problem, as new team members need time to ramp up.
Poor System Scalability and Performance Issues
As technical debt grows, it can create bottlenecks that hinder the system’s scalability and overall performance. Systems burdened with technical debt may struggle to handle increasing traffic or complexity, resulting in slower response times and reduced user satisfaction. Addressing these issues often requires significant refactoring, which can be costly and time-consuming.
Positive Aspects of Technical Debt
Technical debt is not all bad. Sometimes it doesn’t hurt to compromise here and there during development. Here are some of the good aspects of technical debt.
Faster Time-to-Markets
Taking on some technical debt can be a strategic decision in the early product launch stages. The priority at this stage is to validate ideas and capture market interest quickly.
Teams can take shortcuts to push products to market faster, gather user feedback, and make data-driven decisions. In such cases, the goal is to get something working fast and iterate later, rather than investing significant time in perfecting the code upfront.
Prioritizing Business Needs Over Perfection
In many cases, business pressures—such as tight deadlines, competitive forces, or market demands—require development teams to focus on delivering features or fixes rather than ensuring that every piece of code is perfectly clean.
Some level of technical debt might be necessary to meet these demands, as perfection can be the enemy of progress. However, it’s important to refactor the code in the near future so it doesn’t snowball in production.
Short-Term Experimentation
In certain scenarios, taking shortcuts is necessary for testing new concepts, features, or technologies. When teams want to experiment with a new idea or prototype, they may choose to accumulate some technical debt temporarily. This allows them to quickly test the waters and gauge whether the new concept is worth pursuing.
If the experiment proves successful, the code can be refactored and improved in future development cycles.
Debt That is Intentional and Well-Managed
Not all technical debt is problematic. When technical debt is intentionally planned, tracked, and managed, it can be addressed systematically over time.
Teams can prioritize technical debt repayment, balancing immediate needs with long-term sustainability. By making deliberate decisions about which areas of the code can accumulate debt and setting a clear strategy for resolving it, technical debt can be kept under control without negatively impacting the project’s overall success.
“Technical Debt always needs to be contextualized in the form of end user impact - how does spending time on this issue allow us to either go faster later, or bring immediate end user value.” – James Berthoty, CEO, Latio Tech.
Best Practices for Reducing Technical Debt
Technical debt can be used to enhance software delivery and improve the experience of those using your application. However, developers must know how to do this and all it entails. Here are some best practices to keep in mind.
Increase Awareness About Technical Debt
Software teams should recognize technical debt as an inherent part of software development. Technical debt is not a failure, but an unavoidable result of making trade-offs between delivery speed and code quality.
If teams are aware of this, they can make informed decisions about when it is acceptable to take on debt and when it’s necessary to repay it.
Encouraging open discussions about trade-offs allows developers to communicate the reasons behind technical decisions, whether they involve rapid delivery or long-term maintainability. This helps ensure that the team remains aligned on how technical debt impacts project outcomes.
It’s also important to increase awareness among non-technical stakeholders so they see technical debt on the same level as financial debt.
“At Rebrandly, we also had to "fight" to be heard about the need to address technical debt. Especially when things are working (or seem to be working), getting non-technical stakeholders to understand the importance of acting for long-term improvements becomes almost impossible.
That's precisely why, only months after drafting our technical debt lists-across FE, BE, and DevOps-we finally start seeing them as priorities that needed to be assigned sprint by sprint.
The key that unlocked this for us was presenting these lists with business-oriented metrics. We started tracking how often code had to be rewritten, the average bug resolution time, and the feature lead time (time from concept to deployment).
With this data in hand, we were finally able to give clear explanations for statements like: "Refactoring X will reduce feature development time by 30% over the next six months."
In short, we successfully put technical debt on the same level as financial debt, explaining how small, manageable debt are fine, but excessive debt leads to "interest" in the form of slower development and increased bugs.” - Eduard Andrei Capanu, Technical Leader (FE) - MVP Microsoft, Rebrandly
Implement Coding Standards and Best Practices
Enforcing clear and consistent coding standards is one of the most effective ways to prevent unnecessary technical debt. Coding guidelines ensure consistency in coding style, reduce the amount of errors, and put all the team members on the same page.
Adopting proven design patterns and principles is also vital. The SOLID principles, for example, help developers write code that is modular, reusable, and easy to refactor, making it easier to address any future changes or improvements.
Leverage Automation to Prevent Accumulation
Automated static and dynamic code analysis tools can help detect and flag potential technical debt early in development. These tools can identify code smells, inefficiencies, or violations of coding standards, allowing developers to address issues before they accumulate into larger problems.
Beyond SAST and DAST, teams can benefit from incorporating tools such as penetrative testing, CSPM, secrets detection, IaC, and SCA, all of which Codacy supports. These tools can be integrated into the CI/CD pipelines to automate the process and address technical debt before it compounds.
Document and Track Technical Debt
Maintaining a dedicated technical debt backlog separate from the product backlog helps teams prioritize technical debt alongside feature development. This allows for a clear view of what debt exists and what needs to be addressed over time.
Categorizing technical debt based on severity—such as critical versus low-priority debt—helps the team make informed decisions about what to tackle first. Critical debt should be addressed quickly, as it may impact the system's stability or security, while lower-priority debt can be tackled later during maintenance cycles.
Invest in Developer Training and Upskilling
Developer education is key to reducing the likelihood of introducing technical debt due to outdated practices. Providing ongoing training for developers in modern frameworks, tools, and best practices ensures that the team is up-to-date with industry standards.
Internal knowledge-sharing sessions, such as lunch-and-learns or workshops, help the team share insights and avoid common pitfalls. These sessions foster a culture of continuous learning and give developers the tools to improve their coding habits, reducing the chances of technical debt arising from miscommunication or lack of knowledge.
Encouraging mentorship programs within the team helps promote high-quality coding habits. Experienced developers can guide junior members, offering advice on writing maintainable code, avoiding shortcuts, and following best practices, all of which contribute to reducing technical debt.
Opt for Incremental Refactoring
Rather than attempting large-scale rewrites, which can be costly and risky, incremental refactoring allows teams to improve the codebase as part of regular feature development. Small, continuous improvements over time help keep the code clean and secure without complicating it or breaking any functionality.
Teams can reserve specific periods for refactoring and addressing known issues, preventing the backlog of technical debt from growing over time.
Improve Cross-Team Collaboration
Software is often a product of collaboration between development, product management, and QA teams. Good communication between these stakeholders plays a vital role in mitigating technical debt.
Organizations must invest in tools and services that facilitate collaboration. They must streamline working times, communication channels, project management tools, and real-time collaboration platforms to ensure teams can work efficiently, regardless of location.
“Board members? They want to know about money and reputation. IT team? They need the technical information. You need to know your audience.
In order to make things clear, I use everyday language and real-world examples. It helps people grasp what we're dealing with without getting lost in tech speak.
Prioritizing risks is crucial, so I often use visual tools like heat maps. It's a great way to show which issues we need to tackle first. And I don't just point out problems - I come with solutions. For each risk, I lay out a game plan: what we need to do, what resources we'll need, and what we can expect to achieve.
Keeping everyone in the loop is key. Regular updates, whether through reports or meetings, help everyone stay on the same page. And I make sure there's a clear structure for reporting and accountability. Everyone needs to know their role in keeping our digital assets safe.”- Eric Garcia, Founder & Cybersecurity Consultant, Cyber Wise Consulting.
Monitor and Measure Technical Debt
Defining key metrics to track technical debt helps teams objectively measure the impact of their codebase over time. Metrics such as cyclomatic complexity and the maintainability index provide a clear picture of how complex or challenging to maintain the code is, allowing the team to focus on areas that need improvement.
Another important metric is test coverage percentage. High test coverage indicates that the code is well-tested, which indicates a stable codebase and a lesser likelihood of technical debt. Monitor coverage over time to ensure that testing is comprehensive and effective.
Establish Clear Governance Policies for Managing Debt
Defining when and how technical debt should be addressed helps ensure that teams aren’t overwhelmed by debt accumulation. Clear guidelines make it easier for teams to prioritize debt based on its impact and urgency, ensuring that the most critical issues are resolved first.
Setting thresholds for acceptable debt levels provides a clear trigger for action. Teams should know when debt has reached a point where it needs to be addressed proactively, preventing it from spiraling out of control and causing long-term problems.
How to Tackle Technical Debt in the Scrum/Agile Process
Agile methodologies, like Scrum, are characterized by short, iterative cycles that aim to deliver functional software at the end of each sprint. The fast nature of Agile sprints makes them susceptible to tech debt that needs to be carefully managed, as accumulated technical debt can slow future development and impede the team's ability to deliver functional software. Here's how to tackle technical debt in the Scrum/Agile process.
Identify and Assess the Level of Technical Debt
The first step in tackling technical debt is to comprehensively understand the current state of the codebase and system infrastructure. This involves identifying systems and processes that are causing the most pain and assessing the severity of the issues.
Conducting code reviews is one way to identify potential technical debt issues. Encourage developers to provide feedback on each other's code, highlighting areas of complexity, duplication, and poor design. Additionally, use static code analysis tools to scan the codebase for potential technical debt patterns automatically. These tools can identify unused variables, complex conditional statements, and potential security vulnerabilities.
By understanding the level of technical debt within the codebase, engineering leaders can develop a plan to reduce it.
Develop a Roadmap for Solving Technical Debt
Once the technical debt has been identified and assessed, the next step is to create a roadmap for addressing it. This roadmap should outline the specific steps required to tackle the identified issues, prioritize the tasks based on their impact and urgency, and allocate the necessary resources.
For example, a roadmap for a team that wants to upgrade their React library can include the following components:
Vision:
To enhance the security of our application by upgrading the React version and using React best practices.
Goals:
- Upgrade the React version from 16.2 to 18.0, introducing new features and improvements, such as faster rendering, better error handling, and easier migration to future versions.
- Use React hooks, a new way of writing functional components using state and lifecycle methods without classes or higher-order components.
Actions:
- Create a backup of our current codebase and a new branch for the upgrade process.
- Install the latest version of React and update dependencies to be compatible with the new version.
- Refactor class components into functional components and replace the lifecycle methods with React hooks, such as useState, useEffect, or useRef.
- Merge the new branch into the main branch and deploy the application to the production environment.
Timelines:
- Complete the backup and installation of the new version and dependencies—one week.
- Complete the refactoring of the class components—seven weeks.
- Complete the testing and deployment—three weeks.
- Deliver the upgraded and secured application within—three weeks.
Once the goals, actions, and timelines have been defined, the team should embark on determining the necessary developers to address the technical debt.
The first priority should be to address tasks that have the highest potential impact on the application's security posture, such as addressing known security vulnerabilities and improving error handling.
Integrate Technical Debt Management into Sprints
The final step in tackling tech debt is integrating it into the development process. Allocate time for refactoring, code reviews, and code quality improvements during each sprint to ensure the team maintains a balance between delivering new features and maintaining code quality. By addressing technical debt incrementally within the sprint framework, the team can proactively manage and prevent it from causing larger impediments.
Tackling Technical Debt With the Right Tools
Tech debt is something that can cripple a company if it goes unchecked. Addressing technical debt involves periodic efforts to refactor and improve the existing code to ensure that it aligns with best practices and facilitates long-term security and development efficiency.
Codacy can help by automating and standardizing code reviews. Our best-in-class static code analysis solution integrates seamlessly into your workflows, helping engineering teams save time in code reviews and tackle technical debt. Try it for free today.
Technical Debt FAQs
1. What is technical debt, and why is it important?
Technical debt is the result of decisions that favor rapid delivery over long-term quality. It leads to increased maintenance work, security vulnerabilities, and delays in future development.
Lawrence Guyot, President, ETTE, explained, "Quantifying technical debt is challenging, but vital for aligning with non-technical stakeholders. At ETTE, we've used data analytics to translate technical debt into business impacts, showcasing potential costs of not addressing issues like outdated software that can lead to security vulnerabilities."
2. How can technical debt be measured?
Technical debt can be quantified by linking it to business metrics such as defect ratios, maintainability indices, and cost estimates related to system downtime.
Rodger Desai, CEO, Prove, shared, "A Technical Debt Scorecard translates complex code issues into clear, measurable scores, making it easier for non-technical leaders to understand the impact. Weighted criteria such as maintainability, performance impact, and security risks help teams assess which areas need immediate attention."
3. How should teams prioritize technical debt while continuing to develop new features?
Teams need to address technical debt without halting feature development by ranking issues based on risk and business impact.
Marin Cristian-Ovidiu, CEO, Online Games, explained, "Prioritization of technical debt repairs without burdening feature development demands intentional planning. Teams must rank debt as high-impact, moderate, and low issues, starting with those impacting security, scalability, or performance."
Lawrence Guyot of ETTE added, "Instead of just allocating time periodically, we assess the potential return on investment of addressing different debts, such as improved system performance or reduced maintenance costs, to decide what to tackle first."
4. How can technical debt be communicated to non-technical stakeholders?
Technical debt should be explained in terms that relate directly to business outcomes.
Paul Sher, CEO, FuseBase, explained, "At FuseBase, I learned to frame technical debt discussions around business metrics like customer churn and team productivity rather than code complexity scores. We track how long it takes to onboard new developers or implement feature requests, using these metrics to justify debt cleanup sprints to our investors."
Eduard Andrei Capanu, Technical Leader (FE) - MVP Microsoft, Rebrandly, observed, "We started tracking how often code had to be rewritten, the average bug resolution time, and the feature lead time. With this data in hand, we were finally able to give clear explanations for statements like: 'Refactoring X will reduce feature development time by 30% over the next six months.”
5. What role do automated tools play in managing technical debt?
Automated tools are integrated into development pipelines to identify, monitor, and manage technical debt continuously.
Marin Cristian-Ovidiu of Online Games noted that automated tools "play a pivotal role in the detection of code smells, complexity analysis, and notification of outdated dependencies."
Brian Pontarelli, CEO, FusionAuth, shared that these tools help "maintain code quality and reduce the burden of remediation down the line."